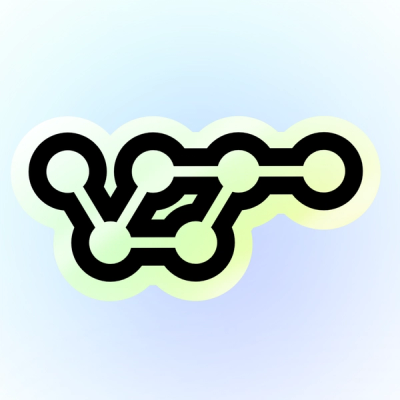
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
markdown-it
Advanced tools
The markdown-it npm package is a Markdown parser that can convert Markdown text into HTML. It is highly extensible and supports various plugins to enhance its functionality. It is commonly used to render user-generated content in web applications, documentation tools, and content management systems.
Basic Markdown Parsing
This feature allows you to convert basic Markdown text into HTML. The code sample demonstrates how to create a new instance of MarkdownIt and use it to render a simple Markdown string.
const MarkdownIt = require('markdown-it');
const md = new MarkdownIt();
const result = md.render('# Markdown-it rulezz!');
HTML Output Customization
Markdown-it allows customization of the HTML output through options. The code sample shows how to enable HTML tags in source, automatically convert URLs to links, and use typographic replacements.
const MarkdownIt = require('markdown-it');
const md = new MarkdownIt({
html: true,
linkify: true,
typographer: true
});
Syntax Extensions with Plugins
Markdown-it supports plugins to extend its syntax. The code sample demonstrates how to add emoji support to the Markdown parser using the markdown-it-emoji plugin.
const MarkdownIt = require('markdown-it');
const emojiPlugin = require('markdown-it-emoji');
const md = new MarkdownIt();
md.use(emojiPlugin);
const result = md.render('Hello :smile:');
Linkify
The linkify feature automatically detects URLs in the text and converts them into clickable links. The code sample shows how to enable this feature in the MarkdownIt instance.
const MarkdownIt = require('markdown-it');
const md = new MarkdownIt({
linkify: true
});
const result = md.render('Visit https://www.example.com');
Marked is a fast Markdown parser and compiler built for speed. It is less extensible than markdown-it but is known for its performance and being a low-level compiler.
Remarkable is another Markdown parser that claims to be very fast and efficient. It offers similar extensibility to markdown-it with a plugin system and is often used for its speed and comprehensive Markdown support.
Showdown is a JavaScript Markdown to HTML converter that can run both on the server and in the browser. It is often chosen for its client-side capabilities and extensibility with extensions.
Markdown parser done right. Fast and easy to extend.
Table of content
node.js & bower:
npm install markdown-it --save
bower install markdown-it --save
browser (CDN):
See also:
// node.js, "classic" way:
var MarkdownIt = require('markdown-it'),
md = new MarkdownIt();
var result = md.render('# markdown-it rulezz!');
// node.js, the same, but with sugar:
var md = require('markdown-it')();
var result = md.render('# markdown-it rulezz!');
// browser without AMD, added to "window" on script load
// Note, there are no dash.
var md = window.markdownit();
var result = md.render('# markdown-it rulezz!');
Single line rendering, without paragraph wrap:
var md = require('markdown-it')();
var result = md.renderInline('__markdown-it__ rulezz!');
(*) preset define combination of active rules and options. Can be
"full"
|"commonmark"
|"zero"
or "default"
if skipped.
// commonmark mode
var md = require('markdown-it')('commonmark');
// default mode
var md = require('markdown-it')();
// enable everything
var md = require('markdown-it')('full', {
html: true,
linkify: true,
typographer: true
});
// full options list (defaults)
var md = require('markdown-it')({
html: false, // Enable HTML tags in source
xhtmlOut: false, // Use '/' to close single tags (<br />).
// This is only for full CommonMark compatibility.
breaks: false, // Convert '\n' in paragraphs into <br>
langPrefix: 'language-', // CSS language prefix for fenced blocks. Can be
// useful for external highlighters.
linkify: false, // Autoconvert URL-like text to links
// Enable some language-neutral replacement + quotes beautification
typographer: false,
// Double + single quotes replacement pairs, when typographer enabled,
// and smartquotes on. Set doubles to '«»' for Russian, '„“' for German.
quotes: '“”‘’',
// Highlighter function. Should return escaped HTML,
// or '' if the source string is not changed and should be escaped externaly.
highlight: function (/*str, lang*/) { return ''; }
});
var md = require('markdown-it')()
.use(plugin1)
.use(plugin2, opts)
.use(plugin3);
Apply syntax highlighting to fenced code blocks with the highlight
option:
var hljs = require('highlight.js') // https://highlightjs.org/
// Actual default values
var md = require('markdown-it')({
highlight: function (str, lang) {
if (lang && hljs.getLanguage(lang)) {
try {
return hljs.highlight(lang, str).value;
} catch (__) {}
}
try {
return hljs.highlightAuto(str).value;
} catch (__) {}
return ''; // use external default escaping
}
});
If you are going to write plugins - take a look at Development info.
Enabled by default:
Disabled by default:
19^th^
H~2~0
++inserted text++
(experimental)==marked text==
(experimental)* Experimental extensions can be changed later for something like Critic Markup, but you will still be able to use old-style rules via external plugins if you prefer.
// Activate/deactivate rules
var md = require('markdown-it')()
.enable([ 'ins', 'mark' ])
.disable([ 'table' ]);
// Enable everything
md = require('markdown-it')('full', {
html: true,
linkify: true,
typographer: true,
});
// Manually enable rules, disabled by default:
var md = require('markdown-it')()
.enable([
/* core */
'abbr',
/* block */
'footnote',
'deflist',
/* inline */
'footnote_inline',
'ins',
'mark',
'sub',
'sup'
]);
Here is result of CommonMark spec parse at Core i5 2.4 GHz (i5-4258U):
$ benchmark/benchmark.js spec
Selected samples: (1 of 27)
> spec
Sample: spec.txt (110610 bytes)
> commonmark-reference x 40.42 ops/sec ±4.07% (51 runs sampled)
> current x 74.99 ops/sec ±4.69% (67 runs sampled)
> current-commonmark x 93.76 ops/sec ±1.23% (79 runs sampled)
> marked-0.3.2 x 22.92 ops/sec ±0.79% (41 runs sampled)
As you can see, markdown-it
doesn't pay with speed for it's flexibility.
Because it's written in monomorphyc style and uses JIT inline caches effectively.
markdown-it is the result of the decision of the authors who contributed to 99% of the Remarkable code to move to a project with the same authorship but new leadership (Vitaly and Alex). It's not a fork.
Big thanks to John MacFarlane for his work on the CommonMark spec and reference implementations. His work saved us a lot of time during this project's development.
Related Links:
[2.2.1] - 2014-12-29
FAQs
Markdown-it - modern pluggable markdown parser.
The npm package markdown-it receives a total of 4,991,458 weekly downloads. As such, markdown-it popularity was classified as popular.
We found that markdown-it demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.