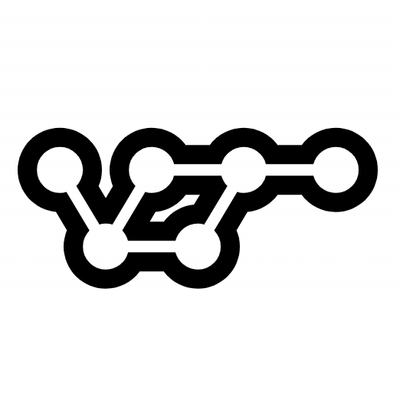
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
marked-highlight
Advanced tools
Highlight code blocks
npm install marked-highlight
You will need to provide a function that transforms the code
to html.
import { Marked } from "marked";
import { markedHighlight } from "marked-highlight";
import hljs from 'highlight.js';
// or UMD script
// <script src="https://cdn.jsdelivr.net/npm/marked/lib/marked.umd.js"></script>
// <script src="https://cdn.jsdelivr.net/npm/marked-highlight/lib/index.umd.js"></script>
// const {markedHighlight} = globalThis.markedHighlight;
const marked = new Marked(
markedHighlight({
langPrefix: 'hljs language-',
highlight(code, lang, info) {
const language = hljs.getLanguage(lang) ? lang : 'plaintext';
return hljs.highlight(code, { language }).value;
}
})
);
marked.parse(`
\`\`\`javascript
const highlight = "code";
\`\`\`
`);
// <pre><code class="hljs language-javascript">
// <span class="hljs-keyword">const</span> highlight = <span class="hljs-string">"code"</span>;
// </code></pre>
The async
option should be set to true
if the highlight
function returns a Promise
.
import { Marked } from "marked";
import { markedHighlight } from "marked-highlight";
import pygmentize from 'pygmentize-bundled';
const marked = new Marked(
markedHighlight({
async: true,
highlight(code, lang, info) {
return new Promise((resolve, reject) => {
pygmentize({ lang, format: 'html' }, code, function (err, result) {
if (err) {
reject(err);
return;
}
resolve(result.toString());
});
});
}
})
)
await marked.parse(`
\`\`\`javascript
const highlight = "code";
\`\`\`
`);
// <pre><code class="language-javascript">
// <div class="highlight">
// <pre>
// <span class="kr">const</span> <span class="nx">highlight</span> <span class="o">=</span> <span class="s2">"code"</span><span class="p">;</span>
// </pre>
// </div>
// </code></pre>
options
option | type | default | description |
---|---|---|---|
async | boolean | false | If the highlight function returns a promise set this to true . Don't forget to await the call to marked.parse |
langPrefix | string | 'language-' | A prefix to add to the class of the code tag. |
highlight | function | (code: string, lang: string) => {} | Required. The function to transform the code to html. |
FAQs
marked highlight
The npm package marked-highlight receives a total of 29,715 weekly downloads. As such, marked-highlight popularity was classified as popular.
We found that marked-highlight demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.