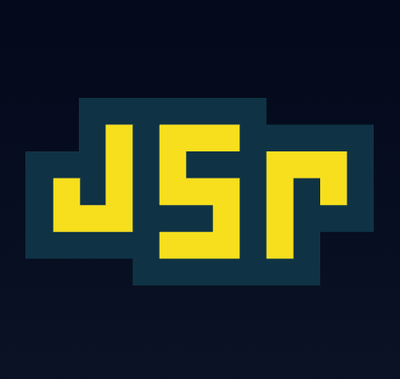
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The marked npm package is a markdown parser and compiler built for speed. It converts markdown syntax to HTML, and it is designed to be as extensible and fast as possible.
Markdown Parsing
This feature allows you to parse markdown text and convert it to HTML. The code sample shows how to use marked to convert a simple markdown string into HTML.
const marked = require('marked');
console.log(marked('# Marked in Node.js\n\nRendered by **marked**.'));
Options Customization
This feature allows you to customize the behavior of the marked parser by setting options such as GitHub Flavored Markdown (GFM), breaks, pedantic, sanitize, smart lists, and smartypants.
const marked = require('marked');
marked.setOptions({
renderer: new marked.Renderer(),
gfm: true,
breaks: false,
pedantic: false,
sanitize: false,
smartLists: true,
smartypants: false
});
console.log(marked('I am using __markdown__.'));
Synchronous Parsing
This feature allows you to parse markdown synchronously, which is useful when you don't need to handle asynchronous operations.
const marked = require('marked');
const html = marked('## Synchronous markdown to HTML');
console.log(html);
Asynchronous Parsing
This feature allows you to parse markdown asynchronously, which can be useful when dealing with file systems or network requests.
const marked = require('marked');
marked('# Asynchronous markdown to HTML', function(err, content) {
if (err) throw err;
console.log(content);
});
Lexer and Parser
This feature exposes the lexer and parser, allowing you to generate tokens from markdown and then parse those tokens into HTML. This can be useful for advanced use-cases where you need to manipulate the tokens before parsing.
const marked = require('marked');
const tokens = marked.lexer('# Lexing markdown');
console.log(tokens);
const html = marked.parser(tokens);
console.log(html);
Remarkable is an npm package that offers similar markdown parsing and rendering capabilities. It provides a full-featured markdown parser and compiler, and it emphasizes extensibility and performance, much like marked.
Showdown is another markdown to HTML converter that can be used both in the browser and on the server. It has a similar feature set to marked but also includes extensions which allow for additional syntax and features beyond the standard markdown.
Markdown-it is a modern markdown parser with a focus on speed and extensibility. It supports the CommonMark specification and has a similar feature set to marked, but it also includes a plugin system for extending its capabilities.
A full-featured markdown parser and compiler. Built for speed.
node v0.4.x
$ node test --bench
marked completed in 12071ms.
showdown (reuse converter) completed in 27387ms.
showdown (new converter) completed in 75617ms.
markdown-js completed in 70069ms.
node v0.6.x
$ node test --bench
marked completed in 6448ms.
marked (gfm) completed in 7357ms.
marked (pedantic) completed in 6092ms.
discount completed in 7314ms.
showdown (reuse converter) completed in 16018ms.
showdown (new converter) completed in 18234ms.
markdown-js completed in 24270ms.
Marked is now faster than Discount, which is written in C.
For those feeling skeptical: These benchmarks run the entire markdown test suite 1000 times. The test suite tests every feature. It doesn't cater to specific aspects.
Benchmarks for other engines to come (?).
$ npm install marked
The point of marked was to create a markdown compiler where it was possible to frequently parse huge chunks of markdown without having to worry about caching the compiled output somehow...or blocking for an unnecesarily long time.
marked is very concise and still implements all markdown features. It is also now fully compatible with the client-side.
marked more or less passes the official markdown test suite in its entirety. This is important because a surprising number of markdown compilers cannot pass more than a few tests. It was very difficult to get marked as compliant as it is. It could have cut corners in several areas for the sake of performance, but did not in order to be exactly what you expect in terms of a markdown rendering. In fact, this is why marked could be considered at a disadvantage in the benchmarks above.
Along with implementing every markdown feature, marked also implements GFM features.
marked has 3 different switches which change behavior.
markdown.pl
as much as possible.
Don't fix any of the original markdown bugs or poor behavior.None of the above are mutually exclusive/inclusive.
// set default options
marked.setOptions({
gfm: true,
pedantic: false,
sanitize: true
});
console.log(marked('i am using __markdown__.'));
You also have direct access to the lexer and parser if you so desire.
var tokens = marked.lexer(str);
console.log(marked.parser(tokens));
$ node
> require('marked').lexer('> i am using marked.')
[ { type: 'blockquote_start' },
{ type: 'text', text: ' i am using marked.' },
{ type: 'blockquote_end' },
links: {} ]
$ marked -o hello.html
hello world
^D
$ cat hello.html
<p>hello world</p>
Marked has an interface that allows for a syntax highlighter to highlight code blocks before they're output.
Example implementation:
var highlight = require('my-syntax-highlighter')
, marked_ = require('marked');
var marked = function(text) {
var tokens = marked_.lexer(text)
, l = tokens.length
, i = 0
, token;
for (; i < l; i++) {
token = tokens[i];
if (token.type === 'code') {
token.text = highlight(token.text, token.lang);
// marked should not escape this
token.escaped = true;
}
}
text = marked_.parser(tokens);
return text;
};
module.exports = marked;
Copyright (c) 2011-2012, Christopher Jeffrey. (MIT License)
See LICENSE for more info.
FAQs
A markdown parser built for speed
The npm package marked receives a total of 8,779,179 weekly downloads. As such, marked popularity was classified as popular.
We found that marked demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.