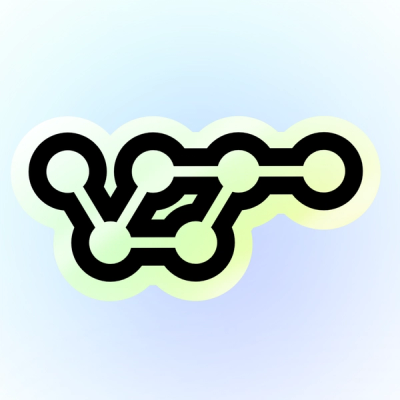
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
DEPRECATION WARNING: Matador has been deprecated and is no longer actively maintained. We do not recommend continuing to use Matador.
Sane defaults and a simple structure, scaling as your application grows.
Matador is a clean, organized framework for Node.js architected to suit MVC enthusiasts. It gives you a well-defined development environment with flexible routing, easy controller mappings, and basic request filtering. It’s built on open source libraries such as SoyNode for view rendering, and connect.js to give a bundle of other Node server related helpers.
$ npm install matador -g
$ matador init my-app
$ cd my-app && npm install matador
$ node server.js
// app/config/routes.js
'/hello/:name': { 'get': 'Home.hello' }
// app/controllers/HomeController.js
hello: function (request, response, name) {
response.send('hello ' + name)
}
Uses SoyNode to render Closure Templates.
// app/controllers/HomeController.js
this.render(response, 'index', {
title: 'Hello Bull Fighters'
})
<!-- app/views/layout.soy -->
{namespace views.layout}
/**
* Renders the index page.
* @param title Title of the page.
*/
{template .layout autoescape="contextual"}
<!DOCTYPE html>
<html>
<head>
<meta http-equiv='Content-type' content='text/html; charset=utf-8'>
<title>{$title}</title>
<link rel='stylesheet' href='/css/main.css' type='text/css'>
</head>
<body>
{$ij.bodyHtml |noAutoescape}
</body>
</html>
{/template}
{namespace views.index}
/**
* Renders a welcome message.
* @param title Title of the page
*/
{template .welcome}
<h1>Welcome to {$title}</h1>
(rendered with Closure Templates)
{/template}
The app/config/routes.js
file is where you specify an array of tuples indicating where incoming requests will map to a controller
and the appropriate method. If no action is specified, it defaults to 'index' as illustrated below:
module.exports = function (app) {
return {
'/': 'Home' // maps to ./HomeController.js => index
, '/admin': 'Admin.show' // maps to ./admin/AdminController.js => show
}
}
You can also specify method names to routes:
module.exports = function (app) {
return {
'/posts': {
'get': 'Posts.index', // maps to PostsController.js => #index
'post': 'Posts.create' // maps to PostsController.js => #create
}
}
}
By default, Models are thin with just a Base and Application Model in place. You can give them some meat, for example, and embed Mongo Schemas. See the following as a brief illustration:
// app/models/ApplicationModel.js
module.exports = function (app, config) {
var BaseModel = app.getModel('Base', true)
function ApplicationModel() {
BaseModel.call(this)
this.mongo = require('mongodb')
this.mongoose = require('mongoose')
this.Schema = this.mongoose.Schema
this.mongoose.connect('mongodb://localhost/myapp')
}
util.inherits(ApplicationModel, BaseModel)
return ApplicationModel
}
Then create, for example, a UserModel.js that extended it...
module.exports = function (app, config) {
var ApplicationModel = app.getModel('Application', true)
function UserModel() {
ApplicationModel.call(this)
this.DBModel = this.mongoose.model('User', new this.Schema({
name: { type: String, required: true, trim: true }
, email: { type: String, required: true, lowercase: true, trim: true }
}))
}
util.inherits(UserModel, ApplicationModel)
return DBModel
UserModel.prototype.create = function (name, email, callback) {
var user = new this.DBModel({
name: name
, email: email
})
user.save(callback)
}
UserModel.prototype.find = function (id, callback) {
this.DBModel.findById(id, callback)
}
}
This provides a proper abstraction between controller logic and how your models interact with a database then return data back to controllers.
Take special note that models do not have access to requests or responses, as they rightfully shouldn't.
Matador uses the default node inheritance patterns via util.inherits
.
$ matador controller [name]
$ matador model [name]
Questions, pull requests, bug reports are all welcome. Submit them here on Github. When submitting pull requests, please run through the linter to conform to the framework style
$ npm install -d
$ npm run-script lint
Obviously, Dustin Senos & Dustin Diaz
Copyright 2012 Obvious Corporation
Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
FAQs
an MVC framework for Node
The npm package matador receives a total of 6 weekly downloads. As such, matador popularity was classified as not popular.
We found that matador demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.