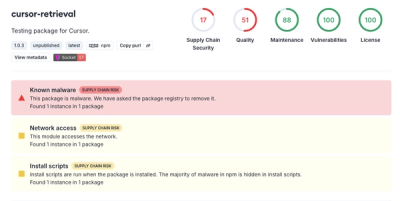
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
A Javascript Library to perform basic matrix operations using the functional nature of Javascript
A Javascript Library to perform basic matrix operations using the functional nature of Javascript
var a = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
var A = matrix(a);
A(); //returns [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
A(0); // returns [1, 2, 3]
A([], 0); // returns [[1], [4], [7]]
A(1, 2); // returns 6
A([1,2]); // returns [[4, 5, 6], [7, 8, 9]]
A([],[1,2]); // returns [[2, 3], [5, 6], [8, 9]]
A([1,2],[1,2]); // returns [[5, 6], [8, 9]]
A([2,1],[]); // returns [[7, 8, 9], [4, 5 ,6]]
A([],[2,1]); // returns [[3, 2], [6, 5], [9, 8]]
A([2,1],[2,1]); // returns [[9, 8], [6, 5]]
A.size(); //returns [3, 3]
A.set(0).to(0); // returns [[0, 0, 0], [4, 5, 6], [7, 8, 9]]
A.set(1,2).to(10); // returns [[1, 2, 3], [4, 5, 10], [7, 8, 9]]
A.set([], 0).to(0); // returns [[0, 2, 3], [0, 5, 6], [0, 8, 9]]
A.set([1,2]).to(4); // returns [[1, 2, 3], [4, 4, 4], [4, 4, 4]]
A.set([], [1,2]).to(1); // returns [[1, 1, 1], [4, 1, 1], [7, 1, 1]]
var B = matrix([[3, 4, 5], [6, 7, 8], [9, 10, 11]]);
A.add(B); // returns [[4, 6, 8], [10, 12, 14], [16, 18, 20]]
B.sub(A); // returns [[2, 2, 2], [2, 2, 2], [2, 2, 2]]
A.mul(B); // returns [[3, 8, 15], [24, 35, 48], [56, 80, 99]]
NOTE: This is not classical matrix multiplication (which is implemented using the prod() method). This simply multiplies together each element in matrix A with the corresponding element in matrix B. If A and B are not the same size, it will produce some NaN results.
A.div(B); // returns [[0.33, 0.5, 0.6], [0.66, 0.71, 0.75], [0.77, 0.8, 0.81]]
A.prod(B); // returns [[42, 48, 54], [96, 111, 126], [150, 174, 198]]
A.trans(); // returns [[1, 4, 7], [2, 5, 8], [3, 6, 9]]
var C = matrix([[5, 4, 7], [4, 8, 2], [9, 0, 4]]);
C.det(); // returns -336
Should be invertible
M = matrix([[1, 3, 3], [1, 4, 3], [1, 3 ,4]]);
M.inv(); // returns [[7, -3, -3], [-1, 1, 0], [-1, 0 ,1]]
Merges two matrices in all directions
M = matrix([[3, 4], [7, 8]]);
M.merge.left([[1, 2], [5, 6]]); // returns [[1, 2, 3, 4], [5, 6, 7, 8]]
M = matrix([[1, 2], [5, 6]]);
M.merge.right([[3, 4], [7, 8]]); // returns [[1, 2, 3, 4], [5, 6, 7, 8]]
M = matrix([5, 6, 7, 8]);
M.merge.top([1, 2, 3, 4]); // returns [[1, 2, 3, 4], [5, 6, 7, 8]]
M = matrix([1, 2, 3 ,4]);
M.merge.bottom([5, 6, 7, 8]); // returns [[1, 2, 3, 4], [5, 6, 7, 8]]
Applies a given function over the matrix, elementwise. Similar to Array.map()
M = matrix([1, 2, 3]);
M.map(x => x*x); // returns [1, 4, 9]
This example shows the arguments provided to the function
M = matrix([[1, 2], [3, 4]]);
M.map((value, pos, mat) => value * pos[1]);
// returns [[0, 2], [0, 4]]
Checks the equality of two matrices and returns a boolean. A matrix is equal to itself.
A = matrix([[1,2],[3,4]]);
A.equals(A);
// returns true
B = matrix([[3,4], [1,2]]);
A.equals(B);
// returns false
Generates a matrix with the value passed across the entire matrix or just the diagonal.
matrix.gen(4).size(2,3); // returns [[4,4,4],[4,4,4]]
matrix.gen(2).size(2); // returns [[2,2],[2,2]]
matrix.gen(1).diag(3); // return [[1,0,0],[0,1,0],[0,0,1]], identity matrix
// Diagonal matrices are normally square. Here, only diagonal elements are filled where row and column indices are same.
matrix.gen(1).diag(3,2); // returns [[1,0],[0,1],[0,0]]
matrix.gen(2).diag(3,4); // returns [[2,0,0,0],[0,2,0,0],[0,0,2,0]
FAQs
A Javascript Library to perform basic matrix operations using the functional nature of Javascript
The npm package matrix-js receives a total of 309 weekly downloads. As such, matrix-js popularity was classified as not popular.
We found that matrix-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.