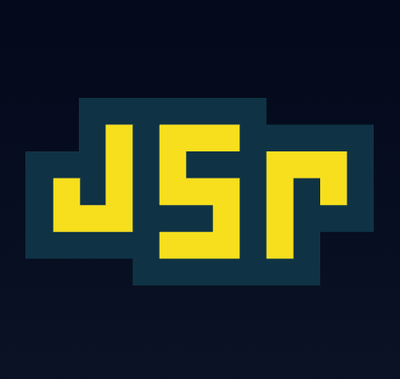
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
mdast-util-gfm-strikethrough
Advanced tools
The `mdast-util-gfm-strikethrough` package is a utility for working with strikethrough text in Markdown Abstract Syntax Trees (MDAST). It provides functionality to parse and serialize strikethrough text according to the GitHub Flavored Markdown (GFM) specification.
Parse Strikethrough
This feature allows you to parse Markdown text containing strikethrough syntax into an MDAST. The code sample demonstrates how to convert a Markdown string with strikethrough text into an MDAST tree.
const { fromMarkdown } = require('mdast-util-from-markdown');
const { gfmStrikethrough } = require('mdast-util-gfm-strikethrough');
const markdown = 'This is ~~strikethrough~~ text.';
const tree = fromMarkdown(markdown, { extensions: [gfmStrikethrough()] });
console.log(JSON.stringify(tree, null, 2));
Serialize Strikethrough
This feature allows you to serialize an MDAST containing strikethrough nodes back into Markdown text. The code sample demonstrates how to convert an MDAST tree with strikethrough nodes into a Markdown string.
const { toMarkdown } = require('mdast-util-to-markdown');
const { gfmStrikethrough } = require('mdast-util-gfm-strikethrough');
const tree = {
type: 'root',
children: [
{
type: 'paragraph',
children: [
{ type: 'text', value: 'This is ' },
{ type: 'delete', children: [{ type: 'text', value: 'strikethrough' }] },
{ type: 'text', value: ' text.' }
]
}
]
};
const markdown = toMarkdown(tree, { extensions: [gfmStrikethrough()] });
console.log(markdown);
The `remark-gfm` package is a plugin for the `remark` ecosystem that adds support for GitHub Flavored Markdown (GFM) syntax, including strikethrough. It provides a more comprehensive set of GFM features compared to `mdast-util-gfm-strikethrough`, which focuses specifically on strikethrough.
The `markdown-it` package is a Markdown parser that supports a wide range of Markdown features, including GFM strikethrough. It is a more general-purpose parser compared to `mdast-util-gfm-strikethrough`, which is specifically designed for working with MDAST.
The `turndown` package is a HTML to Markdown converter that supports various Markdown features, including GFM strikethrough. It is useful for converting HTML content to Markdown, whereas `mdast-util-gfm-strikethrough` is focused on parsing and serializing strikethrough in MDAST.
mdast extensions to parse and serialize GFM strikethrough.
This package contains extensions that add support for the strikethrough syntax
enabled by GFM to mdast-util-from-markdown
and
mdast-util-to-markdown
.
These tools are all rather low-level.
In most cases, you’d want to use remark-gfm
with remark instead.
When you are working with syntax trees and want all of GFM, use
mdast-util-gfm
instead.
When working with mdast-util-from-markdown
, you must combine this package with
micromark-extension-gfm-strikethrough
.
This utility does not handle how markdown is turned to HTML.
That’s done by mdast-util-to-hast
.
If you want a different element, you should configure that utility.
This package is ESM only. In Node.js (version 12.20+, 14.14+, or 16.0+), install with npm:
npm install mdast-util-gfm-strikethrough
In Deno with esm.sh
:
import {gfmStrikethroughFromMarkdown, gfmStrikethroughToMarkdown} from 'https://esm.sh/mdast-util-gfm-strikethrough@1'
In browsers with esm.sh
:
<script type="module">
import {gfmStrikethroughFromMarkdown, gfmStrikethroughToMarkdown} from 'https://esm.sh/mdast-util-gfm-strikethrough@1?bundle'
</script>
Say our document example.md
contains:
*Emphasis*, **importance**, and ~~strikethrough~~.
…and our module example.js
looks as follows:
import fs from 'node:fs/promises'
import {fromMarkdown} from 'mdast-util-from-markdown'
import {toMarkdown} from 'mdast-util-to-markdown'
import {gfmStrikethrough} from 'micromark-extension-gfm-strikethrough'
import {gfmStrikethroughFromMarkdown, gfmStrikethroughToMarkdown} from 'mdast-util-gfm-strikethrough'
const doc = await fs.readFile('example.md')
const tree = fromMarkdown(doc, {
extensions: [gfmStrikethrough()],
mdastExtensions: [gfmStrikethroughFromMarkdown]
})
console.log(tree)
const out = toMarkdown(tree, {extensions: [gfmStrikethroughToMarkdown]})
console.log(out)
Now, running node example
yields:
{
type: 'root',
children: [
{
type: 'paragraph',
children: [
{type: 'emphasis', children: [{type: 'text', value: 'Emphasis'}]},
{type: 'text', value: ', '},
{type: 'strong', children: [{type: 'text', value: 'importance'}]},
{type: 'text', value: ', and '},
{type: 'delete', children: [{type: 'text', value: 'strikethrough'}]},
{type: 'text', value: '.'}
]
}
]
}
*Emphasis*, **importance**, and ~~strikethrough~~.
This package exports the identifiers gfmStrikethroughFromMarkdown
and
gfmStrikethroughToMarkdown
.
There is no default export.
gfmStrikethroughFromMarkdown
Extension for mdast-util-from-markdown
.
gfmStrikethroughToMarkdown
Extension for mdast-util-to-markdown
.
The following interfaces are added to mdast by this utility.
Delete
interface Delete <: Parent {
type: "delete"
children: [TransparentContent]
}
Delete (Parent) represents contents that are no longer accurate or no longer relevant.
Delete can be used where static phrasing content is expected. Its content model is transparent content.
For example, the following markdown:
~~alpha~~
Yields:
{
type: 'delete',
children: [{type: 'text', value: 'alpha'}]
}
StaticPhrasingContent
(GFM strikethrough)type StaticPhrasingContentGfm = Delete | StaticPhrasingContent
This package is fully typed with TypeScript. It does not export additional types.
The Delete
node type is supported in @types/mdast
by default.
Projects maintained by the unified collective are compatible with all maintained versions of Node.js. As of now, that is Node.js 12.20+, 14.14+, and 16.0+. Our projects sometimes work with older versions, but this is not guaranteed.
This plugin works with mdast-util-from-markdown
version 1+ and
mdast-util-to-markdown
version 1+.
remarkjs/remark-gfm
— remark plugin to support GFMsyntax-tree/mdast-util-gfm
— same but all of GFM (autolink literals, footnotes, strikethrough, tables,
tasklists)micromark/micromark-extension-gfm-strikethrough
— micromark extension to parse GFM strikethroughSee contributing.md
in syntax-tree/.github
for
ways to get started.
See support.md
for ways to get help.
This project has a code of conduct. By interacting with this repository, organization, or community you agree to abide by its terms.
FAQs
mdast extension to parse and serialize GFM strikethrough
We found that mdast-util-gfm-strikethrough demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.