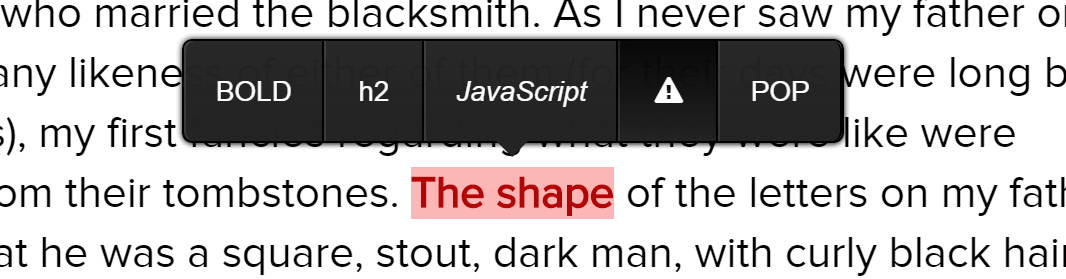
MediumButton
MediumButton extends MediumEditor with your custom buttons.
You can still use the default ones, MediumButton just gives you the ability to add custom buttons.
I need your support to further develop this package. :)
Demo
Installation
Next copy and reference the scripts (located in the dist folder)
<script src="js/medium-editor.min.js"></script>
<script src="js/medium-button.min.js"></script>
Usage
Follow the steps on the MediumEditor Page
Then you can then setup your custom buttons
HTML buttons
'b': new MediumButton({label:'<b>B</b>', start:'<b>', end:'</b>'})
label: '<b>B</b>',
start: '<b>',
end: '</b>'
JavaScript buttons
'pop': new MediumButton({label:'<b>Hello</b>', action: function(html, mark){alert('hello');return html}})
label: '<b>Hello</b>',
action: function(html, mark, parent){
alert('hello')
console.log(parent)
return html
}
(you can combine the two)
Add them to MediumEditor
toolbar: {
buttons: ['b', 'h2', 'JS', 'warning', 'pop']
},
extensions: {
'b': new MediumButton({label:'BOLD', start:'<b>', end:'</b>'}),
}
and you're done.
Example
var editor = new MediumEditor('.editor', {
toolbar: {
buttons: ['b', 'h2', 'warning', 'pop']
},
extensions: {
'b': new MediumButton({label:'BOLD', start:'<b>', end:'</b>'}),
'h2': new MediumButton({label:'h2', start:'<h2>', end:'</h2>'}),
'warning': new MediumButton({
label: '<i class="fa fa-exclamation-triangle"></i>',
start: '<div class="warning">',
end: '</div>'
}),
'pop': new MediumButton({
label:'POP',
action: function(html, mark, parent){
alert('hello :)')
return html
}
})
}
})
Syntax highlighting
Syntax highlighting is possible but not that easy(for now). You need to add an other Script like Prism or highlight.js. Here is an example for JavaScript with highlight.js.
'JS': new MediumButton({
label: '<i>JavaScript</i>',
start: '<pre><code>',
end: '</code></pre>',
action: function(html, mark, parent){
if(mark) return '<!--'+html+'-->' + hljs.highlight('javascript', html.substring(3, html.length - 4).replace(/<\/p><p>/g, "\n").replace(/</g, "<").replace(/>/g, ">")).value;
return html.split('-->')[0].split('<!--').join('');
}
})