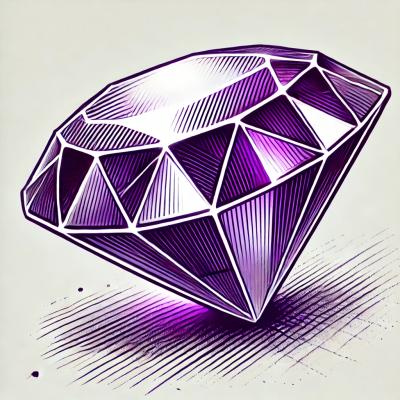
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
meerkat-api
Advanced tools
This is a Node.js implementation of a wrapper for communicating with the Meerkat API.
npm install --save meerkat-api
var Meerkat = require('meerkat-api');
// Create an instance.
var meerkat = new Meerkat(API_TOKEN);
// You can get an API token here: http://developers.meerkatapp.co/api/
/**
* General requests.
*/
// Callback style:
meerkat.getAllBroadcasts(function (err, broadcasts) {
console.log(err || broadcasts);
});
// Promise style:
meerkat.getAllBroadcasts().
then(function (broadcasts) {
console.log(broadcasts);
}, function (err) {
console.log(err);
});
// Callback style:
meerkat.getScheduledBroadcasts(function (err, broadcasts) {
console.log(err || broadcasts);
});
// Promise style:
meerkat.getScheduledBroadcasts().
then(function (broadcasts) {
console.log(broadcasts);
}, function (err) {
console.log(err);
});
/**
* Broadcast-specific requests.
*/
var broadcastId = '6a4da7ba-20f5-4f0d-81de-e5cf37b3072b';
meerkat.getBroadcastSummary(broadcastId, function (err, summary) {
console.log(err || summary);
});
meerkat.getBroadcastSummary(broadcastId).
then(function (summary) {
console.log(summary);
}, function (err) {
console.log(err);
});
meerkat.getBroadcastActivities(broadcastId, function (err, activities) {
console.log(err || activities);
});
meerkat.getBroadcastActivities(broadcastId).
then(function (activities) {
console.log(activities);
}, function (err) {
console.log(err);
});
meerkat.getBroadcastRestreams(broadcastId, function (err, restreams) {
console.log(err || restreams);
});
meerkat.getBroadcastRestreams(broadcastId).
then(function (restreams) {
console.log(restreams);
}, function (err) {
console.log(err);
});
meerkat.getBroadcastComments(broadcastId, function (err, comments) {
console.log(err || comments);
});
meerkat.getBroadcastComments(broadcastId).
then(function (comments) {
console.log(comments);
}, function (err) {
console.log(err);
});
meerkat.getBroadcastLikes(broadcastId, function (err, likes) {
console.log(err || likes);
});
meerkat.getBroadcastLikes(broadcastId).
then(function (likes) {
console.log(likes);
}, function (err) {
console.log(err);
});
meerkat.getBroadcastWatchers(broadcastId, function (err, watchers) {
console.log(err || watchers);
});
meerkat.getBroadcastWatchers(broadcastId).
then(function (watchers) {
console.log(watchers);
}, function (err) {
console.log(err);
});
/**
* User-specific requests.
*/
var userId = '54fb2e454d0000d23bb9c40f';
meerkat.getUserDetails(userId, function (err, userDetails) {
console.log(err || userDetails);
});
meerkat.getUserDetails(userId).
then(function (userDetails) {
console.log(userDetails);
}, function (err) {
console.log(err);
});
/**
* Don't forget to clean up when exiting.
*/
process.on('SIGINT', shutdown);
process.on('SIGTERM', shutdown);
function shutdown () {
// Don't accept any more requests. Finish handling the current requests.
server.close(function () {
meerkat.dispose();
});
}
FAQs
Node.js wrapper for Meerkat API
The npm package meerkat-api receives a total of 0 weekly downloads. As such, meerkat-api popularity was classified as not popular.
We found that meerkat-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.