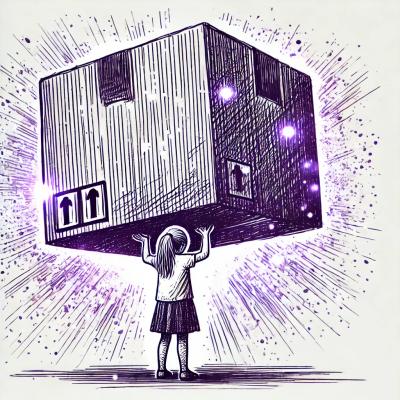
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
meilisearch
Advanced tools
β‘ The MeiliSearch API client written for JavaScript
MeiliSearch JavaScript is the MeiliSearch API client for JavaScript developers. MeiliSearch is a powerful, fast, open-source, easy to use and deploy search engine. Both searching and indexing are highly customizable. Features such as typo-tolerance, filters, and synonyms are provided out-of-the-box.
See our Documentation or our API References.
With npm
:
npm install meilisearch
With yarn
:
yarn add meilisearch
There are many easy ways to download and run a MeiliSearch instance.
For example, if you use Docker:
$ docker pull getmeili/meilisearch:latest # Fetch the latest version of MeiliSearch image from Docker Hub
$ docker run -it --rm -p 7700:7700 getmeili/meilisearch:latest ./meilisearch --master-key=masterKey
NB: you can also download MeiliSearch from Homebrew or APT.
import MeiliSearch from 'meilisearch'
const client = new MeiliSearch({
host: 'http://127.0.0.1:7700',
apiKey: 'masterKey',
})
<script src="https://cdn.jsdelivr.net/npm/meilisearch@latest/dist/bundles/meilisearch.umd.js"></script>
<script>
const client = new MeiliSearch({
host: 'http://127.0.0.1:7700',
apiKey: 'masterKey',
})
client.listIndexes().then(res => {
console.log({ res });
})
</script>
const MeiliSearch = require('meilisearch')
const client = new MeiliSearch({
host: 'http://127.0.0.1:7700',
apiKey: 'masterKey',
})
const MeiliSearch = require('meilisearch')
// Or if you are on a front-end environment:
import MeiliSearch from 'meilisearch'
;(async () => {
const client = new MeiliSearch({
host: 'http://127.0.0.1:7700',
apiKey: 'masterKey',
})
const index = await client.createIndex('books') // If your index does not exist
// OR
const index = client.getIndex('books') // If your index exists
const documents = [
{ book_id: 123, title: 'Pride and Prejudice' },
{ book_id: 456, title: 'Le Petit Prince' },
{ book_id: 1, title: 'Alice In Wonderland' },
{ book_id: 1344, title: 'The Hobbit' },
{ book_id: 4, title: 'Harry Potter and the Half-Blood Prince', genre: 'fantasy' },
{ book_id: 42, title: "The Hitchhiker's Guide to the Galaxy", genre: 'fantasy' }
]
let response = await index.addDocuments(documents)
console.log(response) // => { "updateId": 0 }
})()
With the updateId
, you can check the status (enqueued
, processed
or failed
) of your documents addition using the update endpoint.
// MeiliSearch is typo-tolerant:
const search = await index.search('harry pottre')
console.log(search)
Output:
{
"hits": [
{
"book_id": 4,
"title": "Harry Potter and the Half-Blood Prince"
}
],
"offset": 0,
"limit": 20,
"nbHits": 1,
"exhaustiveNbHits": false,
"processingTimeMs": 1,
"query": "harry pottre"
}
All the supported options are described in the search parameters section of the documentation.
await index.search(
'prince',
{
attributesToHighlight: ['*'],
filters: 'book_id > 10'
}
)
{
"hits": [
{
"book_id": 456,
"title": "Le Petit Prince",
"_formatted": {
"book_id": 456,
"title": "Le Petit <em>Prince</em>"
}
}
],
"offset": 0,
"limit": 20,
"nbHits": 1,
"exhaustiveNbHits": false,
"processingTimeMs": 0,
"query": "prince"
}
Placeholder search makes it possible to receive hits based on your parameters without having any query (q
).
await index.search(
'',
{
facetFilters: ['genre:fantasy'],
facetsDistribution: ['genre']
}
)
{
"hits": [
{
"id": 4,
"title": "Harry Potter and the Half-Blood Prince",
"genre": "fantasy"
},
{
"id": 42,
"title": "The Hitchhiker's Guide to the Galaxy",
"genre": "fantasy"
}
],
"offset": 0,
"limit": 20,
"nbHits": 2,
"exhaustiveNbHits": false,
"processingTimeMs": 0,
"query": "",
"facetsDistribution": { "genre": { "fantasy": 2 } },
"exhaustiveFacetsCount": true
}
You can abort a pending search request by providing an AbortSignal to the request.
const controller = new AbortController()
index
.search('prince', {}, 'POST', {
signal: controller.signal,
})
.then((response) => {
/** ... */
})
.catch((e) => {
/** Catch AbortError here. */
})
controller.abort()
This package only guarantees the compatibility with the version v0.16.0 of MeiliSearch.
The following sections may interest you:
This repository also contains more examples.
Any new contribution is more than welcome in this project!
If you want to know more about the development workflow or want to contribute, please visit our contributing guidelines for detailed instructions!
client.getIndex<T>('xxx').search(query: string, options: SearchParams = {}, method: 'POST' | 'GET' = 'POST', config?: Partial<Request>): Promise<SearchResponse<T>>
client.listIndexes(): Promise<IndexResponse[]>
client.createIndex<T>(uid: string, options?: IndexOptions): Promise<Index<T>>
client.getIndex<T>(uid: string): Index<T>
client.getOrCreateIndex<T>(uid: string, options?: IndexOptions): Promise<Index<T>>
index.show(): Promise<IndexResponse>
index.updateIndex(data: IndexOptions): Promise<IndexResponse>
index.deleteIndex(): Promise<void>
index.getStats(): Promise<IndexStats>
index.getUpdateStatus(updateId: number): Promise<Update>
index.getAllUpdateStatus(): Promise<Update[]>
index.waitForPendingUpdate(updateId: number, { timeOutMs?: number, intervalMs?: number }): Promise<Update>
index.addDocuments(documents: Document<T>[]): Promise<EnqueuedUpdate>
index.updateDocuments(documents: Document<T>[]): Promise<EnqueuedUpdate>
index.getDocuments(params: getDocumentsParams): Promise<Document<T>[]>
index.getDocument(documentId: string): Promise<Document<T>>
index.deleteDocument(documentId: string | number): Promise<EnqueuedUpdate>
index.deleteDocuments(documentsIds: string[] | number[]): Promise<EnqueuedUpdate>
index.deleteAllDocuments(): Promise<Types.EnqueuedUpdate>
index.getSettings(): Promise<Settings>
index.updateSettings(settings: Settings): Promise<EnqueuedUpdate>
index.resetSettings(): Promise<EnqueuedUpdate>
index.getSynonyms(): Promise<object>
index.updateSynonym(synonyms: object): Promise<EnqueuedUpdate>
index.resetSynonym(): Promise<EnqueuedUpdate>
Get Stop Words
index.getStopWords(): Promise<string[]>
Update Stop Words
index.updateStopWords(string[]): Promise<EnqueuedUpdate>
Reset Stop Words
index.resetStopWords(): Promise<EnqueuedUpdate>
Get Ranking Rules
index.getRankingRules(): Promise<string[]>
Update Ranking Rules
index.updateRankingRules(rankingRules: string[]): Promise<EnqueuedUpdate>
Reset Ranking Rules
index.resetRankingRules(): Promise<EnqueuedUpdate>
Get Distinct Attribute
index.getDistinctAttribute(): Promise<string | void>
Update Distinct Attribute
index.updateDistinctAttribute(distinctAttribute: string): Promise<EnqueuedUpdate>
Reset Distinct Attribute
index.resetDistinctAttribute(): Promise<EnqueuedUpdate>
Get Searchable Attributes
index.getSearchableAttributes(): Promise<string[]>
Update Searchable Attributes
index.updateSearchableAttributes(searchableAttributes: string[]): Promise<EnqueuedUpdate>
Reset Searchable Attributes
index.resetSearchableAttributes(): Promise<EnqueuedUpdate>
Get Displayed Attributes
index.getDisplayedAttributes(): Promise<string[]>
Update Displayed Attributes
index.updateDisplayedAttributes(displayedAttributes: string[]): Promise<EnqueuedUpdate>
Reset Displayed Attributes
index.resetDisplayedAttributes(): Promise<EnqueuedUpdate>
client.getKeys(): Promise<Keys>
client.isHealthy(): Promise<true>
client.stats(): Promise<Stats>
client.version(): Promise<Version>
client.createDump(): Promise<Types.EnqueuedDump>
client.getDumpStatus(dumpUid: string): Promise<Types.EnqueuedDump>
MeiliSearch provides and maintains many SDKs and Integration tools like this one. We want to provide everyone with an amazing search experience for any kind of project. If you want to contribute, make suggestions, or just know what's going on right now, visit us in the integration-guides repository.
FAQs
The Meilisearch JS client for Node.js and the browser.
The npm package meilisearch receives a total of 81,781 weekly downloads. As such, meilisearch popularity was classified as popular.
We found that meilisearch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.