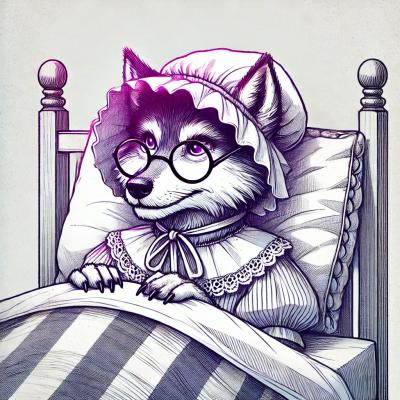
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The memfs npm package is an in-memory filesystem that mimics the Node.js fs module. It allows you to create an ephemeral file system that resides entirely in memory, without touching the actual disk. This can be useful for testing, mocking, and various other scenarios where you don't want to perform I/O operations on the real file system.
Creating and manipulating files
This feature allows you to create, read, and write files in memory as if you were using the native fs module.
const { Volume } = require('memfs');
const vol = new Volume();
vol.writeFileSync('/hello.txt', 'Hello, World!');
const content = vol.readFileSync('/hello.txt', 'utf8');
console.log(content); // Outputs: Hello, World!
Directory operations
This feature enables you to create directories, list their contents, and perform other directory-related operations, all in memory.
const { Volume } = require('memfs');
const vol = new Volume();
vol.mkdirSync('/mydir');
vol.writeFileSync('/mydir/file.txt', 'My file content');
const files = vol.readdirSync('/mydir');
console.log(files); // Outputs: ['file.txt']
Linking and symlinking
This feature allows you to create hard links and symbolic links, mimicking the behavior of links on a real file system.
const { Volume } = require('memfs');
const vol = new Volume();
vol.writeFileSync('/original.txt', 'Content of original');
vol.linkSync('/original.txt', '/link.txt');
vol.symlinkSync('/original.txt', '/symlink.txt');
const linkContent = vol.readFileSync('/link.txt', 'utf8');
const symlinkContent = vol.readlinkSync('/symlink.txt');
console.log(linkContent); // Outputs: Content of original
console.log(symlinkContent); // Outputs: /original.txt
File system watching
This feature provides the ability to watch for changes in the file system, similar to fs.watch in the native fs module.
const { Volume } = require('memfs');
const vol = new Volume();
const fs = vol.promises;
async function watchExample() {
await fs.writeFile('/watched.txt', 'Initial content');
fs.watch('/watched.txt', (eventType, filename) => {
console.log(`Event type: ${eventType}; File: ${filename}`);
});
await fs.writeFile('/watched.txt', 'Updated content');
}
watchExample();
mock-fs is a package that provides a mock file system for Node.js. It is similar to memfs in that it allows you to create an in-memory file system for testing purposes. However, mock-fs overrides the native fs module, while memfs provides a separate file system instance.
unionfs is a package that can combine multiple file systems into a single cohesive file system interface. It can be used with memfs to overlay an in-memory file system on top of the real file system, providing a way to mix real and virtual file system operations.
fs-mock is another in-memory file system module for Node.js. It offers similar functionality to memfs, allowing you to mock the fs module for testing. It differs in API and implementation details, and the choice between fs-mock and memfs may come down to personal preference or specific use-case requirements.
In-memory file-system with Node's fs
API.
fs
API implemented, see old API Status, missing list, missing opendir
Buffer
smemfs-webpack
npm install --save memfs
import { fs } from 'memfs';
fs.writeFileSync('/hello.txt', 'World!');
fs.readFileSync('/hello.txt', 'utf8'); // World!
Create a file system from a plain JSON:
import { fs, vol } from 'memfs';
const json = {
'./README.md': '1',
'./src/index.js': '2',
'./node_modules/debug/index.js': '3',
};
vol.fromJSON(json, '/app');
fs.readFileSync('/app/README.md', 'utf8'); // 1
vol.readFileSync('/app/src/index.js', 'utf8'); // 2
Export to JSON:
vol.writeFileSync('/script.sh', 'sudo rm -rf *');
vol.toJSON(); // {"/script.sh": "sudo rm -rf *"}
Use it for testing:
vol.writeFileSync('/foo', 'bar');
expect(vol.toJSON()).toEqual({ '/foo': 'bar' });
Create as many filesystem volumes as you need:
import { Volume } from 'memfs';
const vol = Volume.fromJSON({ '/foo': 'bar' });
vol.readFileSync('/foo'); // bar
const vol2 = Volume.fromJSON({ '/foo': 'bar 2' });
vol2.readFileSync('/foo'); // bar 2
Use memfs
together with unionfs
to create one filesystem
from your in-memory volumes and the real disk filesystem:
import * as fs from 'fs';
import { ufs } from 'unionfs';
ufs.use(fs).use(vol);
ufs.readFileSync('/foo'); // bar
Use fs-monkey
to monkey-patch Node's require
function:
import { patchRequire } from 'fs-monkey';
vol.writeFileSync('/index.js', 'console.log("hi world")');
patchRequire(vol);
require('/index'); // hi world
spyfs
- spies on filesystem actionsunionfs
- creates a union of multiple filesystem volumeslinkfs
- redirects filesystem pathsfs-monkey
- monkey-patches Node's fs
module and require
functionlibfs
- real filesystem (that executes UNIX system calls) implemented in JavaScriptUnlicense - public domain.
FAQs
In-memory file-system with Node's fs API.
The npm package memfs receives a total of 6,140,402 weekly downloads. As such, memfs popularity was classified as popular.
We found that memfs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.