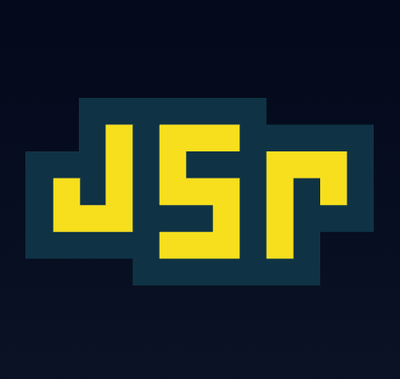
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The memfs npm package is an in-memory filesystem that mimics the Node.js fs module. It allows you to create an ephemeral file system that resides entirely in memory, without touching the actual disk. This can be useful for testing, mocking, and various other scenarios where you don't want to perform I/O operations on the real file system.
Creating and manipulating files
This feature allows you to create, read, and write files in memory as if you were using the native fs module.
const { Volume } = require('memfs');
const vol = new Volume();
vol.writeFileSync('/hello.txt', 'Hello, World!');
const content = vol.readFileSync('/hello.txt', 'utf8');
console.log(content); // Outputs: Hello, World!
Directory operations
This feature enables you to create directories, list their contents, and perform other directory-related operations, all in memory.
const { Volume } = require('memfs');
const vol = new Volume();
vol.mkdirSync('/mydir');
vol.writeFileSync('/mydir/file.txt', 'My file content');
const files = vol.readdirSync('/mydir');
console.log(files); // Outputs: ['file.txt']
Linking and symlinking
This feature allows you to create hard links and symbolic links, mimicking the behavior of links on a real file system.
const { Volume } = require('memfs');
const vol = new Volume();
vol.writeFileSync('/original.txt', 'Content of original');
vol.linkSync('/original.txt', '/link.txt');
vol.symlinkSync('/original.txt', '/symlink.txt');
const linkContent = vol.readFileSync('/link.txt', 'utf8');
const symlinkContent = vol.readlinkSync('/symlink.txt');
console.log(linkContent); // Outputs: Content of original
console.log(symlinkContent); // Outputs: /original.txt
File system watching
This feature provides the ability to watch for changes in the file system, similar to fs.watch in the native fs module.
const { Volume } = require('memfs');
const vol = new Volume();
const fs = vol.promises;
async function watchExample() {
await fs.writeFile('/watched.txt', 'Initial content');
fs.watch('/watched.txt', (eventType, filename) => {
console.log(`Event type: ${eventType}; File: ${filename}`);
});
await fs.writeFile('/watched.txt', 'Updated content');
}
watchExample();
mock-fs is a package that provides a mock file system for Node.js. It is similar to memfs in that it allows you to create an in-memory file system for testing purposes. However, mock-fs overrides the native fs module, while memfs provides a separate file system instance.
unionfs is a package that can combine multiple file systems into a single cohesive file system interface. It can be used with memfs to overlay an in-memory file system on top of the real file system, providing a way to mix real and virtual file system operations.
fs-mock is another in-memory file system module for Node.js. It offers similar functionality to memfs, allowing you to mock the fs module for testing. It differs in API and implementation details, and the choice between fs-mock and memfs may come down to personal preference or specific use-case requirements.
JavaScript file system utilities for Node.js and browser.
npm i memfs
fs
APIexperimental
fs
to File System Access API adapterexperimental
File System Access API to fs
adapterexperimental
crudfs
a CRUD-like file system abstractionexperimental
casfs
Content Addressable Storage file system abstractionsnapshot
utilityprint
directory tree to terminalfs
in browser, creates a .tar
file in real folderfs
in browser, synchronous API, writes to real foldercrudfs
and casfs
in browser and Node.js interoperabilityunionfs
- creates a union of multiple filesystem volumesfs-monkey
- monkey-patches Node's fs
module and require
functionlinkfs
- redirects filesystem pathsspyfs
- spies on filesystem actionsApache 2.0
FAQs
In-memory file-system with Node's fs API.
The npm package memfs receives a total of 10,517,268 weekly downloads. As such, memfs popularity was classified as popular.
We found that memfs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.