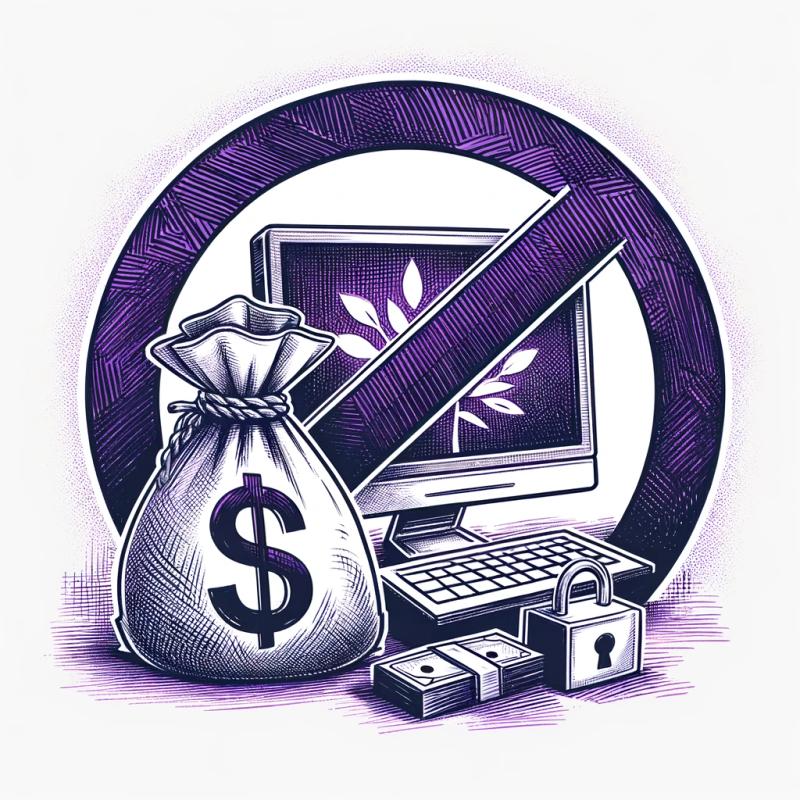
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
memo-cache
Advanced tools
Readme
A memoization and caching library for NodeJS.
$ npm install memo-cache
var memoCache = require('memo-cache');
####Caching: #####memoCache.cache.create(cacheName, options)
cacheName
String - name of the cacheoptions
Object - options for the cache, specifying any of the following:cloneValues
Boolean - should returned values be clones of the original? [Default: false]maxSize
Integer - maximum number of keys to store in this cache; if null, then unlimited [Default: null]Return Value: An object with the following functions to modify the created cache. Please note that these functions do not require the cacheName.
var myCache = memoCache.cache.create('myCache');
console.log(myCache);
// Output:
// { set: [Function], -- function(key, value)
// get: [Function], -- function(key)
// getAll: [Function] -- function ()
// exists: [Function], -- function(key)
// clear: [Function], -- function()
// size: [Function], -- function()
// options: [Function] } -- function()
#####memoCache.cache.set(cacheName, key, value)
cacheName
String - name of the cachekey
String - key to be used to store the valuevalue
Any - value to be stored in the cacheReturn Value: If the item is stored, then the stored value is returned, otherwise null
is returned.
memoCache.cache.set('myCache', 'isExample', true);
// OR (if using the myCache variable from above):
myCache.set('isExample', true);
#####memoCache.cache.get(cacheName, key)
cacheName
String - name of the cachekey
String - key to be used to retrieve the valueReturn Value: If there is an item stored at the given key, then the stored value is returned, otherwise null
is returned.
memoCache.cache.get('myCache', 'isExample'); // => true
memoCache.cache.get('myCache', 'isNotExample'); // => null
// OR (if using the myCache variable from above):
myCache.get('isExample'); // => true
myCache.get('isNotExample'); // => null
#####memoCache.cache.getAll(cacheName)
cacheName
String - name of the cacheReturn Value: If there are items stored in the cache, they will be returned as a JS document.
memoCache.cache.getAll('myCache'); // => {}
memoCache.cache.get('notAValidCache'); // => null
// OR (if using the myCache variable from above):
myCache.getAll('isExample'); // => {}
myCache.getAll('notAValidCache'); // => null
#####memoCache.cache.remove(cacheName, key)
cacheName
String - name of the cachekey
String - key to be deletedReturn Value: If there is an item stored at the given key, then the stored value is returned, otherwise null
is returned.
memoCache.cache.remove('myCache', 'isExample'); // => true
memoCache.cache.remove('myCache', 'isNotExample'); // => null
// OR (if using the myCache variable from above):
myCache.remove('isExample'); // => true
myCache.remove('isNotExample'); // => null
#####memoCache.cache.exists(cacheName, key)
cacheName
String - name of the cachekey
String - key to be checkedReturn Value: If there is an item stored at the given key, then true
is returned, otherwise false
is returned.
memoCache.cache.exists('myCache', 'isExample'); // => true
memoCache.cache.exists('myCache', 'isNotExample'); // => false
// OR (if using the myCache variable from above):
myCache.exists('isExample'); // => true
myCache.exists('isNotExample'); // => false
#####memoCache.cache.clear(cacheName)
cacheName
String - name of the cacheReturn Value: If the cache is cleared, then true
is returned, otherwise false
is returned.
memoCache.cache.clear('myCache'); // => true
// OR (if using the myCache variable from above):
myCache.clear(); // => true
#####memoCache.cache.size(cacheName)
cacheName
String - (optional) name of the cache; If not specified, the size of all caches is returnedReturn Value: The size of the cache(s).
memoCache.cache.size('myCache'); // => 1
memoCache.cache.size(); // => 1
// OR (if using the myCache variable from above):
myCache.size(); // => 1
#####memoCache.cache.options(cacheName)
cacheName
String - name of the cacheReturn Value: If the cache exists, then the options object is returned, otherwise null
is returned.
memoCache.cache.options('myCache'); // => { cloneValues: boolean, maxSize: Number, memoHashFunction: Function }
// OR (if using the myCache variable from above):
myCache.options(); // => { cloneValues: boolean, maxSize: Number, memoHashFunction: Function }
####Memoization: #####memoCache.memoize(function, options)
cacheName
String - name of the cacheoptions
Object - options for the cache, specifying any of the following:cloneValues
Boolean - should returned values be clones of the original? [Default: false]maxSize
Integer - maximum number of keys to store in this cache; if null, then unlimited [Default: null]memoHashFunction
Function - used to map the input arguments to a String. The result of this function becomes the key for the function result valuerequire memoCache = require('memo-cache');
var myFunction = function (aString) { console.log('cache miss!'); return aString; };
var myFunctionMemoized = memoCache.memoize(myFunction, {maxSize: 10});
myFunctionMemoized('testing'); // => 'testing' (Prints 'cache miss!' to the console)
myFunctionMemoized('testing'); // => 'testing' (Does not print 'cache miss!')
$ npm test
Note: This requires mocha
, should
, async
, and underscore
.
FAQs
A memoization and caching library for NodeJS
We found that memo-cache demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).