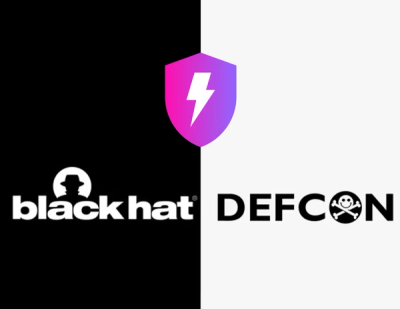
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
memory-cache
Advanced tools
The memory-cache npm package is a simple in-memory caching solution for Node.js applications. It allows you to store and retrieve data quickly without the overhead of a database or external caching service. This can be particularly useful for caching frequently accessed data, reducing the load on your primary data sources, and improving application performance.
Set a cache value
This feature allows you to store a value in the cache with a specified key. The value can be any data type, and it will be stored in memory for quick retrieval.
const cache = require('memory-cache');
cache.put('key', 'value');
Get a cache value
This feature allows you to retrieve a value from the cache using its key. If the key exists in the cache, the corresponding value will be returned; otherwise, it will return null.
const cache = require('memory-cache');
const value = cache.get('key');
Delete a cache value
This feature allows you to delete a value from the cache using its key. This can be useful for invalidating cache entries when the underlying data changes.
const cache = require('memory-cache');
cache.del('key');
Set a cache value with expiration
This feature allows you to store a value in the cache with a specified key and an expiration time in milliseconds. After the expiration time, the value will be automatically removed from the cache.
const cache = require('memory-cache');
cache.put('key', 'value', 10000);
Clear the cache
This feature allows you to clear all entries from the cache. This can be useful for resetting the cache or freeing up memory.
const cache = require('memory-cache');
cache.clear();
node-cache is another in-memory caching solution for Node.js. It offers similar functionality to memory-cache, including setting, getting, and deleting cache values, as well as setting expiration times. One key difference is that node-cache provides additional features such as event listeners for cache hits and misses, and the ability to store multiple values at once.
lru-cache is a caching solution that implements a Least Recently Used (LRU) algorithm. This means that it automatically removes the least recently accessed items when the cache reaches its maximum size. This can be useful for managing memory usage more effectively compared to memory-cache, which does not have built-in eviction policies.
cache-manager is a more advanced caching solution that supports multiple storage backends, including in-memory, Redis, and others. It provides a consistent API for interacting with different types of caches and offers features such as hierarchical caching and custom cache stores. This makes it more versatile than memory-cache, which is limited to in-memory storage.
A simple in-memory cache for node.js
npm install memory-cache --save
var cache = require('memory-cache');
// now just use the cache
cache.put('foo', 'bar');
console.log(cache.get('foo'));
// that wasn't too interesting, here's the good part
cache.put('houdini', 'disappear', 100, function(key, value) {
console.log(key + ' did ' + value);
}); // Time in ms
console.log('Houdini will now ' + cache.get('houdini'));
setTimeout(function() {
console.log('Houdini is ' + cache.get('houdini'));
}, 200);
// create new cache instance
var newCache = new cache.Cache();
newCache.put('foo', 'newbaz');
setTimeout(function() {
console.log('foo in old cache is ' + cache.get('foo'));
console.log('foo in new cache is ' + newCache.get('foo'));
}, 200);
which should print
bar
Houdini will now disappear
houdini did disappear
Houdini is null
foo in old cache is baz
foo in new cache is newbaz
setTimeout
)function(key, value) {}
)null
== size()
unless a setTimeout
removal went wrongexport
into the cacheimport
will remain in the cacheskipDuplicates
is true
options
:
skipDuplicates
: If true
, any duplicate keys will be ignored when importing them. Defaults to false
.require('cache')
would return the default instance of Cacherequire('cache').Cache
is the actual classFAQs
A simple in-memory cache. put(), get() and del()
The npm package memory-cache receives a total of 578,504 weekly downloads. As such, memory-cache popularity was classified as popular.
We found that memory-cache demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.