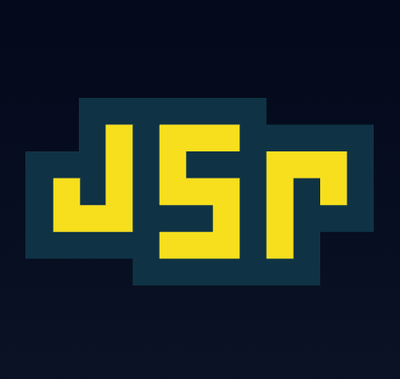
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
micromark-extension-gfm
Advanced tools
The micromark-extension-gfm npm package is an extension for the micromark Markdown parser that adds support for GitHub Flavored Markdown (GFM). This extension enables users to parse and render Markdown text according to the specifications of GFM, which includes several GitHub-specific features such as tables, task lists, and strikethrough.
Tables
This feature allows the parsing of tables in Markdown formatted text. The code sample demonstrates how to convert a simple Markdown table into HTML using the micromark parser with the gfmTable extension.
import {micromark} from 'micromark';
import {gfmTable} from 'micromark-extension-gfm-table';
const markdown = '| Header 1 | Header 2 |\n| -------- | -------- |\n| Cell 1 | Cell 2 |';
const html = micromark(markdown, {extensions: [gfmTable()]});
console.log(html);
Task Lists
This feature supports the rendering of task lists, where tasks can be marked as completed or incomplete. The code sample shows how to convert Markdown task list items into HTML.
import {micromark} from 'micromark';
import {gfmTaskListItem} from 'micromark-extension-gfm-task-list-item';
const markdown = '- [x] Completed task\n- [ ] Incomplete task';
const html = micromark(markdown, {extensions: [gfmTaskListItem()]});
console.log(html);
Strikethrough
This feature enables the use of strikethrough text formatting in Markdown. The code sample illustrates how to parse and convert strikethrough text from Markdown to HTML.
import {micromark} from 'micromark';
import {gfmStrikethrough} from 'micromark-extension-gfm-strikethrough';
const markdown = 'This is ~~strikethrough~~ text.';
const html = micromark(markdown, {extensions: [gfmStrikethrough()]});
console.log(html);
remark-gfm is another npm package that provides support for GitHub Flavored Markdown. It is built on top of the remark Markdown processor and is similar to micromark-extension-gfm in functionality but uses a different underlying Markdown parsing library.
markdown-it is a Markdown parser that can be extended with plugins, including those for GFM features. It is similar to micromark-extension-gfm but offers a different API and plugin ecosystem, potentially providing more customization options for users.
micromark extension to support GitHub flavored markdown. This extension matches either the GFM spec or github.com (default).
This package provides the low-level modules for integrating with the micromark tokenizer and the micromark HTML compiler.
If you’re using micromark
or
mdast-util-from-markdown
, use this package.
Alternatively, if you’re using remark, use remark-gfm
.
If you don’t need all of GFM, the extensions can be used separately:
micromark/micromark-extension-gfm-autolink-literal
— support GFM autolink literalsmicromark/micromark-extension-gfm-footnote
— support GFM footnotesmicromark/micromark-extension-gfm-strikethrough
— support GFM strikethroughmicromark/micromark-extension-gfm-table
— support GFM tablesmicromark/micromark-extension-gfm-tagfilter
— support GFM tagfiltermicromark/micromark-extension-gfm-task-list-item
— support GFM tasklistsThis package is ESM only:
Node 12+ is needed to use it and it must be import
ed instead of require
d.
npm:
npm install micromark-extension-gfm
Say we have the following file, example.md
:
# GFM
## Autolink literals
www.example.com, https://example.com, and contact@example.com.
## Footnote
A note[^1]
[^1]: Big note.
## Strikethrough
~one~ or ~~two~~ tildes.
## Table
| a | b | c | d |
| - | :- | -: | :-: |
## Tag filter
<plaintext>
## Tasklist
* [ ] to do
* [x] done
And our module, example.js
, looks as follows:
import fs from 'node:fs'
import {micromark} from 'micromark'
import {gfm, gfmHtml} from 'micromark-extension-gfm'
const output = micromark(fs.readFileSync('example.md'), {
allowDangerousHtml: true,
extensions: [gfm()],
htmlExtensions: [gfmHtml()]
})
console.log(output)
Now, running node example
yields:
<h1>GFM</h1>
<h2>Autolink literals</h2>
<p><a href="http://www.example.com">www.example.com</a>, <a href="https://example.com">https://example.com</a>, and <a href="mailto:contact@example.com">contact@example.com</a>.</p>
<h2>Footnote</h2>
<p>A note<sup><a href="#user-content-fn-1" id="user-content-fnref-1" data-footnote-ref="" aria-describedby="footnote-label">1</a></sup></p>
<h2>Strikethrough</h2>
<p><del>one</del> or <del>two</del> tildes.</p>
<h2>Table</h2>
<table>
<thead>
<tr>
<th>a</th>
<th align="left">b</th>
<th align="right">c</th>
<th align="center">d</th>
</tr>
</thead>
</table>
<h2>Tag filter</h2>
<plaintext>
<h2>Tasklist</h2>
<ul>
<li><input disabled="" type="checkbox"> to do</li>
<li><input checked="" disabled="" type="checkbox"> done</li>
</ul>
<section data-footnotes="" class="footnotes"><h2 id="footnote-label" class="sr-only">Footnotes</h2>
<ol>
<li id="user-content-fn-1">
<p>Big note. <a href="#user-content-fnref-1" data-footnote-backref="" class="data-footnote-backref" aria-label="Back to content">↩</a></p>
</li>
</ol>
</section>
This package exports the following identifiers: gfm
, gfmHtml
.
There is no default export.
gfm(options?)
gfmHtml
Support GFM or markdown on github.com.
gfm
is a function that can be called with options and returns an extension for
micromark to parse GFM (can be passed in extensions
).
gfmHtml
is a function that can be called and returns an extension for
micromark to compile as elements (can be passed in htmlExtensions
).
options
options.singleTilde
Passed as singleTilde
in
micromark-extension-gfm-strikethrough
.
remarkjs/remark
— markdown processor powered by pluginssyntax-tree/mdast-util-gfm
— mdast utility to support GFMsyntax-tree/mdast-util-from-markdown
— mdast parser using micromark
to create mdast from markdownsyntax-tree/mdast-util-to-markdown
— mdast serializer to create markdown from mdastmicromark/micromark
— the smallest commonmark-compliant markdown parser that existsmicromark/micromark-extension-gfm-autolink-literal
— support GFM autolink literalsmicromark/micromark-extension-gfm-footnote
— support GFM footnotesmicromark/micromark-extension-gfm-strikethrough
— support GFM strikethroughmicromark/micromark-extension-gfm-table
— support GFM tablesmicromark/micromark-extension-gfm-tagfilter
— support GFM tagfiltermicromark/micromark-extension-gfm-task-list-item
— support GFM tasklistsSee contributing.md
in micromark/.github
for ways to get
started.
See support.md
for ways to get help.
This project has a code of conduct. By interacting with this repository, organization, or community you agree to abide by its terms.
FAQs
micromark extension to support GFM (GitHub Flavored Markdown)
We found that micromark-extension-gfm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.