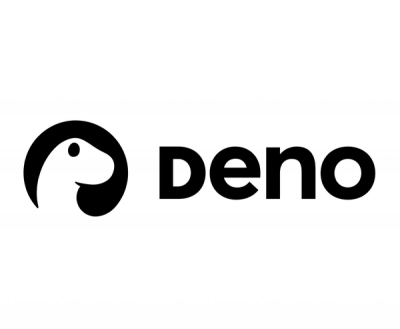
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
JSON-RPC over websocket with multicast service discovery with a browser compatible client
npm install --save microserv
const { Server, Service } = require('microserv')
const server = new Server('my-app', { port: 3000 })
const msgService = new Service('message')
msgService.register('getMessage', () => {
return 'Hello, world!'
})
server.addService(msgService)
server.listen()
server.announce()
const http = require('http')
const { Server, Service } = require('microserv')
const server = new Server('my-app', { port: 3001 })
const mathService = new Service('math')
mathService.register('add', (a, b) => {
return a + b
})
server.addService(mathService)
// Wait for the message service to be ready
server.need('message')
.then(([ message ]) => {
const app = http.createServer((req, res) => {
res.statusCode = 200
res.setHeader('Content-Type', 'text/plain')
// Call the message service `getMessage` method
message.getMessage()
.then(msg => {
res.end(msg)
// { type: 'string', data: 'Hello, world!' }
})
})
app.listen(8080, '127.0.0.1')
})
server.listen()
server.announce()
const { Client } = require('microserv')
const client = new Client()
client.need('message', 'math')
.then(([ message, math ]) => {
message
.getMessage()
.then(msg => console.log(msg))
math
.add(1, 2)
.then(sum => console.log(sum))
})
client.connect('ws://localhost:3000')
client.connect('ws://localhost:3001')
const server = new Server('my-app', { port: 3001 })
Instantiate a client
Parameters:
address
{String}: The service namespace. Only services in the same namespace can connect to each other.options
{Object}: Websocket options
port
{Number}: Websocket portinterval
{Number}: How often to announce the service (in ms). Defaults to 5000
.sever
{http.Server|https.server}: A server to use as the websocket server. If set, will override port
.secure
{Boolean}: Use secure websockets. Defaults to false
.serviceTransform
{Function}: A function to transform the data returned from an rpc call. Defaults to noop.authorization
{Any}: Credentials to pass to Server when connectingauthorizeClient
{Function}: A sync or async function to authorize a Client. Function is passed the Client's authorization
credentialsdns
{Object}: dns-discovery optionsAdd a service to the server, and announce the service to connected peers
Parameters:
service
{Service}: A Service instanceList required services. Resolves with each of the required services of type ClientService
Parameters:
services
{String}: Service namesBegin listening for peers
Announce the service on the network. Will reannounce the service at the interval provided via opts
.
Emitted when there is an error on the server
Emitted when a connected socket is reset
const service = new Service('my-service')
Instantiate a new Service
Parameters:
name
{String}: The service name that will be announced to peersRegister an rpc method with the service
Parameters:
method
{String}: The name of the methodcb
{Function}: The function to invoke when the rpc method is calledresultType
{String}: The type of data returned by the method. Defaults to typeof result
Emits an event via the websocket
Parameters:
name
{String}: The event namedata
{*}: The event dataReturned from server.need()
. This should not be instantiated direclty.
Subscribe to a service event
Parameters:
name
{String}: The name of the eventcb
{Function}: The event callbackEach method registered with the service is exposed a method of the client service. Returns and object with data
and type
properties.
Emitted when the connection to the service is lost
Emitted when the connection to a lost service is reestablished
const client = new Client()
Instantiate a new Client
Parameters:
opts
{Object}: Options
serviceTransform
{Function}: A function to transform the data returned from an rpc call. Defaults to noop.authorization
{Any}: Credentials to pass to Server when connectingrpcOpts
{Object}: Options for rpc-websocketsConnect to a specific websocket server
Parameters:
connection
{String}: The server connection string (eg. ws://localhost:3001)List required services. Resolves with each of the required services of type ClientService
Parameters:
services
{String}: Service namesEmitted when the connection to a service is closed
Emitted when the client fails authorization
npm run test
FAQs
JSON-RPC over websocket with multicast service discovery.
The npm package microserv receives a total of 4 weekly downloads. As such, microserv popularity was classified as not popular.
We found that microserv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.