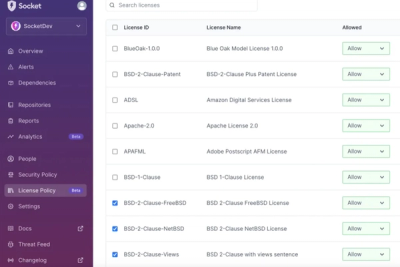
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
mikro-orm
Advanced tools
TypeScript ORM for Node.js based on Data Mapper, Unit of Work and Identity Map patterns. Supports MongoDB, MySQL, PostgreSQL and SQLite databases as well as usage with vanilla JavaScript.
Mikro-ORM is a TypeScript ORM for Node.js based on Data Mapper, Unit of Work, and Identity Map patterns. It supports multiple databases including MongoDB, MySQL, PostgreSQL, SQLite, and MariaDB. It provides a powerful and flexible way to interact with your database using TypeScript and JavaScript.
Entity Definition
Defines an entity with properties that map to database columns. The `@Entity` decorator marks the class as a database entity, while `@PrimaryKey` and `@Property` decorators define the primary key and other properties respectively.
```typescript
import { Entity, PrimaryKey, Property } from 'mikro-orm';
@Entity()
class User {
@PrimaryKey()
id!: number;
@Property()
name!: string;
@Property()
email!: string;
}
```
CRUD Operations
Demonstrates basic Create, Read, Update, and Delete (CRUD) operations. The `persistAndFlush` method saves a new entity to the database, and the `find` method retrieves entities from the database.
```typescript
const user = new User();
user.name = 'John Doe';
user.email = 'john.doe@example.com';
await orm.em.persistAndFlush(user);
const users = await orm.em.find(User, {});
console.log(users);
```
Query Builder
Shows how to use the query builder to construct and execute complex queries. The `createQueryBuilder` method creates a new query builder instance, and the `select` and `where` methods build the query.
```typescript
const qb = orm.em.createQueryBuilder(User);
const users = await qb.select('*').where({ name: 'John Doe' }).execute();
console.log(users);
```
Transactions
Illustrates how to perform operations within a transaction. The `transactional` method ensures that all operations within the callback are executed within a single transaction.
```typescript
await orm.em.transactional(async em => {
const user = new User();
user.name = 'Jane Doe';
user.email = 'jane.doe@example.com';
await em.persistAndFlush(user);
});
```
TypeORM is another TypeScript ORM for Node.js that supports Active Record and Data Mapper patterns. It is highly popular and supports multiple databases. Compared to Mikro-ORM, TypeORM has a larger community and more extensive documentation, but Mikro-ORM offers a more modern and flexible API.
Sequelize is a promise-based Node.js ORM for Postgres, MySQL, MariaDB, SQLite, and Microsoft SQL Server. It is known for its simplicity and ease of use. While Sequelize is more mature and has a larger user base, Mikro-ORM provides better TypeScript support and more advanced features like Unit of Work and Identity Map patterns.
Objection.js is an SQL-friendly ORM for Node.js, built on top of the SQL query builder Knex.js. It aims to stay as close to the SQL syntax as possible while providing a powerful and flexible API. Compared to Mikro-ORM, Objection.js offers more control over raw SQL queries but lacks some of the higher-level abstractions and features provided by Mikro-ORM.
TypeScript ORM for Node.js based on Data Mapper, Unit of Work and Identity Map patterns. Supports MongoDB, MySQL, MariaDB, PostgreSQL and SQLite (including libSQL) databases.
You might be asking: What the hell is Unit of Work and why should I care about it?
Unit of Work maintains a list of objects (entities) affected by a business transaction and coordinates the writing out of changes. (Martin Fowler)
Identity Map ensures that each object (entity) gets loaded only once by keeping every loaded object in a map. Looks up objects using the map when referring to them. (Martin Fowler)
So what benefits does it bring to us?
First and most important implication of having Unit of Work is that it allows handling transactions automatically.
When you call em.flush()
, all computed changes are queried inside a database transaction (if supported by given driver). This means that you can control the boundaries of transactions simply by calling em.persistLater()
and once all your changes are ready, calling flush()
will run them inside a transaction.
You can also control the transaction boundaries manually via
em.transactional(cb)
.
const user = await em.findOneOrFail(User, 1);
user.email = 'foo@bar.com';
const car = new Car();
user.cars.add(car);
// thanks to bi-directional cascading we only need to persist user entity
// flushing will create a transaction, insert new car and update user with new email
// as user entity is managed, calling flush() is enough
await em.flush();
MikroORM allows you to implement your domain/business logic directly in the entities. To maintain always valid entities, you can use constructors to mark required properties. Let's define the User
entity used in previous example:
@Entity()
export class User {
@PrimaryKey()
id!: number;
@Property()
name!: string;
@OneToOne(() => Address)
address?: Address;
@ManyToMany(() => Car)
cars = new Collection<Car>(this);
constructor(name: string) {
this.name = name;
}
}
Now to create new instance of the User
entity, we are forced to provide the name
:
const user = new User('John Doe'); // name is required to create new user instance
user.address = new Address('10 Downing Street'); // address is optional
Once your entities are loaded, make a number of synchronous actions on your entities,
then call em.flush()
. This will trigger computing of change sets. Only entities
(and properties) that were changed will generate database queries, if there are no changes,
no transaction will be started.
const user = await em.findOneOrFail(User, 1, {
populate: ['cars', 'address.city'],
});
user.title = 'Mr.';
user.address.street = '10 Downing Street'; // address is 1:1 relation of Address entity
user.cars.getItems().forEach(car => car.forSale = true); // cars is 1:m collection of Car entities
const car = new Car('VW');
user.cars.add(car);
// now we can flush all changes done to managed entities
await em.flush();
em.flush()
will then execute these queries from the example above:
begin;
update "user" set "title" = 'Mr.' where "id" = 1;
update "user_address" set "street" = '10 Downing Street' where "id" = 123;
update "car"
set "for_sale" = case
when ("id" = 1) then true
when ("id" = 2) then true
when ("id" = 3) then true
else "for_sale" end
where "id" in (1, 2, 3)
insert into "car" ("brand", "owner") values ('VW', 1);
commit;
Thanks to Identity Map, you will always have only one instance of given entity in one context. This allows for some optimizations (skipping loading of already loaded entities), as well as comparison by identity (ent1 === ent2
).
MikroORM documentation, included in this repo in the root directory, is built with Docusaurus and publicly hosted on GitHub Pages at https://mikro-orm.io.
There is also auto-generated CHANGELOG.md file based on commit messages (via semantic-release
).
QueryBuilder
You can find example integrations for some popular frameworks in the mikro-orm-examples
repository:
First install the module via yarn
or npm
and do not forget to install the database driver as well:
Since v4, you should install the driver package, but not the db connector itself, e.g. install
@mikro-orm/sqlite
, but notsqlite3
as that is already included in the driver package.
yarn add @mikro-orm/core @mikro-orm/mongodb # for mongo
yarn add @mikro-orm/core @mikro-orm/mysql # for mysql/mariadb
yarn add @mikro-orm/core @mikro-orm/mariadb # for mysql/mariadb
yarn add @mikro-orm/core @mikro-orm/postgresql # for postgresql
yarn add @mikro-orm/core @mikro-orm/mssql # for mssql
yarn add @mikro-orm/core @mikro-orm/sqlite # for sqlite
yarn add @mikro-orm/core @mikro-orm/better-sqlite # for better-sqlite
yarn add @mikro-orm/core @mikro-orm/libsql # for libsql
or
npm i -s @mikro-orm/core @mikro-orm/mongodb # for mongo
npm i -s @mikro-orm/core @mikro-orm/mysql # for mysql/mariadb
npm i -s @mikro-orm/core @mikro-orm/mariadb # for mysql/mariadb
npm i -s @mikro-orm/core @mikro-orm/postgresql # for postgresql
npm i -s @mikro-orm/core @mikro-orm/mssql # for mssql
npm i -s @mikro-orm/core @mikro-orm/sqlite # for sqlite
npm i -s @mikro-orm/core @mikro-orm/better-sqlite # for better-sqlite
npm i -s @mikro-orm/core @mikro-orm/libsql # for libsql
Next, if you want to use decorators for your entity definition, you will need to enable support for decorators as well as esModuleInterop
in tsconfig.json
via:
"experimentalDecorators": true,
"emitDecoratorMetadata": true,
"esModuleInterop": true,
Alternatively, you can use EntitySchema
.
Then call MikroORM.init
as part of bootstrapping your app:
To access driver specific methods like
em.createQueryBuilder()
we need to specify the driver type when callingMikroORM.init()
. Alternatively we can cast theorm.em
toEntityManager
exported from the driver package:
import { EntityManager } from '@mikro-orm/postgresql'; const em = orm.em as EntityManager; const qb = em.createQueryBuilder(...);
import type { PostgreSqlDriver } from '@mikro-orm/postgresql'; // or any other SQL driver package
const orm = await MikroORM.init<PostgreSqlDriver>({
entities: ['./dist/entities'], // path to your JS entities (dist), relative to `baseDir`
dbName: 'my-db-name',
type: 'postgresql',
});
console.log(orm.em); // access EntityManager via `em` property
There are more ways to configure your entities, take a look at installation page.
Read more about all the possible configuration options in Advanced Configuration section.
Then you will need to fork entity manager for each request so their identity maps will not collide. To do so, use the RequestContext
helper:
const app = express();
app.use((req, res, next) => {
RequestContext.create(orm.em, next);
});
You should register this middleware as the last one just before request handlers and before any of your custom middleware that is using the ORM. There might be issues when you register it before request processing middleware like
queryParser
orbodyParser
, so definitely register the context after them.
More info about RequestContext
is described here.
Now you can start defining your entities (in one of the entities
folders). This is how simple entity can look like in mongo driver:
./entities/MongoBook.ts
@Entity()
export class MongoBook {
@PrimaryKey()
_id: ObjectID;
@SerializedPrimaryKey()
id: string;
@Property()
title: string;
@ManyToOne(() => Author)
author: Author;
@ManyToMany(() => BookTag)
tags = new Collection<BookTag>(this);
constructor(title: string, author: Author) {
this.title = title;
this.author = author;
}
}
For SQL drivers, you can use id: number
PK:
./entities/SqlBook.ts
@Entity()
export class SqlBook {
@PrimaryKey()
id: number;
}
Or if you want to use UUID primary keys:
./entities/UuidBook.ts
import { v4 } from 'uuid';
@Entity()
export class UuidBook {
@PrimaryKey()
uuid = v4();
}
More information can be found in defining entities section in docs.
When you have your entities defined, you can start using ORM either via EntityManager
or via EntityRepository
s.
To save entity state to database, you need to persist it. Persist takes care or deciding whether to use insert
or update
and computes appropriate change-set. Entity references that are not persisted yet (does not have identifier) will be cascade persisted automatically.
// use constructors in your entities for required parameters
const author = new Author('Jon Snow', 'snow@wall.st');
author.born = new Date();
const publisher = new Publisher('7K publisher');
const book1 = new Book('My Life on The Wall, part 1', author);
book1.publisher = publisher;
const book2 = new Book('My Life on The Wall, part 2', author);
book2.publisher = publisher;
const book3 = new Book('My Life on The Wall, part 3', author);
book3.publisher = publisher;
// just persist books, author and publisher will be automatically cascade persisted
await em.persistAndFlush([book1, book2, book3]);
To fetch entities from database you can use find()
and findOne()
of EntityManager
:
const authors = em.find(Author, {}, { populate: ['books'] });
for (const author of authors) {
console.log(author); // instance of Author entity
console.log(author.name); // Jon Snow
for (const book of author.books) { // iterating books collection
console.log(book); // instance of Book entity
console.log(book.title); // My Life on The Wall, part 1/2/3
}
}
More convenient way of fetching entities from database is by using EntityRepository
, that carries the entity name, so you do not have to pass it to every find
and findOne
calls:
const booksRepository = em.getRepository(Book);
const books = await booksRepository.find({ author: '...' }, {
populate: ['author'],
limit: 1,
offset: 2,
orderBy: { title: QueryOrder.DESC },
});
console.log(books); // Loaded<Book, 'author'>[]
Take a look at docs about working with EntityManager
or using EntityRepository
instead.
Contributions, issues and feature requests are welcome. Please read CONTRIBUTING.md for details on the process for submitting pull requests to us.
👤 Martin Adámek
See also the list of contributors who participated in this project.
Please ⭐️ this repository if this project helped you!
Copyright © 2018 Martin Adámek.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
TypeScript ORM for Node.js based on Data Mapper, Unit of Work and Identity Map patterns. Supports MongoDB, MySQL, PostgreSQL and SQLite databases as well as usage with vanilla JavaScript.
The npm package mikro-orm receives a total of 144,585 weekly downloads. As such, mikro-orm popularity was classified as popular.
We found that mikro-orm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.