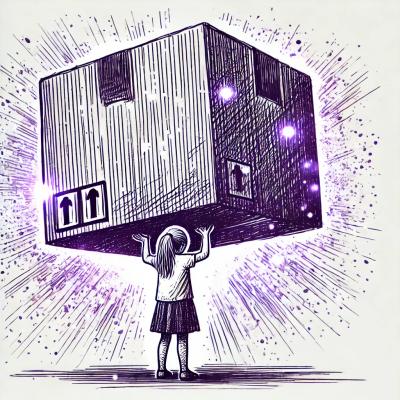
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
mobservable
Advanced tools
Changes are coming! Small library for creating observable properties en functions
Changes are coming!
MOBservable is simple observable implementation, based on the ideas of observables in bigger frameworks like knockout
, ember
etc, but this one does not have 'strings attached'. Furthermore it should fit well in any typescript project.
The mobservable.property
method takes a value or function and creates an observable value from it.
This way properties that listen that observe each other can be created. A quick example:
import mobservable = require('mobservable');
var property = mobservable.property;
var vat = property(0.20);
var order = {};
order.price = property(10),
order.priceWithVat = property(() => order.price() * (1+vat()));
order.priceWithVat.subscribe((price) => console.log("New price: " + price));
order.price(20);
// Prints: New price: 24
order.price(10);
// Prints: New price: 10
Constructs a new Property
, value can either be a string, number, boolean or function that takes no arguments and returns a value. In the body of the function, references to other properties will be tracked, and on change, the function will be re-evaluated. The returned value is an IProperty
function/object.
Optionally accepts a scope parameter, which will be returned by the setter for chaining, and which will used as scope for calculated properties.
Defines a property using ES5 getters and setters. This is useful in constructor functions, and allows for direct assignment / reading from observables:
var vat = property(0.2);
var Order = function() {
mobservable.defineProperty(this, 'price', 20);
mobservable.defineProperty(this, 'amount', 2);
mobservable.defineProperty(this, 'total', function() {
return (1+vat()) * this.price * this.amount; // price and amount are now properties!
});
};
var order = new Order();
order.price = 10;
order.amount = 3;
// order.total now equals 36
In typescript, it might be more convenient for the typesystem to directly define getters and setters instead of using mobservable.defineProperty
:
class Order {
_price = new mobservable.property(20, this);
get price() {
return this._price();
}
set price(value) {
this._price(value);
}
}
guard
invokes func
and returns a tuple consisting of the return value of func
and an unsubscriber. guard
will track which observables func
was observing, but it will not recalculate func
if necessary, instead, it will fire the onInvalidate
callback to notify that the output of func
can no longer be trusted.
The onInvalidate
function will be called only once, after that, the guard has finished. To abort a guard, use the returned unsubscriber.
Guard is useful in functions where you want to have func
observable, but func is actually invoked as side effect or part of a bigger change flow or where unnecessary recalculations of func
or either pointless or expensive.
Batch postpones the updates of computed properties until the (synchronous) workerFunction
has completed. This is useful if you want to apply a bunch of different updates throughout your model before needing the updated computed values, for example while refreshing a value from the database.
The listener is invoked each time the complete model has become stable. The listener is always invoked asynchronously, so that even without batch
the listener is only invoked after a bunch of changes have been applied
onReady
returns a function with wich the listener can be unsubscribed from future events
Returns the current value of the property
Sets a new value to the property. Returns the scope with which this property was created for chaining.
Registers a new listener to change events. Listener should be a function, its first argument will be the new value, and second argument the old value.
Returns a function that upon invocation unsubscribes the listener from the property.
FAQs
Observable data. Reactive functions. Simple code.
The npm package mobservable receives a total of 40 weekly downloads. As such, mobservable popularity was classified as not popular.
We found that mobservable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.