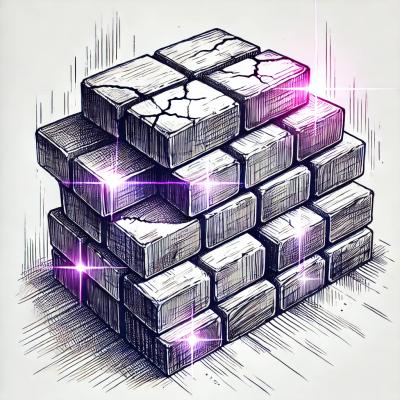
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
mobx-decorated-models
Advanced tools
mobx-decorated-models is a collection of es7 decorators to make a class observable and serializable.
Mobx makes state management super simple, but it doesn't offer an opinion on how to get data in and out of the observed data structures.
Serializr takes care of that nicely.
Combining the two libraries isn’t difficult, but then you end up specifing each attribute twice; once so Mobx will observe it, and once to create a schema for Serializr.
This library is a collection of decorators that co-ordinates making fields both observable and serializable.
While it’s at it, it also handles model lookups so different models can refer to one another regardless of import order. When one model refers to another, a reference of the requirement is stored and then later resolved when the class becomes known.
import { model, field, session, belongsTo, hasMany, identifier } from 'mobx-decorated-models';
@modelDecorator
export class Box {
@identifier id;
@field width = 0;
@field height = 0;
@field depth = 0;
@computed get volume() {
return this.width * this.height * this.depth;
}
@hasMany items;
@belongsTo container;
@belongsTo({ model: 'Address' }) warehouse;
}
additional examples used for testing are located in specs/test-models.js
boxes = @observable.array([])
fetch('/my/api/endpoints/boxes/1.json').then(function(response) {
boxes.concat(Box.deserialize(response.json()));
});
const box = Box.deserialize({ id: 1, width: 2, height: 3, depth: 8 }); // returns an instance of Box
console.log(box.volume); // => 48
console.log(box.serialize()); // => { id: 1, width: 2, height: 3, depth: 8, items: [] }
By default, the class @modelDecorator
uses the name
property of each class as a lookup key so
that hasMany
and belongsTo
relation ships can be established.
This allows things like the below mappings to still work even though the two files can't easily include each other:
// chair.js
import { modelDecorator, belongsTo } from 'mobx-decorated-models';
@modelDecorator
class Chair {
belongsTo 'table'
}
// table.js
import { model, hasMany } from 'mobx-decorated-models';
@modelDecorator
class Table {
hasMany({ model: 'Chair' }) 'seats'
}
This works well enough, however using the name
property is fragile, since it relies on the class name
not changing. Certain JS minimizers may rename classes.
If custom logic is needed, it's possible to supply custom "record" and "lookup" functions.
Example that uses a static identifiedBy
property.
import { model, lookupModelUsing, rememberModelUsing } from 'mobx-decorated-models';
import { capitalize, singularize } from 'utility';
const Models = {};
lookupModelUsing((propertyName, propertyOptions) => {
return Models[propertyOptions.className] ||
Models[capitalize(propertyName)] ||
Models[capitalize(singularize(propertyName))];
});
rememberModelUsing(klass => Models[klass.identifiedBy] = klass);
@modelDecorator
class ATestingModel {
static identifiedBy = 'test';
belongsTo document;
}
@modelDecorator
class Document {
static identifiedBy = 'document';
hasMany({ className: 'test' }) testRuns;
}
Marks a class as serializable.
It adds a few convenience methods to classes:
deserialize
method. Used to turn JSON structure into a model (or collection of models)update
method. Updates a model's attributes and child associations.serialize
. Converts the model's attributes and it's associations to JSON.However, it's primary purpose is to remember classes for hasMany/belongsTo lookups. See discussion
above regarding lookupModelUsing
and rememberModelUsing
.
The primary key for the model
marks a class property as observable and serializable.
The type of field can be set to array
or object
by specifying options.
example:
class Foo {
@field({ type: 'object' }) options; // will default to an observable map
@field({ type: 'array' }) tags; // defaults to []
}
const foo = new Foo();
foo.tags.push('one');
foo.options.set('one', 1);
foo.serialize(); // => { tags: ['one'], options: { one: 1 } }
Makes a property as referring to another model. Will attempt to map
the referenced class based on the name, i.e. a property named box
will
look for a class named Box
.
Optionally can be given an option object with a className
property to control the mapping.
example:
class Person({
@identifier id;
@field name;
@belongsTo({ className: 'Pants', inverseOf: 'owner' }) outfit;
})
class Pants {
@session color;
@belongsTo({ className: 'Person' }) owner; // looks for class `Person`
}
Can be given a inverseOf
which will set auto set this property to it's parent when it's deserialized.
For instance the Pants model above will have it's owner property set to the "Ralph" Person model:
Person.deserialize({
id: 1, name: 'Ralph', outfit: { color: 'RED' }
})
Note: When using inverseOf
, the auto-set property is not serialized in order to prevent circular references.
Marks a property as belonging to an mobx observable array of models.
Sets the default value to an empty observable array
As in belongsTo
, can be optionally given an option object with a className
property to control the mapping.
hasMany
also accepts inverseOf
and defaults
properties. If an inverseOf is provided,
when a model is added to the array, it will have the property named by inverseOf
to the parent model
If defaults
are provided the new model's attributes will be defaulted to them. defaults
may
also be a function, which will be called and it's return values used.
class Tire {
@session numberInSet;
@belongsTo vehicle; // will be autoset by the `inverseOf: auto` on Car
}
class Car {
@belongsTo home;
@session color;
@hasMany({ className: 'Tire', inverseOf: 'vehicle', defaults: {numberInSet: 4} }) tires;
}
class Garage {
@session owner;
@hasMany({
className: 'Car',
inverseOf: 'home',
defaults(collection, parent) {
return { color: this.owner.favoriteColor };
}
}) cars;
}
FAQs
Decorators to make using Mobx for model type structures easier
The npm package mobx-decorated-models receives a total of 21 weekly downloads. As such, mobx-decorated-models popularity was classified as not popular.
We found that mobx-decorated-models demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.