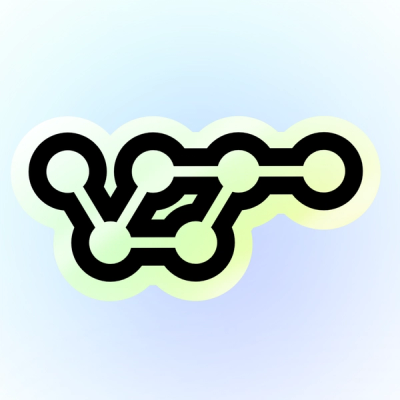
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
mobx-react
Advanced tools
The mobx-react package provides React bindings for MobX, a state management library. It allows you to connect your React components to MobX observables, making it easier to manage and react to state changes in your application.
Observer Component
The observer function is used to create a reactive component that will automatically re-render when the observed state changes.
import React from 'react';
import { observer } from 'mobx-react';
import { observable } from 'mobx';
const appState = observable({
count: 0
});
const Counter = observer(() => (
<div>
<button onClick={() => appState.count++}>Increment</button>
<p>{appState.count}</p>
</div>
));
export default Counter;
Injecting Stores
The inject function is used to inject stores into components, making it easier to access MobX stores within your React components.
import React from 'react';
import { Provider, inject, observer } from 'mobx-react';
import { observable } from 'mobx';
class Store {
@observable count = 0;
}
const store = new Store();
const Counter = inject('store')(observer(({ store }) => (
<div>
<button onClick={() => store.count++}>Increment</button>
<p>{store.count}</p>
</div>
)));
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
export default App;
Using MobX with React Hooks
The useLocalObservable hook is used to create local observable state within a functional component, allowing you to use MobX with React hooks.
import React from 'react';
import { useLocalObservable, observer } from 'mobx-react';
const Counter = observer(() => {
const state = useLocalObservable(() => ({
count: 0,
increment() {
this.count++;
}
}));
return (
<div>
<button onClick={state.increment}>Increment</button>
<p>{state.count}</p>
</div>
);
});
export default Counter;
Redux is a popular state management library for JavaScript applications. Unlike MobX, which uses observables and decorators, Redux relies on a single immutable state tree and pure reducer functions to manage state. Redux is often used with the react-redux bindings to connect React components to the Redux store.
Recoil is a state management library for React that provides a more fine-grained approach to state management compared to Redux. It allows you to create atoms and selectors to manage state and derive state. Recoil is designed to work seamlessly with React's concurrent mode and hooks.
Zustand is a small, fast, and scalable state management library for React. It uses hooks to create and manage state, providing a simple and intuitive API. Unlike MobX, which uses observables, Zustand relies on React's built-in state management capabilities.
Package with react component wrapper for combining React with mobx.
Exports the observer
decorator and some development utilities.
For documentation, see the mobx project.
This package supports both React and React-Native.
npm install mobx-react --save
import {observer} from 'mobx-react';
// - or -
import {observer} from 'mobx-react/native';
This package provides the bindings for MobX and React. See the official documentation for how to get started.
Function (and decorator) that converts a React component definition, React component class or stand-alone render function into a reactive component. See the mobx documentation for more details.
import {observer} from "mobx-react";
// ---- ES5 syntax ----
const TodoView = observer(React.createClass({
displayName: "TodoView",
render() {
return <div>this.props.todo.title</div>
}
}));
// ---- ES6 syntax ----
@observer class TodoView extends React.Component {
render() {
return <div>this.props.todo.title</div>
}
}
// ---- or just use a stateless component function: ----
const TodoView = observer(({todo}) => <div>{todo.title}</div>)
Should I use observer
for each component?
You should use observer
on every component that displays observable data.
Even the small ones. observer
allows components to render independently from their parent and in general this means that
the more you use observer
, the better the performance become.
The overhead of observer
itself is neglectable.
See also Do child components need @observer
?
I see React warnings about forceUpdate
/ setState
from React
The following warning will appear if you trigger a re-rendering between instantiating and rendering a component:
Warning: forceUpdate(...): Cannot update during an existing state transition (such as within
render). Render methods should be a pure function of props and state.
Usually this means that (another) component is trying to modify observables used by this components in their constructor
or getInitialState
methods.
This violates the React Lifecycle, componentWillMount
should be used instead if state needs to be modified before mounting.
Enables the tracking from components. Each rendered reactive component will be added to the componentByNodeRegistery
and its renderings will be reported through the renderReporter
event emitter.
Event emitter that reports render timings and component destructions. Only available after invoking trackComponents()
.
New listeners can be added through renderReporter.on(function(data) { /* */ })
.
Data will have one of the following formats:
{
event: 'render',
renderTime: /* time spend in the .render function of a component, in ms. */,
totalTime: /* time between starting a .render and flushing the changes to the DOM, in ms. */,
component: /* component instance */,
node: /* DOM node */
}
{
event: 'destroy',
component: /* component instance */,
node: /* DOM Node */
}
WeakMap. It's get
function returns the associated reactive component of the given node. The node needs to be precisely the root node of the component.
This map is only available after invoking trackComponents
.
The debug name stateless function components of babel transpiled jsx are now properly picked up if the wrapper is applied after defining the component:
const MyComponent = () => <span>hi</span>
export default observer(MyComponent);
Removed peer dependencies, React 15 (and 0.13) are supported as well. By @bkniffler
Removed the warning introduced in 3.0.1. It triggered always when using shallow rendering (when using shallow rendering componentDidMount
won't fire. See https://github.com/facebook/react/issues/4919).
Added warning when changing state in getInitialState
/ constructor
.
Upgraded to MobX 2.0.0
Improved typescript typings overloads of observer
Added empty 'dependencies' section to package.json, fixes #26
Added support for context to stateless components. (by Kosta-Github).
Fixed #12: fixed React warning when a component was unmounted after scheduling a re-render but before executing it.
Upped dependency of mobx to 1.1.1.
It is now possible to define propTypes
and getDefaultProps
on a stateless component:
const myComponent = (props) => {
// render
};
myComponent.propTypes = {
name: React.PropTypes.string
};
myComponent.defaultProps = {
name: "World"
};
export default observer(myComponent);
All credits to Jiri Spac for this contribution!
Use React 0.14 instead of React 0.13. For React 0.13, use version mobx-react@1.0.2
or higher.
Minor fixes and improvements
Fixed issue with typescript typings. An example project with MobX, React, Typescript, TSX can be found here: https://github.com/mobxjs/mobx-react-typescript
reactiveComponent
has been renamed to observer
Added separte import for react-native: use var reactiveComponent = require('mobx-react/native').reactiveComponent
for native support; webpack clients will refuse to build otherwise.
Added react-native as dependency, so that the package works with either react
or react-native
.
Upgraded to MobX 0.7.0
Fixed issue where Babel generated component classes where not properly picked up.
observer
now accepts a pure render function as argument, besides constructor function. For example:
var TodoItem = observer(function TodoItem(props) {
var todo = props.todo;
return <li>{todo.task}</li>;
});
observer is now defined in terms of side effects.
Added support for React 0.14(RC) by dropping peer dependency
FAQs
React bindings for MobX. Create fully reactive components.
The npm package mobx-react receives a total of 873,151 weekly downloads. As such, mobx-react popularity was classified as popular.
We found that mobx-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.