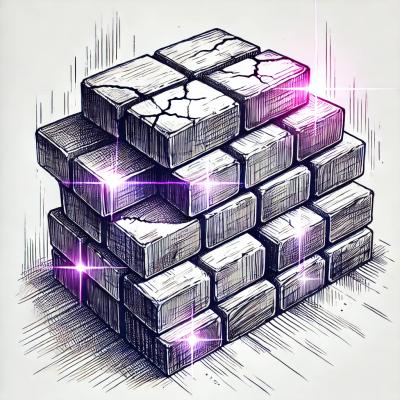
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
mockdata-generator
Advanced tools
Collection of data generators to create complex data structures and any number of records
A library of mock data object generator. Allows developers and users to define real life looking mock data.
npm install mockdata-generator --save-dev
The following is a complete program that generates 10 records of data. Each of the valid dataTypes is included in the example
/**
* This will get the constructor of the main class
* The constructor accepts a configuration object with these properties
* 'name': An optional name of the object
* 'metadata': An array of descriptions for each of the attributes of the object
**/
"use strict";
var Generator,
meta,
gen,
pretty,
value;
Generator = require('./index.js').Generator;
pretty = require('js-object-pretty-print').pretty;
meta = {
'name': 'Mockdata test set',
'metadata': [
{
'columnName': 'creditCards',
'dataType': 'Array',
'metadata': [
{
'columnName': 'ccNumber',
'dataType': 'Pattern',
'pattern': '####-####-####-####'
},
{
'columnName': 'cvv',
'dataType': 'Pattern',
'pattern': '###'
},
{
'columnName': 'expiration',
'dataType': 'Date',
'min': 30,
'max': 1000
},
{
'columnName': 'limit',
'dataType': 'OneOfList',
'list': [500, 1000, 2000, 5000, 10000],
'mode': 'shuffle'
}
],
'min': 2,
'max': 5
},
{
'columnName': 'balance',
'dataType': 'Currency',
'min': 50,
'max': 1000
},
{
'columnName': 'txDate',
'dataType': 'Date',
'min': 10,
'max': 30
},
{
'columnName': 'id',
'dataType': 'Guid',
'prefix': 'test'
},
{
'columnName': 'branches',
'dataType': 'Integer',
'min': 10,
'max': 30
},
{
'columnName': 'description',
'dataType': 'Ipsum',
'paragraphNum': 1,
'wordMin': 10,
'wordMax': 30
},
{
'columnName': 'accountOwner',
'dataType': 'Name',
'order': 'fl'
},
{
'columnName': 'beneficiaryContact',
'dataType': 'Name',
'order': 'lf'
},
{
'columnName': 'averageTxPerDay',
'dataType': 'Number',
'min': 5,
'max': 20
},
{
'columnName': 'beneficiary',
'dataType': 'OneOfList',
'listName': 'ListBase.Beneficiaries',
'mode': 'sequence',
'index': 0
},
{
'columnName': 'clothesCategory',
'dataType': 'OneOfList',
'list': ['Accessories', 'Blouses', 'Cardigans', 'Dresses', 'Skirts'],
'mode': 'shuffle'
},
{
'columnName': 'accountNumber',
'dataType': 'Pattern',
'pattern': '###-AA-###-aaa-####'
},
{
'columnName': 'accountName',
'dataType': 'String',
'min': 6,
'max': 30
}
]
};
gen = new Generator(meta);
value = gen.getValue(10);
process.stdout.write(pretty(value));
mockdata-generator provides a way to generate data in the following dataTypes
The base clase for all the other generators. It implements several attributes and methods. Base is not used to generate data and it provides all the common functionality for the other data types
Attribute | Default | Description |
---|---|---|
columnName | n/a | Identifies the attribute inside the object. It must be unique for the object |
dataType | The data type of the attribute. Any of the names in the dataType list above | |
min | 0 | The minimum value of a range. Not all the data types require a min |
max | 1 | The maximum value of a range. Not all the data types require a max |
Generates a number of object values in the range of (min, max).
Attribute | Default | Description |
---|---|---|
metadata | n/a | An array of metadata descriptors for the attributes in each object |
min | 1 | The minimum number of values to generate |
max | 1 | The maximum number of values to generate |
Generates a random numeric value in the (min, max) range, assign one of the listed currencies and generates an object with three attributes: currency, value and formatted value, for instance
{
currency: 'MXN',
value: 943.9473666250706,
formatted: '943.95 MXN'
}
Attribute | Default | Description |
---|---|---|
currencyCode | ['AUD', 'CAD', 'CHF', 'DKK', 'EUR', 'GBP', 'HKD', 'JPY', 'MXN', 'NZD', 'PHP', 'SEK', 'SGD', 'THB', 'USD', 'ZAR'] | A list of the currencies to select. |
decimalPlaces | 2 | Number of decimal places |
decimalSeparator | "." | Character to separate decimal fractions |
thousandsSeparator | "," | Character to separate thousands |
min | 0 | The minimum value in range |
max | 500 | The maximum value in range |
Generates a random date in the range of (min, max) days. To create a date in the past the range can be negative. For instance (-60, 0) will generate a date in the last 60 days. The result is an object like this:
expiration: {
value: "Thu Jun 09 2016 14:33:26 GMT-0700 (Pacific Daylight Time)",
formatted: "Thu, Jun 09, 2016 2:33:26 PM PDT",
days: 666
}
Attribute | Default | Description |
---|---|---|
dateSeed | today = new Date() | Javascript date instance to use as the base for the computation. Defaults to today |
min | 0 | The minimum value in range, relative to dateSeed |
max | 30 | The maximum value in range, relative to dateSeed |
format | %c | Any strftime string is supported, such as "%I:%M:%S %p". strftime has several format specifiers defined by the Open group at Open group strftime PHP added a few of its own, defined at PHP strftime. You can also see the list in the YUI3 site |
%c is the preferred date and time representation for the current locale
Generates a quasi global unique identifier. It uses the YUI.guid() method and then replaces some characters with a provided prefix
Attribute | Default | Description |
---|---|---|
prefix | 'mockdata-generator' | Arbitrary string to prefix the guid. Default value is 'mockdata-generator' |
Generates a random integer in the range (min, max)
Attribute | Default | Description |
---|---|---|
min | 1 | The minimum value in range |
max | 10 | The maximum value in range |
Generates a random string using words taken from "Ipsum Lorem". It can generate any size of strings
Attribute | Default | Description |
---|---|---|
phraseMin | 6 | Minimum number of words in a phrase |
phraseMax | 10 | Maximum number of words in a phrase. Once the generator determines that a phrase is complete it will make sure that the first character is capitalized and that a punctuation mark is appended to the end of it |
paragraphNum | 1 | Number of paragraphs to generate |
wordMin | 50 | Minimum number of words in a paragraph |
wordMax | 150 | Maximum number of words in a paragraph |
Generates a random name (first last) or (last, first). It uses the list of the most popular names for men and women, as well as the most frequent last names in the United States
Attribute | Default | Description |
---|---|---|
order | fl | The order in which the name is rendered. Use "fl" for (first last) and "lf" for (last, first) |
Generates a random number in the range (min, max)
Attribute | Default | Description |
---|---|---|
min | 0 | The minimum value in range |
max | 1 | The maximum value in range |
Generates an object with the attributes described in the metadata. This is the entry point for the module, and it can be used at any level.
Attribute | Default | Description |
---|---|---|
metadata | n/a | An array of metadata descriptors for the attributes in each object |
Chooses a value out of a list. It has two modes:
Attribute | Default | Description |
---|---|---|
listName | n/a | A list to be found inside of the YUI instance object, dot notation is used to fully identify the list. For instance the name ListBase.Places will be resolved if Y.ListBase.Places exist |
list | n/a | A comma separated list of values. This attribute supersedes listName |
mode | shuffle | Either sequence of shuffle |
index | n/a | Specifies the first element in the list to be returned if the shuffle mode is specified. If not provided a random integer in the range (0, list length - 1) will be chosen |
Generates a string that follows the defined pattern
Attribute | Default | Description |
---|---|---|
pattern | n/a | A sequence of characters to be replaced when generating a value. See table below |
Character replacement rules
Character | What is generated |
---|---|
a | Generates a lower case letter (a-z) |
A | Generates an upper case letter (A-Z) |
# | Generates a digit (0-9) |
@ | Generates a letter (A-Z) and (a-z) |
\ | The next character will not be replaced. Useful to insert a, A, # or @ in the pattern |
Any other character | Will be rendered as is |
Examples (values are only representative, actual rendering is random within the category)
Pattern | Renders |
---|---|
aaa | kwt |
AAA | UYT |
@@@ | UmY |
(###) ###-#### | (716) 837-0932 \A-AAA | A-UER
Renders a string of random characters. The length of the string is a number in the range of (min, max)
Attribute | Default | Description |
---|---|---|
min | 1 | The minimum value in range |
max | 10 | The maximum value in range |
FAQs
Collection of data generators to create complex data structures and any number of records
The npm package mockdata-generator receives a total of 0 weekly downloads. As such, mockdata-generator popularity was classified as not popular.
We found that mockdata-generator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.