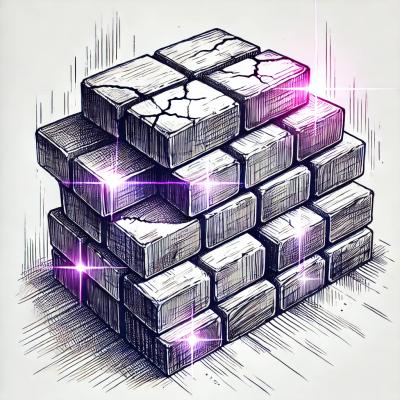
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
mocker-api
Advanced tools
Mocker API
Quick Start · Usage · Options · Delayed · Example · License
mocker-api
that creates mocks for REST APIs. It will be helpful when you try to test your application without the actual REST API server.
Features:
🔥 Built in support for hot Mocker file replacement.
🚀 Quickly and easily configure the API via JSON.
🌱 Mock API proxying made simple.
💥 Can be used independently without relying on webpack and webpack-dev-server.
mkdir mocker-app && cd mocker-app
# Create a mocker configuration file based on rules
touch api.js
# Global install dependent.
npm install mocker-api -g
# Run server
mocker ./api.js
you can put it the package.json
config as a current project dependency.
npm install mocker-api --save-dev
mocker-api
dev support mock, configured in mocker/index.js
.
you can modify the http-proxy options and add the event listeners by adding the httpProxy configuration
const proxy = {
// Priority processing.
// apiMocker(app, path, option)
// This is the option parameter setting for apiMocker
_proxy: {
proxy: {
// Turn a path string such as `/user/:name` into a regular expression.
// https://www.npmjs.com/package/path-to-regexp
'/repos/(.*)': 'https://api.github.com/',
'/:owner/:repo/raw/:ref/(.*)': 'http://127.0.0.1:2018',
'/api/repos/(.*)': 'http://127.0.0.1:3721/'
},
// rewrite target's url path. Object-keys will be used as RegExp to match paths.
// https://github.com/jaywcjlove/mocker-api/issues/62
pathRewrite: {
'^/api/repos/': '/repos/',
},
changeHost: true,
// modify the http-proxy options
httpProxy: {
options: {
ignorePath: true,
},
listeners: {
proxyReq: function (proxyReq, req, res, options) {
console.log('proxyReq');
},
},
},
},
// =====================
// The default GET request.
// https://github.com/jaywcjlove/mocker-api/pull/63
'/api/user': {
id: 1,
username: 'kenny',
sex: 6
},
'GET /api/user': {
id: 1,
username: 'kenny',
sex: 6
},
'GET /api/user/list': [
{
id: 1,
username: 'kenny',
sex: 6
}, {
id: 2,
username: 'kenny',
sex: 6
}
],
'GET /api/:owner/:repo/raw/:ref/(.*)': (req, res) => {
const { owner, repo, ref } = req.params;
// http://localhost:8081/api/admin/webpack-mock-api/raw/master/add/ddd.md
// owner => admin
// repo => webpack-mock-api
// ref => master
// req.params[0] => add/ddd.md
return res.json({
id: 1,
owner, repo, ref,
path: req.params[0]
});
},
'POST /api/login/account': (req, res) => {
const { password, username } = req.body;
if (password === '888888' && username === 'admin') {
return res.json({
status: 'ok',
code: 0,
token: "sdfsdfsdfdsf",
data: {
id: 1,
username: 'kenny',
sex: 6
}
});
} else {
return res.status(403).json({
status: 'error',
code: 403
});
}
},
'DELETE /api/user/:id': (req, res) => {
console.log('---->', req.body)
console.log('---->', req.params.id)
res.send({ status: 'ok', message: '删除成功!' });
}
}
module.exports = proxy;
proxy
=> {}
Proxy settings, Turn a path string such as /user/:name
into a regular expression.pathRewrite
=> {}
rewrite target's url path. Object-keys will be used as RegExp to match paths. #62changeHost
=> Boolean
Setting req headers host.httpProxy
=> {}
Set the listen event and configuration of http-proxybodyParserJSON
JSON body parserbodyParserText
Text body parserbodyParserRaw
Raw body parserbodyParserUrlencoded
URL-encoded form body parserbodyParserConf
=> {}
bodyParser settings. eg: bodyParserConf : {'text/plain': 'text','text/html': 'text'}
will parsed Content-Type='text/plain' and Content-Type='text/html'
with bodyParser.text
watchOptions
=> object
Options object as defined chokidar api optionsheader
=> {}
Access Control Allow options.
{
header: {
'Access-Control-Allow-Methods': 'POST, GET, OPTIONS, PUT, DELETE',
}
}
⚠️ No wildcard asterisk - use parameters instead *
(.*)
, suport v1.7.3+
You can use functional tool to enhance mock. #17
const delay = require('mocker-api/lib/delay');
const noProxy = process.env.NO_PROXY === 'true';
const proxy = {
'GET /api/user': {
id: 1,
username: 'kenny',
sex: 6
},
// ...
}
module.exports = (noProxy ? {} : delay(proxy, 1000));
apiMocker(app, mockerFilePath[, options])
apiMocker(app, Mocker[, options])
Multi entry mocker
file watching
const apiMocker = require('mocker-api');
const mockerFile = ['./mock/index.js'];
// or
// const mockerFile = './mock/index.js';
apiMocker(app, mockerFile, options)
⚠️ Not dependent on webpack and webpack-dev-server.
# Global install dependent.
npm install mocker-api -g
# Run server
mocker ./mocker/index.js
Or you can put it the package.json
config as a current project dependency.
{
"name": "base-example",
"scripts": {
+ "api": "mocker ./mocker"
},
"devDependencies": {
+ "mocker-api": "^1.6.4"
},
"license": "MIT"
}
⚠️ Not dependent on webpack and webpack-dev-server.
const express = require('express');
+ const path = require('path');
+ const apiMocker = require('mocker-api');
const app = express();
+ apiMocker(app, path.resolve('./mocker/index.js'))
app.listen(8080);
or
const express = require('express');
+ const apiMocker = require('mocker-api');
const app = express();
+ apiMocker(app, {
+ 'GET /api/user': {
+ id: 1,
+ sex: 0
+ }
+ });
app.listen(8080);
To use api mocker on your Webpack projects, simply add a setup options to your webpack-dev-server options:
Change your config file to tell the dev server where to look for files: webpack.config.js
.
const HtmlWebpackPlugin = require('html-webpack-plugin');
+ const path = require('path');
+ const apiMocker = require('mocker-api');
module.exports = {
entry: {
app: './src/index.js',
print: './src/print.js'
},
devtool: 'inline-source-map',
+ devServer: {
+ ...
+ before(app){
+ apiMocker(app, path.resolve('./mocker/index.js'), {
+ proxy: {
+ '/repos/*': 'https://api.github.com/',
+ '/:owner/:repo/raw/:ref/*': 'http://127.0.0.1:2018'
+ },
+ changeHost: true,
+ })
+ }
+ },
plugins: [
new HtmlWebpackPlugin({
title: 'Development'
})
],
output: {
filename: '[name].bundle.js',
path: require.resolve(__dirname, 'dist')
}
};
Must have a file suffix! For example: ./mocker/index.js
.
Let's add a script to easily run the dev server as well: package.json
{
"name": "development",
"version": "1.0.0",
"description": "",
"main": "webpack.config.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
+ "start": "webpack-dev-server --open",
"build": "webpack"
},
"keywords": [],
"author": "",
"license": "MIT",
"devDependencies": {
....
}
}
Mock API proxying made simple.
{
before(app){
+ apiMocker(app, path.resolve('./mocker/index.js'), {
+ proxy: {
+ '/repos/*': 'https://api.github.com/',
+ },
+ changeHost: true,
+ })
}
}
FAQs
This is dev support mock RESTful API.
The npm package mocker-api receives a total of 5,241 weekly downloads. As such, mocker-api popularity was classified as popular.
We found that mocker-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.