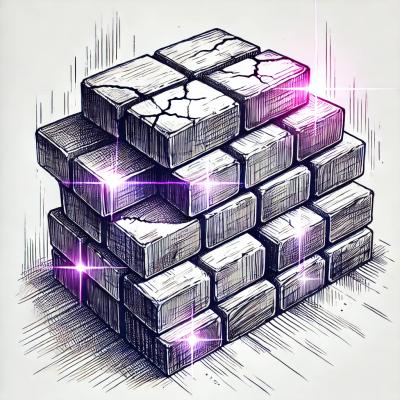
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
A tiny ODM for MongoDB with multy tenancy support.
$ npm install moltyjs --save
const { connect } = require('moltys');
// ES2015
import { connect } from ('moltyjs');
To connect to a database use the "connect()" function passing trough 'options' payload all the settings required:
const { connect } = require('moltys');
const options = {
engine: 'mongodb', // By default
uri: 'mongodb://localhost:27017/test',
max: 100, // By default
min: 1, // By default
};
const connection = connect(options);
"connect()" will return a connection instance which will allow you to perform all the actions availables on the DB.
Note: For the time being MoltyJS only support Mongo Databases.
const res = await connection.dropDatabase('test');
// true
Molty Schema are based on Mongoose Schema structure with some changes on the declaration of the inherit schema options. I even keep some field options name to make the Molty integration as easier as posible in those project are currently running Mongoose.
To create a new Schema use the "Schema()" constructor passing the schema and the options:
const { Schema } = require('moltys');
const newSchema = Schema(
{
email: {
type: String,
required: true,
unique: true,
maxlength: 100,
},
password: {
type: Number,
required: true,
},
name: {
type: String,
default: '',
},
age: {
type: Number,
},
},
{
timestamps: true,
inheritOptions: {
discriminatorKey: '__kind',
},
},
);
The schema field properties alowed are:
And the schema options allowed are:
Once we have created our schema we need to register as a model so we can start to create, find, updete and delete documents. To do this you must provide the a proper schema and a model name. The model name will be the collection name on the DB so use the criteria you want since Molty does not make any accomodation on them like auto plurilize.
const { Model } = require('moltys');
const TestModel = new Model(newSchema, 'TestModel');
You can also create models which inherits from other models and you can decide in which fashion you wan to do it. You have to make sure that the discriminator key of the child models are the same than the parents and also set what you want to merge from the parent model.
const { Schema } = require('moltys');
const newSchemaDiscriminator = Schema(
{
job: {
type: String,
default: '',
},
position: {
type: String,
},
},
{
timestamps: true,
inheritOptions: {
discriminatorKey: '__kind',
merge: ['methods', 'preHooks', 'postHooks'],
},
},
);
TestModelDiscriminator = TestModel.discriminator(
newSchemaDiscriminator,
'TestModelDiscriminator',
);
The merge option must be an array with the element you want to merge from the parent model, teh options are:
Once we have already set up the Schema and registered the Model with it we can start creating document from that Model as follow:
const { Model } = require('moltys');
const TestModel = new Model(newSchema, 'TestModel');
newDoc = TestModel.new({
email: 'test@moltyjs.com',
password: '1321321',
name: 'Mario Lemes',
});
Document middleware is supported for the following document functions.
In document middleware functions, this refers to the document.
Query middleware is supported for the following Model and Query functions.
In query middleware functions, this refers to the query.
MoltyJS support referencing document that later on you wil be able to populate on find queries. To make a refference to another Model just add a new field on the Schema with the type ObjectId and the "ref" propertie with the Model name referenciated.
const { Schema } = require('moltys');
const referenceSchema = new Schema(
{
email: {
type: String,
required: true,
unique: true,
maxlength: 100,
},
password: {
type: String,
required: true,
unique: true,
maxlength: 150,
default: () => crypto.randomBytes(20).toString('hex'),
},
accountExpiration: {
type: Date,
default: () =>
moment()
.add(24, 'hours')
.utc()
.format(),
},
status: {
type: String,
required: true,
enum: ['pending', 'accepted', 'error'],
default: 'pending',
},
model: {
type: Schema.types().ObjectId,
ref: 'TestModel',
},
},
{
timestamps: true,
},
);
You can use an array of ObjectId also as type ([ObjectId]). Noticed that to get the proper ObjectId type you must tu get it from the Schema.types() method object is returned.
There are several operations to save a document into the database:
insertOne(tenant, doc)
const res = await connection.insertOne('tenant_test', newDoc);
// Document || Error
There are several operations to recover a document from the database:
findOne(tenant, collection, query = {}, options = {})
const resFind = await connection.findOne('tenant_test', 'TestModel', {
name: 'Mario Lemes',
});
// Document || Error
There are several operations to recover a document from the database:
updateOne(tenant, collection, filter, payload, options = {})
const resUpdate = await connection.updateOne(
'tenant_test',
'TestModel',
{ name: 'Mario Lemes' },
{
$set: {
name: 'Some other name',
},
},
);
// Document || Error
Updating a document support all the update operators from MongoDB
[0.1.5] - 2017-12-17
FAQs
A tiny ODM for MongoDB with multy tenancy support.
The npm package moltyjs receives a total of 6 weekly downloads. As such, moltyjs popularity was classified as not popular.
We found that moltyjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.