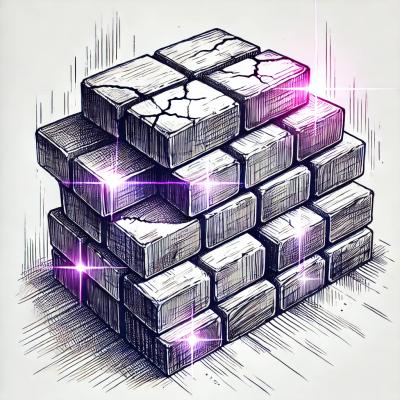
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
MongoT is a modern ODM library for MongoDb.
Just type npm i -S mongot
to install this package.
You may need TypeScript 2+ and should enable experimentalDecorators
,
emitDecoratorMetadata
in your tsconfig.json
.
A collection is a class which support CRUD operations for own documents.
Let's create a simple collection and name it as UserCollection
:
# UserCollection.ts
import {Collection, collection} from 'mongot';
import {UserDocument} from './UserDocument';
@index('login', {unique: true})
@collection('users', UserDocument)
class UserCollection extends Collection<UserDocument> {
findByEmail(email: string) {
return this.findOne({email});
}
}
Any collections should refer to their own document schemas so
we link the class UserCollection
to a users
collection in
database and a UserDocument
schema by a @collection
decorator.
A document class describes a schema (document properties, getters/setters, hooks for insertions/updates and helper functions).
Schema supports these types: ObjectID
, string
, boolean
, number
,
date
, Object
, SchemaFragment
(also known as sub-document)
and array
. A buffer
type doesn't tested at this time.
let's look at a UserDocument
schema:
# UserDocument.ts
import {SchemaDocument, SchemaFragment, Events} from 'mongot';
import {hook, prop, document} from 'mongot';
import * as crypto from 'crypto';
@fragment
class UserContactsFragment extends SchemaFragment {
type: 'phone' | 'email' | 'im';
title: string;
value: string;
}
@document
class UserDocument extends SchemaDocument {
@prop
public email: string;
@prop
public password: string;
@prop
public firstName: string;
@prop
public lastName: string;
@prop
registered: Date = new Date();
@prop
updated: Date;
@prop(UserContactsFragment)
children: SchemaFragmentArray<UserContactsFragment>;
@hook(Events.beforeUpdate)
refreshUpdated() {
this.updated = new Date();
}
get displayName() {
return [this.firstName, this.lastName]
.filter(x => !!x)
.join(' ') || 'Unknown';
}
checkPassword(password: string) {
return this.password === crypto.createHash('sha1')
.update(password)
.digest('hex');
}
}
To connect collections to a MongoDb instance you should create a repository:
# index.ts
import {Repository} from 'mongot';
const options = {};
const repository = new Repository('mongodb://localhost/test', options);
The Repository
class constructor has same arguments that
MongoClient.
Before querying you should get a connected collection:
# index.ts
import {Repository} from 'mongot';
import {UserCollection} from './UserCollection';
const options = {};
const repository = new Repository('mongodb://localhost/test', options);
async function main(): void {
const users: UserCollection = repository.get(UserCollection);
const user = await users.findByEmail('username@example.com');
// do something with user
...
}
You can use projection and aggregation with partial schemas:
# partial.ts
import {PartialDocumentFragment, prop} from 'mongot';
import {UserCollection} from './UserCollection';
// initialize repo ...
@fragment
class PartialUser extends PartialDocumentFragment {
@prop email: strng;
@prop created: Date;
}
(async function() {
const Users = repository.get(UserCollection);
const partialUser = await (await Users.find())
.map(doc => PartialUser.factory(doc))
.project<PartialUser>({email, created})
.fetch();
console.log(partialUser instanceof PartialDocumentFragment); // true
)();
MIT
FAQs
MongoT is a modern ODM library for MongoDb.
We found that mongot demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.