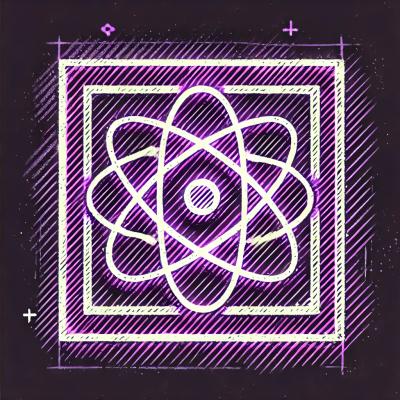
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Morse code translator, Morse encoder and decoder which can also generate audio.
Morsify is a Morse code encoder and decoder with no dependencies. Currently, it supports Latin, Cyrillic, Greek, Hebrew, Arabic, Persian, Japanese, Korean, and Thai, with audio-generation functionality using the Web Audio API.
$ npm install morsify --save
$ yarn add morsify
const morsify = require('morsify');
const encoded = morsify.encode('SOS'); // ... --- ...
const decoded = morsify.decode('... --- ...'); // SOS
const characters = morsify.characters(); // {'1': {'A': '.-', ...}, ..., '11': {'ㄱ': '.-..', ...}}
const audio = morsify.audio('SOS');
audio.play(); // play audio
audio.stop(); // stop audio
Alternatively, you can use the library directly with including the source file.
<script src="https://rawgit.com/ozdemirburak/morsify/master/dist/morsify.min.js"></script>
<script>
var encoded = morsify.encode('SOS'); // ... --- ...
var decoded = morsify.decode('... --- ...'); // SOS
var characters = morsify.characters(); // {'1': {'A': '.-', ...}, ..., '11': {'ㄱ': '.-..', ...}}
var audio = morsify.audio('SOS');
var oscillator = audio.oscillator; // OscillatorNode
var context = audio.context; // AudioContext
var gainNode = audio.gainNode; // GainNode
audio.play(); // play audio
audio.stop(); // stop audio
</script>
You can customize the dash, dot, or space characters and specify the alphabet with the priority option for an accurate encoding and decoding.
The priority option gives direction to the plugin to start searching for the given character set first.
Set the priority option according to the list below.
const cyrillic = morsify.encode('Ленинград', { priority: 5 }); // .-.. . -. .. -. --. .-. .- -..
const greek = morsify.decode('... .- --. .- .--. .--', { priority: 6 }); // ΣΑΓΑΠΩ
const hebrew = morsify.decode('.. ––– . –––', { dash: '–', dot: '.', priority: 7 }); // יהוה
const japanese = morsify.encode('NEWS', { priority: 10, dash: '-', dot: '・', separator: ' ' }); // -・ ・ ・-- ・・・
const characters = morsify.characters({ dash: '–', dot: '•' }); // {'1': {'A': '•–', ...}, ..., '11': {'ㄱ': '•–••', ...}}
const arabicAudio = morsify.audio('البراق', { // generates the Morse .- .-.. -... .-. .- --.- then generates the audio from it
unit: 0.1, // period of one unit, in seconds, 1.2 / c where c is speed of transmission, in words per minute
fwUnit: 0.1, // period of one Farnsworth unit to control intercharacter and interword gaps
oscillator: {
type: 'sine', // sine, square, sawtooth, triangle
frequency: 500, // value in hertz
onended: function () { // event that fires when the tone stops playing
console.log('ended');
}
}
});
const oscillator = arabicAudio.oscillator; // OscillatorNode
const context = arabicAudio.context; // AudioContext;
const gainNode = arabicAudio.gainNode; // GainNode
arabicAudio.play(); // will start playing Morse audio
arabicAudio.stop(); // will stop playing Morse audio
Contributions are welcome.
Install node and npm and run the commands below.
$ npm install --global gulp-cli
$ npm install
$ gulp
The MIT License (MIT). Please see License File for more information.
FAQs
Morse code translator, Morse encoder and decoder which can also generate audio.
The npm package morsify receives a total of 1 weekly downloads. As such, morsify popularity was classified as not popular.
We found that morsify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.