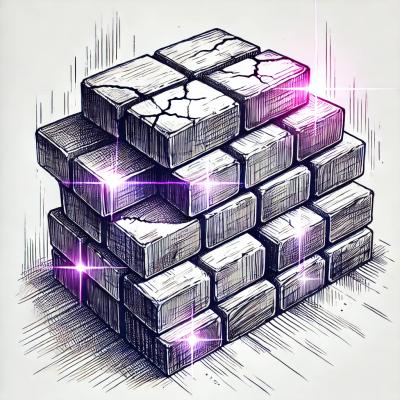
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
JavaScript library that aims to allow the developer to easily build web applications.
Test MotaJS now with Tonic! (no installation needed)
MotaJS is a JavaScript library that includes a lot of different things to help you build web applications easily from scratch. The idea is to include API's higher than the ones provided by the browser but lower enough so that you can build on top of them if you like. I'm open to other ideas so, if you want to contribute with code or documentation, see the Contribute section below. Thanks.
Includes a lot of useful functions: assign, keys, isEqual, isObject, etc...
var object1 = {
apple: 0,
banana: { weight: 52, price: 100 },
cherry: 97
};
var object2 = {
banana: { price: 200 },
durian: 100
};
mota.assign( object1, object2 );
// { apple: 0, banana: { price: 200 }, cherry: 97, durian: 100 }
A module that can be mixed in to any object, giving the object the ability to bind and trigger custom named events.
var object = {};
mota.assign( object, mota.Events );
object.on( "expand", function() {
alert( "expanded!" );
} );
object.trigger( "expand" );
It supports regular expressions!
obj.on( /a/, function( data, ...args ) {
data.string; // "ab"
data.match; // [ "a" ]
} );
obj.trigger( "ab", ...args );
The Map object is a simple key/value map. Any value (both objects and primitive values) may be used as either a key or a value.
Implemented by a hash-array mapped trie.
You can create mutable maps...
var map1 = mota.Map( { a: 1, b: 2, c: 3 } );
var map2 = map1.set( "b", 50 );
map1.get( "b" ); // 50
map2.get( "b" ); // 50
map1 === map2 // true
... but you can also create immutable ones.
var map1 = mota.ImmutableMap( { a: 1, b: 2, c: 3 } );
var map2 = map1.set( "b", 2 );
map1 === map2 // no change
var map3 = map1.set( "b", 50 );
map1 !== map3 // change
map1.get( "b" ); // 2
map3.get( "b" ); // 50
If you use it as mutable data, you can observe changes:
var map1 = mota.Map( { a: 1, b: 2, c: 3 } );
map1.on( "change", function( changes ) {
// changes: list of changes
} );
Allows you to resolve and normalize pathnames.
An implementation of the Observable proposal + some other features.
A complete Promise polyfill.
It also emits unhandledRejection
/rejectionHandled
events on NodeJS and unhandledrejection
/rejectionhandled
events on the Browser.
Simple express-style router. Works in NodeJS and the browser.
var router = new mota.Router();
router.get( "/:foo", function( req, res, next ) {
next();
} );
router.handle( { url: "/something", method: "GET" }, {}, function() {} );
Helps you use the History API in the browser.
var router = new mota.Router();
router.get( "/page", function( request, response, next ) {
next();
} );
var history = new mota.History( router );
history.navigate( "/page" );
...
var TodoApp = mota.view.createClass( {
...
render: function( dom ) {
var items = this.map.get( "items" );
dom.setType( "div" );
dom.open( "h3" );
dom.text( "TODO" );
dom.close();
dom.void( TodoList, null, { items: items } );
dom.open( "form" );
dom.attr( "onSubmit", this.handleSubmit );
dom.open( "input" );
dom.attr( "onInput", this.onInput );
dom.attr( "value", this.map.get( "text" ) );
dom.close();
dom.open( "button" );
dom.text( "Add #" + ( items.length + 1 ) );
dom.close();
dom.close();
}
} );
mota.view.render( function( dom ) {
dom.void( TodoApp );
}, document.body );
Complete example here.
List of all releases and changelog
<script src="mota.min.js"></script>
MotaJS is also available through jsDelivr CDN and cdnjs.
npm install motajs
All the information about how to use MotaJS is available here.
If you want to see documentation of the latest or previous versions, just select a different tag instead of master
.
The project is hosted on GitHub. You can report bugs and discuss features on the issues page.
How to build and test your copy of MotaJS
FAQs
JavaScript library that aims to allow the developer to easily build web applications.
The npm package motajs receives a total of 13 weekly downloads. As such, motajs popularity was classified as not popular.
We found that motajs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.