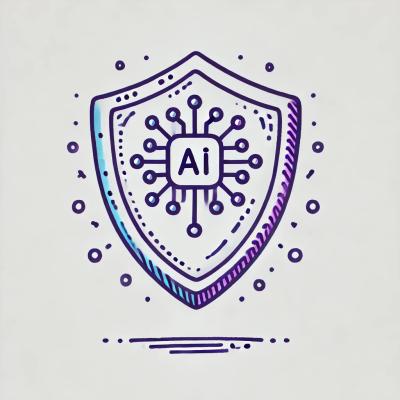
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
movie-metadata
Advanced tools
A simple utility to easily fetch movie metadata, given an Array of movie titles, using the API from the Open Movie Database.
A simple utility to easily fetch movie metadata from a list of movie titles using the API from the Open Movie Database.
movie-metadata
will take an Array, List or JSON file filled with movie titles, and search the Open Movie Database (omdb) API for their respective metadata (Title, Rating, Writers, Actors, Plot, Runtime, etc.) and output the metadata into a JSON file (CLI) or return an Object with the same information.
movie-metadata
is available via npm.
It can be installed either locally (for programmatic implementation) or globally (for general usage)
Local Installation:
npm install movie-metadata --save
Global Installation:
npm install -g movie-metadata --save
This is how you can use movie-metadata
.
How to use movie-metadata
with the Command-Line Interface (CLI).
Note: Assuming that
movie-metadata
is globally installed
Example
$ getmetadata --key YOUR_API_KEY /Users/me/moviesList.json
This will create a moviesList-metadata.json
and moviesList-notFound.json
file in the /Users/me/
directory.
Note: The
moviesList-notFound.json
file is only created if some movies in the list were not found on the omdb API server.
The moviesList-metadata.json
file will contain an Array of Objects containing each movies respective metadata fetched from omdb's API server omdbapi.com.
How to use movie-metadata
from within a .js
file
Note: Assuming that
movie-metadata
is locally installed
Example: Async/Await
const { getMetadata } = require('movie-metadata')
async function getIt() {
const metadata = await getMetadata({
key: 'YOUR_API_KEY',
source: ['dead man\'s chest', 'at world\'s end', 'ralph breaks the internet', 'Ocean\'s Eight']
})
console.log(metadata)
}
getIt()
// Outputs
{ fetchedMetadata: [
{
Title: 'Pirates of the Caribbean: Dead Man\'s Chest',
Year: '2006',
Rated: 'PG-13',
Released: '07 Jul 2006',
Runtime: '151 min',
Genre: 'Action, Adventure, Fantasy',
Director: 'Gore Verbinski',
Writer: 'Ted Elliott, Terry Rossio, Ted Elliott (characters), Terry Rossio (characters), Stuart Beattie (characters), Jay Wolpert...'
Actors: 'Johnny Depp, Orlando Bloom, Keira Knightley, Jack Davenport',
Plot: 'Jack Sparrow races to recover the heart of Davy Jones to avoid enslaving his soul to Jones\' service, as other friends a...'
Language: 'English, Turkish, Greek, Mandarin, French',
Country: 'USA',
Awards: 'Won 1 Oscar. Another 42 wins & 53 nominations.',
Poster: 'https://m.media-amazon.com/images/M/MV5BMTcwODc1MTMxM15BMl5BanBnXkFtZTYwMDg1NzY3._V1_SX300.jpg',
Ratings: [Array],
Metascore: '53',
imdbRating: '7.3',
imdbVotes: '597,591',
imdbID: 'tt0383574',
Type: 'movie',
DVD: '05 Dec 2006',
BoxOffice: '$423,032,628',
Production: 'Buena Vista',
Website: 'http://pirates.movies.com',
Response: 'True'
},
...
],
notFoundMovies: [] }
Example: Promises
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: ['dead man\'s chest', 'at world\'s end', 'ralph breaks the internet', 'Ocean\'s Eight']
}).then(metadata => {
console.log(metadata)
})
// Same output
Below is a reference to all of movie-metadata
's API properties.
This is the API when movie-metadata
is installed globally, and is used within the CLI.
--key|-k
omdb API key
none
String
Example
$ getmetadata -k YOUR_API_KEY
// or
$ getmetadata --key YOUR_API_KEY
--source|-s
Source JSON file of movie titles to search with
none
String / JSON File Path
Example
$ getmetadata -k YOUR_API_KEY -s /path/to/movies/list.json
// or
$ getmetadata -k YOUR_API_KEY --source /path/to/movies/list.json
--progress|-p
Whether or not to show a CLI progress bar while downloading the metadata
true
Boolean
Example
$ getmetadata -k YOUR_API_KEY -s /movies/list.json -p false
// or
$ getmetadata -k YOUR_API_KEY -s /movies/list.json --progress false
--verbose|-v
Whether or not to run verbosely
Note: This disables
--progress
parameter, if enabled
false
Boolean
Example
$ getmetadata -k YOUR_API_KEY -s /movies/list.json -v
// or
$ getmetadata -k YOUR_API_KEY -s /movies/list.json --verbose
--dest|-d
Where to save the JSON file with metadata
Same path as source with '-metadata' appended
String
Example
$ getmetadata -k YOUR_API_KEY -s /movies/list.json -d /movies/metadata.json
// or
$ getmetadata -k YOUR_API_KEY -s /movies/list.json --dest /movies/metadata.json
--notFound|-n
Where to save the JSON file that holds the movies that were not found on the omdb API server
Note: the
notFound
file will only be create if there was any movies that were not found on the omdb API server.
Same path as source with '-notFound' appended
String
Example
$ getmetadata -k YOUR_API_KEY -s /movies/list.json -n /movies/metadata.json
// or
$ getmetadata -k YOUR_API_KEY -s /movies/list.json --notFound /movies/notFound.json
--overwrite|-o
Whether or not to overwrite the source file with the metadata JSON data
Note: If enabled, this disables
dest
parameter.
false
Boolean
Example
$ getmetadata -k YOUR_API_KEY -s /movies/list.json -o
// or
$ getmetadata -k YOUR_API_KEY -s /movies/list.json --overwrite
This is the API when movie-metadata
is installed locally, and is used within a .js
file.
key
omdb API key
none
String
Example
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: 'path/to/list/of/movies.json'
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
source
Path to JSON file, Array, or list of movie titles to fetch metadata for
none
String|Array|JSON file path
Note: If a string is provided and it is not a path to a JSON file, then the "splitter" parameter is used to
split
theString
into anArray
ofStrings
Example: JSON file
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: 'path/to/list/of/movies.json'
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
// movies.json file format
[
"Ocean's Eight",
"Ralph Breaks the Internet"
// ...
]
Example: Array (inline)
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: ["Ocean's Eight", "Ralph Breaks the Internet", "Pulp Fiction"]
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
progress
Whether or not to show a CLI progress bar while downloading the metadata
true
Boolean
Example
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: 'path/to/list/of/movies.json',
progress: true
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
This will display a CLI progress bar using the cli-progress
module.
verbose
Whether or not to run verbosely
Note: This disables
progress
parameter, if enabled
false
Boolean
Example
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: 'path/to/list/of/movies.json',
verbose: true
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
This will output each movie title to the console, along with whether or not it was found.
dest
Where to save the fetched Array of movie metadata
Note: If set to false, the fetched movie metadata will be returned as a Promise of an Object with two properties
fetchedMetadata
:[Object]
andnotFoundMovies
:[String]
.
Same path as source with '-metadata' appended
String|Boolean
Example
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: 'path/to/list/of/movies.json',
dest: false
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
notFound
Where to save the JSON file that holds the movies that were not found on the omdb API server
Note: If set to false, the fetched movie metadata will be returned as a Promise of an Object with two properties
fetchedMetadata
:[Object]
andnotFoundMovies
:[String]
.
Same path as source with '-notFound' appended
String|Boolean
Example
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: 'path/to/list/of/movies.json',
notFound: false
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
overwrite
Whether or not to overwrite the source file with the metadata JSON data
Note: If enabled, this disables
dest
parameter. This is automatically disabled ifdest
parameter is disabled (false
)
false
Boolean
Example
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: 'path/to/list/of/movies.json',
overwrite: true
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
splitter
(Not Yet Working)What character to use to split the source
String
into an Array
Note: This is only applied if the
source
parameter is not a JSON file, or is a plainString
.
Note: As of version
1.0.3
this feature is not completely working yet. If thesource
parameter is a file, it must be aJSON
file
\n
String
Example
const { getMetadata } = require('movie-metadata')
getMetadata({
key: 'YOUR_API_KEY',
source: "Lucy::Se7en::Dead Man's Chest::Ocean's Eleven",
splitter: '::'
}).then(metadata => {
// Do stuff with the Array of movies (with metadata)
})
We use SemVer for versioning.
This project is licensed under the CC BY-NC 4.0 License - see the LICENSE file for details.
movie-metadata
is not affiliated or endorsed by omdbapi.com in any way. An API Key (free) must be obtained prior to using this module.
movie-metadata
FAQs
A simple utility to easily fetch movie metadata, given an Array of movie titles, using the API from the Open Movie Database.
The npm package movie-metadata receives a total of 0 weekly downloads. As such, movie-metadata popularity was classified as not popular.
We found that movie-metadata demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.