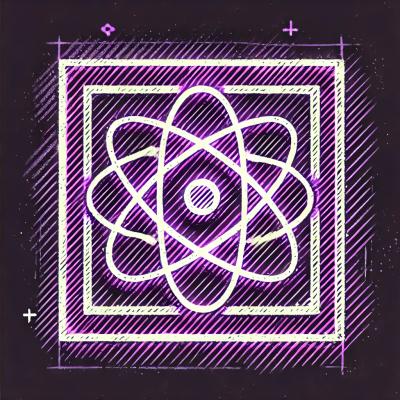
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
msw-validate
Advanced tools
Mock Service Worker allows you to intercept requests and return mock responses from handlers. It works great for unit tests where mock handlers can be registered to verify application behavior for different responses.
But testing network calls should not be limited to just mocking responses. Validating that a particular endpoint was called with the correct params, headers, query, cookie or body is a must for a good unit test.
msw-validate allows you to declaratively define handlers that perform request validations, and also to easily setup per test scenarios. This library follows the msw best practice of asserting request validity by returning a error response when validation fails.
Define a handler and declare request assertions in the validate
block
import { defineHttpHandler } from "msw-validate";
const handler = defineHttpHandler({
method: "GET",
url: "customer/:id/orders",
validate: {
"params.id": "1234",
"query.status": ["ordered", "shipped"],
},
return: {
orders: [{ id: 1, status: "shipped" }]
}
});
Write the test
import { setupServer } from "msw/node";
import { orderService } from "services/order";
const server = setupServer();
beforeAll(() => server.listen());
afterEach(() => server.resetHandlers());
afterAll(() => server.close());
describe("order service", () => {
it("should fetch current orders", async () => {
// register handler
server.use(handler())
const res = await orderService.getCurrentOrders("1234");
const data = await res.json()
expect(data.orders.length).toBe(1)
})
})
If the order service incorrectly sends a ?status=canceled
to the service, a 400 response will be returned with following details.
{
path: "status",
value: "canceled",
error: 'expected ["ordered", "shipped"]'
}
More often than not, tests that verify requests or responses end up simply asserting that the data equals some deeply nested json (like a saved response from an actual call to an endpoint)
import expected from "./mocks/create-order.json";
expect(request.body).toEqual(expected);
Though these work great as regression tests, it is recommended to declare important expectations in the validate block.
Adding a few assertions automaticaly surfaces the actual intent of the code as opposed to a blob of data.
defineHttpHandler({
validate: {
"params.id": "1234",
"cookies.promo": "BF2024",
"query.status": ["ordered", "shipped"],
"query.limit": (limit) => (limit > 0 && limit < 50) || "invalid limit",
"headers.authorization": (auth) => auth === "Bearer secret" || HttpResponse.text(null, 403)
"body.orders[1].description": "second order",
"body": (data) => _.isEqual(data, jsonBlob)
},
return: { ... }
});
The Validation Object
query.limit
aboveheaders.authorization
aboverequestBodyType: "form-data"
--forceExit
to jestOn success, the data or HttpResponse specified as return value is sent as a json response
Custom response functions can be specified and take the same argument as a msw resolver plus an invocation count.
This allows the same handler to return different responses for successive calls.
See below example from response.test.ts
const handler = defineHttpHandler({
method: "GET",
url: buildUrl("/test/:id"),
validate: {},
return: ({ params, invocation }) => {
const response = { id: params.id, invocation };
return invocation == 1 ? response : HttpResponse.json(response);
},
});
FAQs
Mock Service Worker Request Validation
We found that msw-validate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.