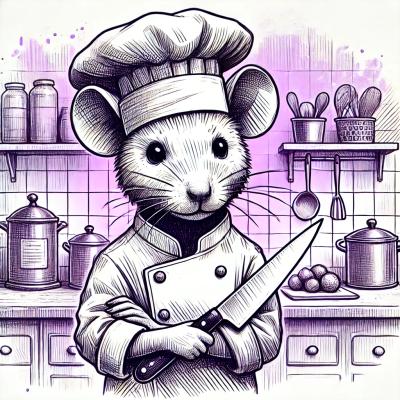
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Method, URL, Headers and Body
Install with: npm i muhb
.
Getting NodeJS homepage:
const muhb = require('muhb');
var { status, headers, body } = await muhb('get', 'https://nodejs.org/en/');
MUHB exposes a short signature for all HTTP verbs:
method ( String url [, Object headers] [, String body] )
Posting a random form:
const { post } = require('muhb');
var { status, headers, body } = await post('https://nodejs.org/en/', 'key=value&key=value');
Sending headers:
const { put } = require('muhb');
var { status, headers, body } = await put(
'https://nodejs.org/en/',
{ myHeader: 'example' },
'key=value&key=value'
);
If you would like MUHB to not generate automatic content and date headers, send a ghost parameter like this:
const { put } = require('muhb');
var { status, headers, body } = await put(
'https://nodejs.org/en/',
{ 'no-auto': true, myHeader: 'example' },
'key=value&key=value'
);
Having all available muhb methods:
const muhb = require('muhb');
muhb.get //=> [function]
muhb.post //=> [function]
muhb.patch //=> [function]
muhb.del //=> [function]
muhb.put //=> [function]
muhb.head //=> [function]
muhb.options //=> [function]
If you need to access the nodejs res
object, all muhb methods return it modified
to have our status
and body
keys.
const { get } = require('muhb');
let res = await get('https://nodejs.org/en/');
Testing response data:
var { assert } = await get('https://example.com');
// Assert about your reposnse body.
assert.body.exactly('foobar');
assert.body.contains('oba');
assert.body.match(/oo.a/);
// Mostly chainable.
assert.body.type('application/json').length(23);
// Test JSON bodies.
assert.body.json
.hasKey('foo')
.match('foo', 'bar')
.empty(); // test for {}
// Test JSON array.
assert.body.json.array
.match(1, 'bar')
.includes('foo')
.length(2)
.empty();
// Assert about response status code
assert.status.is(200);
assert.status.not(400);
assert.status.in([ 200, 203, 404 ]);
assert.status.notIn([ 500, 403, 201 ]);
assert.status.type(2); // Test for 2xx
assert.status.notType(5) // Test for NOT 5xx
// Assert about response headers
assert.headers
.has('authorization')
.match('connection', 'close');
As of now the only auth methods supported are Basic and MD5 Digest.
You must ensure your server responds with 401
and a WWW-Authenticate
header
so muhb knows to perform the auth.
Then just send your credentials in the headers object as follows:
let { body } = await post('http://example.com', {
auth: { username: 'my-user', password: 'my-pass' }
});
Or use the user and password syntax (they will be stripped from the URL before being sent).
let { body } = await post('http://my-user:my-pass@example.com');
Define a pool with a max size of 10 requests and a timeout of 2 seconds:
const { Pool } = require('muhb');
let myPool = new Pool({ size: 10, timeout: 2000 });
Then run the pool over an array of say request bodies:
let bodies = 'abcdefghijklmnopqrstuvwxyz'.split('');
bodies.forEach( body => myPool.post('http://localhost/fail', body) );
Wait until all requests recive responses.
// With promises
let responses = await myPool.done();
// With events
myPool.on('finish', responses => console.log(responses));
Wait for a single request in the pool to finish.
let res = await myPool.post('http://localhost/fail');
Act on every request that is responded.
myPool.on('response', (req, res) => {
console.log(res.status);
});
[v3.2.1] - 2023-10-23
FAQs
A set of functions for coding easy to read HTTP requests.
The npm package muhb receives a total of 571 weekly downloads. As such, muhb popularity was classified as not popular.
We found that muhb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.