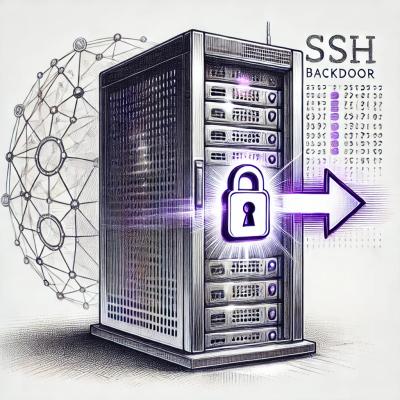
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
import {
map,
filter,
...
} from 'musejs';
map
Higher order map
map([1, 2, 3, 4], i => i + 1)
// => [2, 3, 4, 5]
filter
Higher order filter
filter([1, 'hey', '3', 4], !isNaN);
// => [1, 3, 4]
reduce
Higher order reduce
reduce([1, 2, 3, 4], (i, j) => i + j);
// => 10
foreach
Higher order forEach
forEach([1, 2, 3, 4], i => console.log (i))
// => 1
// => 2
// => 3
// => 4
reverse
Higher order reverse
reverse([1, 2, 3])
// => [3, 2, 1]
id
Return true if the value is not 0, undefined or null
filter([1, null, 4, undefined, 'Hello'], id)
// => [1, 4, 'Hello']
complement
Negates a function
let isNumber = complement(isNaN);
isNumber(1)
// => true
range
Yields a range from min
to max
by step
[...range(0, 10)]
// => [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
times
Performs an action n times
times(3, () => console.log ('Hello'))
// => Hello
// => Hello
// => Hello
flatten
Flatten an array
flatten([1, 2, 3, [4, [5, [6, [7, [8, 9]]]]]])
// => [1, 2, 3, 4, 5, 6, 7, 8, 9]
partial
Take a function fn and an array of args with empty spaces
and returns a partial function g
that take a single arg
let rgb = (r, g, b) => [r, g, b];
let varyGreen = partial(rgb, 4, null, 5);
varyGreen(4)
// => rgb(4, 4, 5);
thread
Pass a value to a list of function, one function at a time
thread(
1,
i => i + 1,
i => i - 2
)
// => -1
compose
Return a function that takes a value to be threaded in a list of functions
add3AndMultiply3 = compose(add3, multiply3);
add3AndMultiply3(3);
// => 18
promisify
Transform a callback style function into a Promise style function
let readfile = promisify(fs.readFile)
let text = readfile('./test.txt')
text.then(console.log.bind(console))
isNumber
, isArray
, isString
, isObject
Primitives type checking functions
isNumber('3')
// => true
isString('Hello')
// => true
isArray({name: guillaume})
// => false
dotProduct
Dot product between 2 vectors
dotProduct([1, 2, 3], [4, 5, 6]);
// => 32
addMatrix
Matrix/vector addition
addMatrix(
[[1, 1, 1, 1],
[1, 1, 1, 1]],
[[1, 1, 1, 1],
[1, 1, 1, 1]]
)
// => [[2, 2, 2, 2], [2, 2, 2, 2]]
getRow
Returns a matrix row as a vector
getRow(
[[1, 1, 1, 1, 1],
[2, 2, 2, 2, 2],
[1, 1, 1, 1, 1],
[1, 1, 1, 1, 1]],
1
)
// => [2, 2, 2, 2, 2]
getColumn
Returns a matrix column as a vector
getColumn(
[[2, 1, 1, 1, 1],
[2, 1, 1, 1, 1],
[2, 1, 1, 1, 1],
[2, 1, 1, 1, 1]],
0
)
// => [2, 2, 2, 2, 2]
multMatrix
Performs a matricex multiplication
const m1 = [
[1, 2, 3],
[4, 5, 6]
];
const m2 = [
[7, 8],
[9 , 10],
[11, 12]
]
multMatrix(m1, m2)
// => [ [58, 64],
// [139, 154]]
matrixDimensions
Returns a matrix dimensions [rows, columns]
const [rows, columns] = matrixDimensions(myMatrix);
transpose
Transpose a matrix
console.log (transpose([
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]))
// => [ [ 1, 4, 7 ],
// [ 2, 5, 8 ],
// [ 3, 6, 9 ] ]
FAQs

The npm package musejs receives a total of 0 weekly downloads. As such, musejs popularity was classified as not popular.
We found that musejs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.