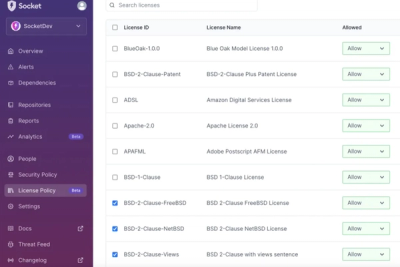
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
nano
(short for NanoCouch) is a minimalistic couchdb
driver for node.js
.
npm install nano
A quick example on using nano
.
In nano
callback always return three arguments:
err: The error, if any. Check error.js for more info.
headers: The HTTP response headers from CouchDB, if no error.
response: The HTTP response body from CouchDB, if no error.
Because in nano
you can do database operations you are not bound to one and only one database. The first thing you do is load the module pointing either providing a JSON configuration object or a string that represents the relative file path of that config, e.g. cfg/tests.js
. Do refer to cfg/couch.example.js for a example. There you can use it to specify where couchdb
lives, if you want to use https
, or even to use a proxy
.
var nano = require('nano')('./cfg/tests.js');
Now you can do your database operations using nano
. These include things like create, delete or list databases. Let's create a database to store some documents:
nano.db.create("alice");
Where is my callback? Well in nano
you have the option of not having a callback and say "I don't care".
Of course now you want to insert some documents and you wish you had the callback, so let's add it:
// Clean up the database we created previously
nano.db.destroy("alice", function(err,headers,response) {
nano.db.create("alice", function(){
// Specify the database we are going to use
var alicedb = nano.use("alice");
alicedb.insert("rabbit", {crazy: true}, function(e,h,r){
if(e) { throw e; }
console.log("You have inserted the rabbit.")
});
});
});
The alicedb.use
method creates a scope
where you operate inside a single database. This is just a convenience so you don't have to specify the database name every single time you do an update or delete.
Don't forget to delete the database you created:
nano.db.destroy("alice");
Assuming var db = nano.use("somedb");
:
db.insert(doc_name,doc,callback) // doc_name is optional
db.update(doc_name,rev,doc,callback)
db.destroy(doc_name,rev,callback)
db.get(doc_name,callback)
db.list(callback)
nano.db.create(db_name,callback)
nano.db.destroy(db_name,callback)
nano.db.get(db_name,callback)
nano.db.list(callback)
nano.db.compact(db_name,callback)
nano.db.replicate(source,target,continuous,callback) // continuous is optional
nano.use(db_name)
nano.request(opts,callback)
You can use nano.scope
instead of nano.use
. They are both also available inside db, e.g. nano.db.scope
.
When using a database with nano.use
you can still replicate
, compact
, and list
the database you are using. e.g. to list you can use db.info
(because db.list
is for listing documents). Other methods remain the same, e.g. db.replicate
.
Some future plans are mostly:
pipe
, support as provided by request_changes
feed_uuids
, _stats
, _config
, _active_tasks
, _all_docs_by_seq
batch
in updates and inserts.Great segway to contribute.
Everyone is welcome to contribute.
nano
in githubgit checkout -b my_branch
git push origin my_branch
To run the tests simply browse to test/
and ./run
. Don't forget to install the packages referred as dev dependencies in package.json
.
Always make sure all the tests pass before sending in your pull request! OR I'll tell Santa!
_
/ _) ROAR! I'm a vegan!
.-^^^-/ /
__/ /
/__.|_|-|_|
git clone git://github.com/dscape/nano.git
(oO)--',-
in caos
FAQs
The official CouchDB client for Node.js
The npm package nano receives a total of 27,477 weekly downloads. As such, nano popularity was classified as popular.
We found that nano demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new license scanner with an improved suite of features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.