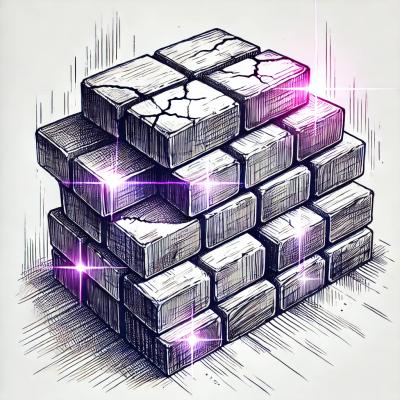
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
nativescript-bottom-navigation
Advanced tools
Nativescript plugin to add a bottom navigation component for Android & iOS
Nativescript plugin for Android & iOS to have the Bottom Navigation bar.
You need the version of NS3 to use this plugin.
tns plugin add nativescript-bottom-navigation
Before start using the plugin you need to add the icons for android & iOS in your App_Resources directory.
You can set the tabs using the tabs
property
<Page xmlns="http://schemas.nativescript.org/tns.xsd"
xmlns:bottomNav="nativescript-bottom-navigation"
loaded="pageLoaded"
class="page">
<GridLayout columns="*"
rows="*, auto">
<StackLayout row="0">
<Label text="content"></Label>
</StackLayout>
<bottomNav:BottomNavigation tabs="{{ tabs }}"
activeColor="green"
inactiveColor="red"
backgroundColor="black"
loaded="bottomNavigationLoaded"
row="1"></bottomNav:BottomNavigation>
</GridLayout>
</Page>
import * as observable from 'tns-core-modules/data/observable';
import * as pages from 'tns-core-modules/ui/page';
import { BottomNavigation, BottomNavigationTab, OnTabSelectedEventData } from "nativescript-bottom-navigation";
// Event handler for Page 'loaded' event attached in main-page.xml
export function pageLoaded(args: observable.EventData) {
// Get the event sender
let page = <pages.Page>args.object;
page.bindingContext = {
tabs: [
new BottomNavigationTab('First', 'ic_home'),
new BottomNavigationTab('Second', 'ic_view_list'),
new BottomNavigationTab('Third', 'ic_menu')
]
};
}
export function bottomNavigationLoaded(args) {
let bottomNavigation: BottomNavigation = args.object;
// For some reason the tabSelected event doesn't work if you
// handle it from the view, so you need to handle the event in this way.
bottomNavigation.on('tabSelected', tabSelected);
}
export function tabSelected(args: OnTabSelectedEventData) {
console.log('tab selected ' + args.newIndex);
}
or you can add the tabs directly in your xml view
<Page xmlns="http://schemas.nativescript.org/tns.xsd"
xmlns:bottomNav="nativescript-bottom-navigation"
loaded="pageLoaded"
class="page">
<GridLayout columns="*"
rows="*, auto">
<StackLayout row="0">
<Label text="content"></Label>
</StackLayout>
<bottomNav:BottomNavigation activeColor="green"
inactiveColor="red"
backgroundColor="black"
loaded="bottomNavigationLoaded"
row="1">
<bottomNav:BottomNavigationTab title="First" icon="ic_home"></bottomNav:BottomNavigationTab>
<bottomNav:BottomNavigationTab title="Second" icon="ic_view_list"></bottomNav:BottomNavigationTab>
<bottomNav:BottomNavigationTab title="Third" icon="ic_menu"></bottomNav:BottomNavigationTab>
</bottomNav:BottomNavigation>
</GridLayout>
</Page>
import * as observable from 'tns-core-modules/data/observable';
import * as pages from 'tns-core-modules/ui/page';
import { BottomNavigation, BottomNavigationTab, OnTabSelectedEventData } from "nativescript-bottom-navigation";
// Event handler for Page 'loaded' event attached in main-page.xml
export function pageLoaded(args: observable.EventData) {
// Get the event sender
let page = <pages.Page>args.object;
}
export function bottomNavigationLoaded(args) {
let bottomNavigation: BottomNavigation = args.object;
bottomNavigation.on('tabSelected', tabSelected);
}
export function tabSelected(args: OnTabSelectedEventData) {
console.log('tab selected ' + args.newIndex);
}
First you need to include the NativescriptBottomNavigationModule
in your app.module.ts
import { NativescriptBottomNavigationModule} from "nativescript-bottom-navigation/angular";
@NgModule({
imports: [
NativescriptBottomNavigationModule
],
...
})
As the examples in the Javascript/Typescript version you can use the tabs
property to set the BottomNavigationTabs
<GridLayout rows="*, auto">
<StackLayout row="0">
<Label text="content"></Label>
</StackLayout>
<BottomNavigation [tabs]="tabs"
activeColor="red"
inactiveColor="yellow"
backgroundColor="black"
(tabSelected)="onBottomNavigationTabSelected($event)"
row="1"></BottomNavigation>
</GridLayout>
or you can declare the BottomNavigationTabs in your html directly
<GridLayout rows="*, auto">
<StackLayout row="0">
<Label text="content"></Label>
</StackLayout>
<BottomNavigation activeColor="red"
inactiveColor="yellow"
backgroundColor="black"
(tabSelected)="onBottomNavigationTabSelected($event)"
row="1">
<BottomNavigationTab title="First" icon="ic_home"></BottomNavigationTab>
<BottomNavigationTab title="Second" icon="ic_view_list"></BottomNavigationTab>
<BottomNavigationTab title="Third" icon="ic_menu"></BottomNavigationTab>
</BottomNavigation>
</GridLayout>
import { Component, OnInit } from "@angular/core";
import { BottomNavigation, BottonNavigationTab, OnTabSelectedEventData } from 'nativescript-bottom-navigation';
@Component(
{
selector: "ns-app",
moduleId: module.id,
templateUrl: "./app.component.html",
}
)
export class AppComponent {
public tabs: BottonNavigationTab[] = [
new BottomNavigationTab('First', 'ic_home'),
new BottomNavigationTab('Second', 'ic_view_list'),
new BottomNavigationTab('Third', 'ic_menu')
];
onBottomNavigationTabSelected(args: OnTabSelectedEventData): void {
console.log(`Tab selected: ${args.oldIndex}`);
}
}
You can also use your css file to set or change the activeColor, inactiveColor & backgroundColor of the Bottom Navigation.
.botom-nav {
tab-active-color: green;
tab-inactive-color: black;
tab-background-color: blue;
}
** properties (bindable) = properties settable thew XML/HTML ** properties (internal) = properties settable thew JS/TS instance
Property | Required | Default | Type | Description |
---|---|---|---|---|
tabs | true | null | Array<BottomNavigationTab> | Array containing the tabs for the BottomNavigation |
activeColor | false | "blue" | String | Color of the BottomNavigationTab when it's selected |
inactiveColor | false | "gray" | String | Color of the BottomNavigationTab when it's not selected |
backgroundColor | false | "white" | String | Color of the BottomNavigation background |
Property | Required | Default | Type | Description |
---|---|---|---|---|
selectedTabIndex | true | 0 | Number | Index of the selected tab |
Property | Type | Description |
---|---|---|
selectTab(index: number) | Void | Select a tab programmatically |
createTabs(tabs: BottomNavigationTab[]) | Void | Create the tabs programmatically |
Property | Required | Default | Type | Description |
---|---|---|---|---|
title | true | null | String | Title of the tab |
icon | true | null | String | Icon of the tab |
FAQs
NativeScript plugin to add a bottom navigation component for Android & iOS
The npm package nativescript-bottom-navigation receives a total of 30 weekly downloads. As such, nativescript-bottom-navigation popularity was classified as not popular.
We found that nativescript-bottom-navigation demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.