nativescript-parallax
Advanced tools
Comparing version 0.1.1 to 1.0.0
@@ -16,2 +16,26 @@ "use strict"; | ||
exports.ParallaxView = ParallaxView; | ||
var Header = (function (_super) { | ||
__extends(Header, _super); | ||
function Header() { | ||
_super.apply(this, arguments); | ||
} | ||
return Header; | ||
}(nativescript_parallax_common_1.Header)); | ||
exports.Header = Header; | ||
var Content = (function (_super) { | ||
__extends(Content, _super); | ||
function Content() { | ||
_super.apply(this, arguments); | ||
} | ||
return Content; | ||
}(nativescript_parallax_common_1.Content)); | ||
exports.Content = Content; | ||
var Anchored = (function (_super) { | ||
__extends(Anchored, _super); | ||
function Anchored() { | ||
_super.apply(this, arguments); | ||
} | ||
return Anchored; | ||
}(nativescript_parallax_common_1.Anchored)); | ||
exports.Anchored = Anchored; | ||
//# sourceMappingURL=nativescript-parallax.android.js.map |
@@ -1,5 +0,19 @@ | ||
import {ParallaxViewCommon} from './nativescript-parallax.common'; | ||
import { | ||
ParallaxViewCommon, | ||
Header as HeaderTemplate, | ||
Content as ContentTemplate, | ||
Anchored as AnchoredTemplate | ||
} from './nativescript-parallax.common'; | ||
export class ParallaxView extends ParallaxViewCommon { | ||
} | ||
export class Header extends HeaderTemplate { | ||
} | ||
export class Content extends ContentTemplate { | ||
} | ||
export class Anchored extends AnchoredTemplate { | ||
} |
@@ -7,5 +7,34 @@ "use strict"; | ||
}; | ||
var app = require('application'); | ||
var _scrollViewModule = require('ui/scroll-view'); | ||
var _gridLayout = require('ui/layouts/grid-layout'); | ||
var _absoluteLayout = require('ui/layouts/absolute-layout'); | ||
var _view = require('ui/core/view'); | ||
var _label = require('ui/label'); | ||
var _stackLayout = require('ui/layouts/stack-layout'); | ||
var _color = require('color'); | ||
var Header = (function (_super) { | ||
__extends(Header, _super); | ||
function Header() { | ||
_super.apply(this, arguments); | ||
} | ||
return Header; | ||
}(_stackLayout.StackLayout)); | ||
exports.Header = Header; | ||
var Anchored = (function (_super) { | ||
__extends(Anchored, _super); | ||
function Anchored() { | ||
_super.apply(this, arguments); | ||
} | ||
return Anchored; | ||
}(_stackLayout.StackLayout)); | ||
exports.Anchored = Anchored; | ||
var Content = (function (_super) { | ||
__extends(Content, _super); | ||
function Content() { | ||
_super.apply(this, arguments); | ||
} | ||
return Content; | ||
}(_stackLayout.StackLayout)); | ||
exports.Content = Content; | ||
var ParallaxViewCommon = (function (_super) { | ||
@@ -16,19 +45,76 @@ __extends(ParallaxViewCommon, _super); | ||
_super.call(this); | ||
this._addChildFromBuilder = function (name, value) { | ||
if (value instanceof _view.View) { | ||
_this._childLayouts.push(value); | ||
} | ||
}; | ||
this._childLayouts = []; | ||
var headerView; | ||
var scrollView; | ||
var contentView; | ||
var scrollView = new _scrollViewModule.ScrollView(); | ||
var viewsToFade; | ||
var maxTopViewHeight; | ||
var controlsToFade; | ||
var wrapperStackLayout = new _stackLayout.StackLayout(); | ||
this._addChildFromBuilder = function (name, value) { | ||
if (value instanceof _view.View) { | ||
if (_this.content == null) { | ||
_this.content = wrapperStackLayout; | ||
var anchoredRow = new _absoluteLayout.AbsoluteLayout(); | ||
var row = new _gridLayout.ItemSpec(2, _gridLayout.GridUnitType.star); | ||
var column = new _gridLayout.ItemSpec(1, _gridLayout.GridUnitType.star); | ||
var invalidSetup = false; | ||
this.addRow(row); | ||
this.addColumn(column); | ||
this.verticalAlignment = 'top'; | ||
scrollView.verticalAlignment = 'top'; | ||
anchoredRow.verticalAlignment = 'top'; | ||
this._includesAnchored = false; | ||
this.on(_gridLayout.GridLayout.loadedEvent, function (data) { | ||
_this.addChild(scrollView); | ||
_this.addChild(anchoredRow); | ||
_gridLayout.GridLayout.setRow(scrollView, 1); | ||
_gridLayout.GridLayout.setRow(anchoredRow, 0); | ||
_gridLayout.GridLayout.setColumn(scrollView, 1); | ||
_gridLayout.GridLayout.setColumn(anchoredRow, 0); | ||
var wrapperStackLayout = new _stackLayout.StackLayout(); | ||
scrollView.content = wrapperStackLayout; | ||
_this._childLayouts.forEach(function (element) { | ||
if (element instanceof Header) { | ||
wrapperStackLayout.addChild(element); | ||
headerView = element; | ||
} | ||
wrapperStackLayout.addChild(value); | ||
}); | ||
_this._childLayouts.forEach(function (element) { | ||
if (element instanceof Content) { | ||
wrapperStackLayout.addChild(element); | ||
contentView = element; | ||
} | ||
}); | ||
_this._childLayouts.forEach(function (element) { | ||
if (element instanceof Anchored) { | ||
anchoredRow.addChild(element); | ||
anchoredRow.height = element.height; | ||
_this._includesAnchored = true; | ||
} | ||
}); | ||
if (headerView == null || contentView == null) { | ||
var warningText = new _label.Label(); | ||
warningText.text = "Parallax ScrollView Setup Invalid. You must have Header and Content tags"; | ||
warningText.color = new _color.Color('red'); | ||
warningText.textWrap = true; | ||
warningText.marginTop = 50; | ||
_this.addChild(warningText); | ||
if (headerView != null) { | ||
headerView.visibility = 'collapse'; | ||
} | ||
if (contentView != null) { | ||
contentView.visibility = 'collapse'; | ||
return; | ||
} | ||
} | ||
}; | ||
viewsToFade = []; | ||
scrollView = this; | ||
scrollView.on(_scrollViewModule.ScrollView.loadedEvent, function (data) { | ||
maxTopViewHeight = headerView.height; | ||
if (_this._includesAnchored) { | ||
anchoredRow.marginTop = maxTopViewHeight; | ||
if (app.android) { | ||
anchoredRow.marginTop = anchoredRow.marginTop - 5; | ||
} | ||
contentView.marginTop = anchoredRow.height; | ||
} | ||
viewsToFade = []; | ||
if (controlsToFade == null && _this.controlsToFade == null) { | ||
@@ -40,8 +126,5 @@ controlsToFade = []; | ||
} | ||
var prevOffset = -10; | ||
var topOpacity = 1; | ||
headerView = wrapperStackLayout.getChildAt(0); | ||
maxTopViewHeight = headerView.height; | ||
controlsToFade.forEach(function (id) { | ||
var newView = wrapperStackLayout.getViewById(id); | ||
var newView = headerView.getViewById(id); | ||
if (newView != null) { | ||
@@ -55,2 +138,6 @@ viewsToFade.push(newView); | ||
headerView.height = maxTopViewHeight; | ||
scrollView.on(_scrollViewModule.ScrollView.loadedEvent, function (data) { | ||
}); | ||
var prevOffset = -10; | ||
var topOpacity = 1; | ||
scrollView.on(_scrollViewModule.ScrollView.scrollEvent, function (args) { | ||
@@ -67,2 +154,19 @@ if (prevOffset <= scrollView.verticalOffset) { | ||
} | ||
if (_this._includesAnchored) { | ||
if (scrollView.verticalOffset <= maxTopViewHeight) { | ||
anchoredRow.marginTop = maxTopViewHeight - (scrollView.verticalOffset * 2); | ||
if (anchoredRow.marginTop > maxTopViewHeight) { | ||
anchoredRow.marginTop = maxTopViewHeight; | ||
} | ||
if (app.android) { | ||
anchoredRow.marginTop = anchoredRow.marginTop - 5; | ||
} | ||
} | ||
else { | ||
anchoredRow.marginTop = 0; | ||
} | ||
if (anchoredRow.marginTop < 0) { | ||
anchoredRow.marginTop = 0; | ||
} | ||
} | ||
if (scrollView.verticalOffset < maxTopViewHeight) { | ||
@@ -113,4 +217,4 @@ topOpacity = parseFloat((1 - (scrollView.verticalOffset * 0.01)).toString()); | ||
return ParallaxViewCommon; | ||
}(_scrollViewModule.ScrollView)); | ||
}(_gridLayout.GridLayout)); | ||
exports.ParallaxViewCommon = ParallaxViewCommon; | ||
//# sourceMappingURL=nativescript-parallax.common.js.map |
import * as app from 'application'; | ||
import * as _scrollViewModule from 'ui/scroll-view'; | ||
import * as _gridLayout from 'ui/layouts/grid-layout'; | ||
import * as _absoluteLayout from 'ui/layouts/absolute-layout'; | ||
import * as _view from 'ui/core/view'; | ||
import * as _frame from 'ui/frame'; | ||
import * as _pages from 'ui/page'; | ||
import * as _label from 'ui/label'; | ||
import * as _stackLayout from 'ui/layouts/stack-layout'; | ||
import * as _color from 'color'; | ||
export class ParallaxViewCommon extends _scrollViewModule.ScrollView implements _view.AddChildFromBuilder { | ||
private _controlsToFade: string; | ||
export class Header extends _stackLayout.StackLayout { | ||
} | ||
export class Anchored extends _stackLayout.StackLayout { | ||
} | ||
export class Content extends _stackLayout.StackLayout { | ||
} | ||
export class ParallaxViewCommon extends _gridLayout.GridLayout implements _view.AddChildFromBuilder { | ||
private _controlsToFade: string; | ||
private _childLayouts: _view.View[]; | ||
private _includesAnchored: boolean; | ||
get controlsToFade(): string { | ||
@@ -22,26 +37,85 @@ return this._controlsToFade; | ||
super(); | ||
let headerView: _view.View; | ||
let scrollView: _scrollViewModule.ScrollView; | ||
this._childLayouts = []; | ||
let headerView: Header; | ||
let contentView: Content; | ||
let scrollView: _scrollViewModule.ScrollView = new _scrollViewModule.ScrollView(); | ||
let viewsToFade: _view.View[]; | ||
let maxTopViewHeight: number; | ||
let controlsToFade: string[]; | ||
let anchoredRow: _absoluteLayout.AbsoluteLayout = new _absoluteLayout.AbsoluteLayout(); | ||
let row = new _gridLayout.ItemSpec(2, _gridLayout.GridUnitType.star); | ||
let column = new _gridLayout.ItemSpec(1, _gridLayout.GridUnitType.star); | ||
let invalidSetup = false; | ||
//creates a new stack layout to wrap the content inside of the plugin. | ||
let wrapperStackLayout = new _stackLayout.StackLayout(); | ||
//overides default addChildFromBuilder to insert the wrapper and then add any child views. | ||
this._addChildFromBuilder = (name: string, value: any) => { | ||
if (value instanceof _view.View) { | ||
if (this.content == null) { | ||
this.content = wrapperStackLayout; | ||
this.addRow(row); | ||
this.addColumn(column); | ||
//must set the vertical alignmnet or else there is issues with margin-top of 0 being the middle of the screen. | ||
this.verticalAlignment = 'top'; | ||
scrollView.verticalAlignment = 'top'; | ||
anchoredRow.verticalAlignment = 'top'; | ||
this._includesAnchored = false; | ||
this.on(_gridLayout.GridLayout.loadedEvent, (data: any) => { | ||
this.addChild(scrollView); | ||
this.addChild(anchoredRow); | ||
_gridLayout.GridLayout.setRow(scrollView, 1); | ||
_gridLayout.GridLayout.setRow(anchoredRow, 0); | ||
_gridLayout.GridLayout.setColumn(scrollView, 1); | ||
_gridLayout.GridLayout.setColumn(anchoredRow, 0); | ||
//creates a new stack layout to wrap the content inside of the plugin. | ||
let wrapperStackLayout = new _stackLayout.StackLayout(); | ||
scrollView.content = wrapperStackLayout; | ||
this._childLayouts.forEach(element => { | ||
if (element instanceof Header) { | ||
wrapperStackLayout.addChild(element); | ||
headerView = element; | ||
} | ||
wrapperStackLayout.addChild(value); | ||
}); | ||
this._childLayouts.forEach(element => { | ||
if (element instanceof Content) { | ||
wrapperStackLayout.addChild(element); | ||
contentView = element; | ||
} | ||
}); | ||
this._childLayouts.forEach(element => { | ||
if (element instanceof Anchored) { | ||
anchoredRow.addChild(element); | ||
anchoredRow.height = element.height; | ||
this._includesAnchored = true; | ||
} | ||
}); | ||
if (headerView == null || contentView == null) { | ||
let warningText = new _label.Label(); | ||
warningText.text = "Parallax ScrollView Setup Invalid. You must have Header and Content tags"; | ||
warningText.color = new _color.Color('red'); | ||
warningText.textWrap = true; | ||
warningText.marginTop = 50; | ||
this.addChild(warningText); | ||
if (headerView != null) { | ||
headerView.visibility = 'collapse'; | ||
} | ||
if (contentView != null) { | ||
contentView.visibility = 'collapse'; | ||
return; | ||
} | ||
} | ||
}; | ||
//not supported yet; | ||
viewsToFade = []; | ||
maxTopViewHeight = headerView.height; | ||
scrollView = <_scrollViewModule.ScrollView>this; | ||
if (this._includesAnchored) { | ||
anchoredRow.marginTop = maxTopViewHeight; | ||
if (app.android) { //helps prevent background leaking int on scroll; | ||
anchoredRow.marginTop = anchoredRow.marginTop - 5; // get rid of white line that happens on android | ||
} | ||
//pushes content down a to compensate for anchor. | ||
contentView.marginTop = anchoredRow.height; | ||
} | ||
scrollView.on(_scrollViewModule.ScrollView.loadedEvent, (data: _scrollViewModule.ScrollEventData) => { | ||
//sets up controls to fade in and out. | ||
viewsToFade = []; | ||
//scrollView = <_scrollViewModule.ScrollView>this; | ||
if (controlsToFade == null && this.controlsToFade == null) { | ||
@@ -53,13 +127,6 @@ controlsToFade = []; | ||
//setting this to negative 10 assures there is no confusion when starting the on scroll event. | ||
let prevOffset = -10; | ||
let topOpacity = 1; | ||
//first child always needs to be the headerView | ||
headerView = wrapperStackLayout.getChildAt(0); | ||
maxTopViewHeight = headerView.height; | ||
controlsToFade.forEach((id: string): void => { | ||
let newView: _view.View = wrapperStackLayout.getViewById(id); | ||
let newView: _view.View = headerView.getViewById(id); | ||
if (newView != null) { | ||
@@ -76,2 +143,9 @@ viewsToFade.push(newView); | ||
scrollView.on(_scrollViewModule.ScrollView.loadedEvent, (data: _scrollViewModule.ScrollEventData) => { | ||
//sets up controls to fade in and out. | ||
}); | ||
let prevOffset = -10; | ||
let topOpacity = 1; | ||
scrollView.on(_scrollViewModule.ScrollView.scrollEvent, (args: _scrollViewModule.ScrollEventData) => { | ||
@@ -89,3 +163,18 @@ if (prevOffset <= scrollView.verticalOffset) { | ||
} | ||
if (this._includesAnchored) { | ||
if (scrollView.verticalOffset <= maxTopViewHeight) { | ||
anchoredRow.marginTop = maxTopViewHeight - (scrollView.verticalOffset * 2); | ||
if (anchoredRow.marginTop > maxTopViewHeight) { | ||
anchoredRow.marginTop = maxTopViewHeight; | ||
} | ||
if (app.android) { | ||
anchoredRow.marginTop = anchoredRow.marginTop - 5; // get rid of white line that happens on android | ||
} | ||
} else { | ||
anchoredRow.marginTop = 0; | ||
} | ||
if (anchoredRow.marginTop < 0) { | ||
anchoredRow.marginTop = 0; | ||
} | ||
} | ||
//fades in and out label in topView | ||
@@ -104,2 +193,3 @@ if (scrollView.verticalOffset < maxTopViewHeight) { | ||
}); | ||
} | ||
@@ -122,4 +212,9 @@ | ||
} | ||
_addChildFromBuilder = (name: string, value: any) => { | ||
if (value instanceof _view.View) { | ||
this._childLayouts.push(value); | ||
} | ||
}; | ||
} | ||
@@ -16,2 +16,26 @@ "use strict"; | ||
exports.ParallaxView = ParallaxView; | ||
var Header = (function (_super) { | ||
__extends(Header, _super); | ||
function Header() { | ||
_super.apply(this, arguments); | ||
} | ||
return Header; | ||
}(nativescript_parallax_common_1.Header)); | ||
exports.Header = Header; | ||
var Content = (function (_super) { | ||
__extends(Content, _super); | ||
function Content() { | ||
_super.apply(this, arguments); | ||
} | ||
return Content; | ||
}(nativescript_parallax_common_1.Content)); | ||
exports.Content = Content; | ||
var Anchored = (function (_super) { | ||
__extends(Anchored, _super); | ||
function Anchored() { | ||
_super.apply(this, arguments); | ||
} | ||
return Anchored; | ||
}(nativescript_parallax_common_1.Anchored)); | ||
exports.Anchored = Anchored; | ||
//# sourceMappingURL=nativescript-parallax.ios.js.map |
@@ -1,5 +0,19 @@ | ||
import {ParallaxViewCommon} from './nativescript-parallax.common'; | ||
import { | ||
ParallaxViewCommon, | ||
Header as HeaderTemplate, | ||
Content as ContentTemplate, | ||
Anchored as AnchoredTemplate | ||
} from './nativescript-parallax.common'; | ||
export class ParallaxView extends ParallaxViewCommon { | ||
} | ||
export class Header extends HeaderTemplate { | ||
} | ||
export class Content extends ContentTemplate { | ||
} | ||
export class Anchored extends AnchoredTemplate { | ||
} |
{ | ||
"name": "nativescript-parallax", | ||
"version": "0.1.1", | ||
"version": "1.0.0", | ||
"description": "NativeScript Parallax View Plugin.", | ||
@@ -10,2 +10,5 @@ "main": "nativescript-parallax.js", | ||
"ios": "1.7.0" | ||
}, | ||
"tns-ios": { | ||
"version": "1.7.0" | ||
} | ||
@@ -15,3 +18,3 @@ }, | ||
"build": "tsc", | ||
"demo.ios": "npm run preparedemo && cd demo && tns emulate ios", | ||
"demo.ios": "npm run preparedemo && cd demo && tns run ios", | ||
"demo.android": "npm run preparedemo && cd demo && tns run android", | ||
@@ -18,0 +21,0 @@ "preparedemo": "npm run build && cd demo && tns plugin remove nativescript-parallax && tns plugin add .. && tns install", |
#NativeScript Parallax View Plugin. | ||
###Parallax Scroll | ||
[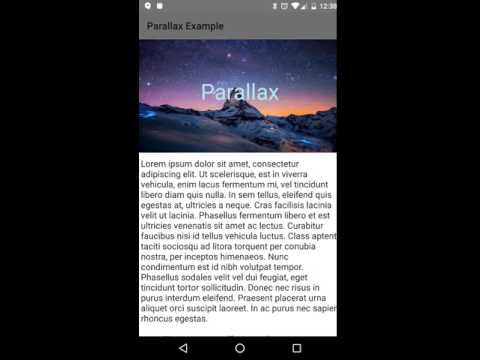](https://www.youtube.com/embed/sR_Ku7dsm2c) | ||
###Parallax Scroll with Anchor. | ||
[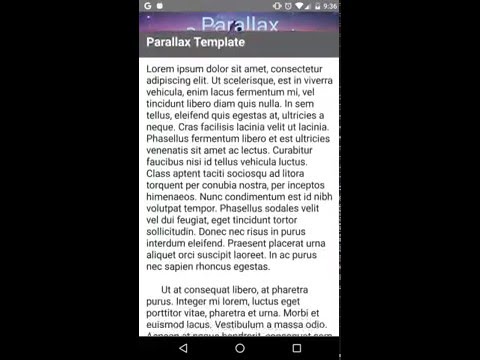](https://www.youtube.com/embed/uQsZr7PyGSM) | ||
###Install | ||
@@ -16,10 +17,11 @@ `$ tns plugin add nativescript-parallax` | ||
loaded="pageLoaded"> | ||
<StackLayout> | ||
<parallax:ParallaxView controlsToFade="headerLabel,headerLabel2"> | ||
<StackLayout class="header-template"> | ||
<parallax:Header class="header-template"> | ||
<Label id="headerLabel" text="Parallax"></Label> | ||
<Label id="headerLabel2" text="Component"></Label> | ||
</StackLayout> | ||
<StackLayout class="body-template"> | ||
</parallax:Header> | ||
<parallax:Anchored class="anchor"> | ||
<Label id="titleLabel" text="Parallax Template"></Label> | ||
</parallax:Anchored> | ||
<parallax:Content class="body-template"> | ||
<TextView editable="false" text="Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut scelerisque, est in viverra vehicula, enim lacus fermentum mi, vel tincidunt libero diam quis nulla. In sem tellus, eleifend quis egestas at, ultricies a neque. Cras facilisis lacinia velit ut lacinia. Phasellus fermentum libero et est ultricies venenatis sit amet ac lectus. Curabitur faucibus nisi id tellus vehicula luctus. Class aptent taciti sociosqu ad litora torquent per conubia nostra, per inceptos himenaeos. Nunc condimentum est id nibh volutpat tempor. Phasellus sodales velit vel dui feugiat, eget tincidunt tortor sollicitudin. Donec nec risus in purus interdum eleifend. Praesent placerat urna aliquet orci suscipit laoreet. In ac purus nec sapien rhoncus egestas. | ||
@@ -29,5 +31,4 @@ | ||
</TextView> | ||
</StackLayout> | ||
</parallax:Content> | ||
</parallax:ParallaxView> | ||
</StackLayout> | ||
</Page> | ||
@@ -54,5 +55,6 @@ | ||
background-image:url('~/images/mountains.png'); | ||
background-size:400 320; | ||
background-size: cover; | ||
height: 200; | ||
} | ||
.body-template textview{ | ||
.body-template TextView{ | ||
font-size:20; | ||
@@ -62,6 +64,12 @@ padding:5 15; | ||
} | ||
.anchor{ | ||
height: 55; | ||
width:100%; | ||
background-color:#616161; | ||
} | ||
#titleLabel{ | ||
font-weight:bold; | ||
font-size:30; | ||
font-size:25; | ||
padding:5 15; | ||
color:#fff; | ||
@@ -72,6 +80,18 @@ } | ||
when using the parallax plugin you need at least two layout views inside of the ``<parallax:ParallaxView>`` element. The first layout view being your header, which is seen in the above example with the background image. | ||
when using the parallax plugin you need at least two layout views inside of the ``<parallax:ParallaxView>`` element. ``<parallax:Header>`` and ``<parallax:Content>``. there is an optional third view called | ||
``<parallax:Anchored>`` which will positions it's self below the Header and once it reaches the top of the screen anchor it's self there. | ||
To add views such as labels you want to fade out in the ``<parallax:Parallaxiew>`` add ``controlsToFade`` and pass it a comma delimited string with each control ID you want to fade out. In the above example it looks like ``controlsToFade="headerLabel,headerLabel2"`` and will fade out both of those labels. | ||
If the inner content of ``<parallax:Content>`` isn't long enough to cause the page to scroll. If not it will not show the Parallax effect. | ||
###Work Flow. | ||
* Clone repository to your machine. | ||
* First run `npm install` | ||
* Then run `npm run setup` to prepare the demo project | ||
* Build with `npm run build` | ||
* Run and deploy to your device or emulator with `npm run demo.android` or `npm run demo.ios` | ||
###Special thanks to: | ||
@@ -78,0 +98,0 @@ [Nathanael Anderson](https://github.com/NathanaelA) for helping with understand how plugins in {N} work. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
42602
830
0
99