What is neo-async?
The neo-async package is a utility module which provides straight-forward, powerful functions for working with asynchronous JavaScript. It is similar to the async package but with some performance improvements.
What are neo-async's main functionalities?
Control Flow
Execute a series of functions in sequential order. Each function is passed a callback it must call on completion.
async.series([
function(callback) {
// do some stuff ...
callback(null, 'one');
},
function(callback) {
// do some more stuff ...
callback(null, 'two');
}
],
function(err, results) {
// results is now equal to ['one', 'two']
});
Collections
Apply a function to each item in a collection and collect the results.
async.map(['file1','file2','file3'], fs.stat, function(err, results) {
// results is now an array of stats for each file
});
Utilities
Call a function a certain number of times and collect the results.
async.times(5, function(n, next) {
createUser(n, function(err, user) {
next(err, user);
});
}, function(err, users) {
// we should now have 5 users
});
Other packages similar to neo-async
async
The original async package offers a wide array of functions for working with asynchronous code. Neo-async claims to offer similar functionality with improved performance.
bluebird
Bluebird is a full-featured promise library with a focus on innovative features and performance. It can be used as an alternative to neo-async for handling asynchronous operations using promises instead of callbacks.
q
Q is a tool for making and composing asynchronous promises in JavaScript. It's an older promise library that can serve similar purposes to neo-async but with a different style of handling async operations.
Neo-Async v1.7.3

Neo-Async is thought to be used as a drop-in replacement for Async, it almost fully covers its functionality and runs faster.
Async allows double callbacks in waterfall
, but Neo-Async does not allow. (test)
PR is welcome ! Especially improvement for English documents :)
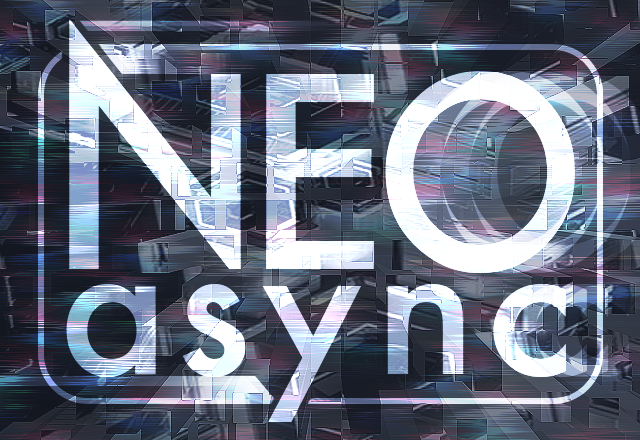

Installation
In a browser
<script src="async.min.js"></script>
In an AMD loader
require(['async'], function(async) {});
Node.js
standard
$ npm install neo-async
var async = require('neo-async');
var async = require('neo-async').safe;
replacement
$ npm install neo-async
$ ln -s ./node_modules/neo-async ./node_modules/async
var async = require('async');
Bower
bower install neo-async
Feature
JSDoc
* not in Async
Collections
Control Flow
Utils
Safe
var async = require('neo-async').safe;
var async = require('neo-async');
async.safe.each(collection, iterator, callback);
Speed Comparison
- async v1.3.0
- neo-async v1.3.0
Server-side
Speed comparison of server-side measured by func-comparator.
Specifications are as follows.
- n times trials
- Random execution order
- Measure the average speed[μs] of n times
execute
- 100 times trials
- 500000 tasks
Execution environment are as follows.
- node v0.10.40
- node v0.12.7
- iojs v2.3.4
$ node perf/func-comparator
result
The value is the ratio (Neo-Async/Async) of the average speed per n times.
collections
function | node v0.10.40 | node v0.12.7 | iojs v2.3.4 |
---|
each | 2.01 | 1.95 | 2.19 |
eachSeries | 2.28 | 2.62 | 2.28 |
eachLimit | 2.33 | 3.32 | 2.81 |
eachOf | 1.93 | 1.92 | 2.12 |
eachOfSeries | 2.17 | 2.79 | 2.98 |
eachOfLimit | 2.03 | 1.54 | 2.57 |
map | 3.10 | 3.11 | 3.38 |
mapSeries | 2.36 | 1.98 | 2.32 |
mapLimit | 1.76 | 1.84 | 2.06 |
filter | 2.33 | 3.70 | 6.59 |
filterSeries | 2.11 | 2.71 | 3.68 |
reject | 2.71 | 4.38 | 7.33 |
rejectSeries | 2.31 | 3.09 | 3.86 |
detect | 2.31 | 2.69 | 2.92 |
detectSeries | 2.13 | 1.96 | 2.71 |
reduce | 2.09 | 1.94 | 2.26 |
reduceRight | 2.19 | 1.93 | 2.51 |
sortBy | 1.41 | 1.66 | 1.52 |
some | 2.23 | 2.29 | 2.50 |
every | 2.22 | 2.25 | 2.93 |
concat | 12.0 | 7.23 | 10.0 |
concatSeries | 8.37 | 5.15 | 8.05 |
control flow
function | node v0.10.40 | node v0.12.7 | iojs v2.3.4 |
---|
parallel | 4.13 | 5.00 | 3.37 |
series | 3.13 | 2.70 | 3.03 |
parallelLimit | 2.69 | 2.96 | 2.49 |
waterfall | 3.45 | 7.24 | 7.59 |
whilst | 1.02 | 1.09 | 1.14 |
doWhilst | 1.26 | 1.36 | 1.28 |
until | 1.02 | 1.08 | 1.13 |
doUntil | 1.25 | 1.31 | 1.34 |
during | 2.15 | 2.08 | 2.08 |
doDuring | 5.08 | 5.77 | 5.39 |
times | 4.07 | 3.16 | 3.44 |
timesSeries | 2.82 | 2.58 | 2.71 |
timesLimit | 2.23 | 2.05 | 1.93 |