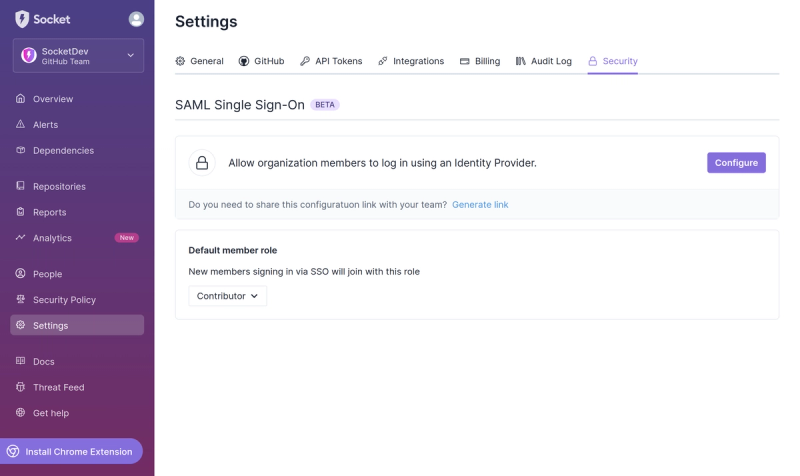
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
neo-async
Advanced tools
Package description
The neo-async package is a utility module which provides straight-forward, powerful functions for working with asynchronous JavaScript. It is similar to the async package but with some performance improvements.
Control Flow
Execute a series of functions in sequential order. Each function is passed a callback it must call on completion.
async.series([
function(callback) {
// do some stuff ...
callback(null, 'one');
},
function(callback) {
// do some more stuff ...
callback(null, 'two');
}
],
function(err, results) {
// results is now equal to ['one', 'two']
});
Collections
Apply a function to each item in a collection and collect the results.
async.map(['file1','file2','file3'], fs.stat, function(err, results) {
// results is now an array of stats for each file
});
Utilities
Call a function a certain number of times and collect the results.
async.times(5, function(n, next) {
createUser(n, function(err, user) {
next(err, user);
});
}, function(err, users) {
// we should now have 5 users
});
The original async package offers a wide array of functions for working with asynchronous code. Neo-async claims to offer similar functionality with improved performance.
Bluebird is a full-featured promise library with a focus on innovative features and performance. It can be used as an alternative to neo-async for handling asynchronous operations using promises instead of callbacks.
Q is a tool for making and composing asynchronous promises in JavaScript. It's an older promise library that can serve similar purposes to neo-async but with a different style of handling async operations.
Readme
Neo-Async is thought to be used as a drop-in replacement for Async, it almost fully covers its functionality and runs faster.
Benchmark is here!
Bluebird's benchmark is here!
<script src="async.min.js"></script>
require(['async'], function(async) {});
I recommend to use Aigle
.
It is optimized for Promise handling and has almost the same functionality as neo-async
.
$ npm install neo-async
var async = require('neo-async');
$ npm install neo-async
$ ln -s ./node_modules/neo-async ./node_modules/async
var async = require('async');
bower install neo-async
* not in Async
each
eachSeries
eachLimit
forEach
-> each
forEachSeries
-> eachSeries
forEachLimit
-> eachLimit
eachOf
-> each
eachOfSeries
-> eachSeries
eachOfLimit
-> eachLimit
forEachOf
-> each
forEachOfSeries
-> eachSeries
eachOfLimit
-> forEachLimit
map
mapSeries
mapLimit
mapValues
mapValuesSeries
mapValuesLimit
filter
filterSeries
filterLimit
select
-> filter
selectSeries
-> filterSeries
selectLimit
-> filterLimit
reject
rejectSeries
rejectLimit
detect
detectSeries
detectLimit
find
-> detect
findSeries
-> detectSeries
findLimit
-> detectLimit
pick
*pickSeries
*pickLimit
*omit
*omitSeries
*omitLimit
*reduce
inject
-> reduce
foldl
-> reduce
reduceRight
foldr
-> reduceRight
transform
transformSeries
*transformLimit
*sortBy
sortBySeries
*sortByLimit
*some
someSeries
someLimit
any
-> some
anySeries
-> someSeries
anyLimit
-> someLimit
every
everySeries
everyLimit
all
-> every
allSeries
-> every
allLimit
-> every
concat
concatSeries
concatLimit
*parallel
series
parallelLimit
tryEach
waterfall
angelFall
*angelfall
-> angelFall
*whilst
doWhilst
until
doUntil
during
doDuring
forever
compose
seq
applyEach
applyEachSeries
queue
priorityQueue
cargo
auto
autoInject
retry
retryable
iterator
times
timesSeries
timesLimit
race
apply
setImmediate
nextTick
memoize
unmemoize
ensureAsync
constant
asyncify
wrapSync
-> asyncify
log
dir
timeout
reflect
reflectAll
createLogger
$ node perf
The value is the ratio (Neo-Async/Async) of the average speed.
function | benchmark |
---|---|
each/forEach | 2.43 |
eachSeries/forEachSeries | 1.75 |
eachLimit/forEachLimit | 1.68 |
eachOf | 3.29 |
eachOfSeries | 1.50 |
eachOfLimit | 1.59 |
map | 3.95 |
mapSeries | 1.81 |
mapLimit | 1.27 |
mapValues | 2.73 |
mapValuesSeries | 1.59 |
mapValuesLimit | 1.23 |
filter | 3.00 |
filterSeries | 1.74 |
filterLimit | 1.17 |
reject | 4.59 |
rejectSeries | 2.31 |
rejectLimit | 1.58 |
detect | 4.30 |
detectSeries | 1.86 |
detectLimit | 1.32 |
reduce | 1.82 |
transform | 2.46 |
sortBy | 4.08 |
some | 2.19 |
someSeries | 1.83 |
someLimit | 1.32 |
every | 2.09 |
everySeries | 1.84 |
everyLimit | 1.35 |
concat | 3.79 |
concatSeries | 4.45 |
funciton | benchmark |
---|---|
parallel | 2.93 |
series | 1.96 |
waterfall | 1.29 |
whilst | 1.00 |
doWhilst | 1.12 |
until | 1.12 |
doUntil | 1.12 |
during | 1.18 |
doDuring | 2.42 |
times | 4.25 |
auto | 1.97 |
FAQs
Neo-Async is a drop-in replacement for Async, it almost fully covers its functionality and runs faster
The npm package neo-async receives a total of 19,812,268 weekly downloads. As such, neo-async popularity was classified as popular.
We found that neo-async demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.