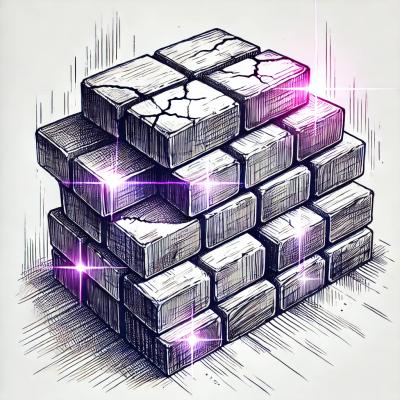
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
nest-jsonapi
Advanced tools
A Nest module that provides JSONAPI integration.
npm install --save nest-jsonapi
Import the JsonapiModule
into the root AppModule
and use the forRoot()
method to configure it:
import { JsonapiModule } from "nest-jsonapi";
@Module({
imports: [
JsonapiModule.forRoot({
// options
}),
],
})
export class AppModule {}
Inject JsonapiService
:
import { JsonapiService, JSONAPI_MODULE_SERVICE } from "nest-jsonapi";
@Controller("photos")
export class PhotosController {
constructor(@Inject(JSONAPI_MODULE_SERVICE) private readonly jsonapiService: JsonapiService) {}
}
Note that JsonapiModule
is a global module, therefore it will be available in all modules.
If you plan on using the JsonapiPayload
decorator (more info below), you must use the JsonapiMiddleware
in your application. This does 2 things:
e.g.
export class AppModule implements NestModule {
public configure(consumer: MiddlewareConsumer): void {
consumer.apply(JsonapiMiddleware).forRoutes(PhotosController);
}
}
The JsonapiInterceptor
is used to properly transform your controller result data to JSONAPI. You can decorate at a class or method level:
@UseInterceptors(JsonapiInterceptor)
@Controller("photos")
export default class PhotosController {}
In order for the JsonapiInterceptor
to know how to transform your data, you need to decorate your methods.
@JsonapiPayload({ resource: RESOURCE_PHOTOS })
@Get()
public async findPhotos(@Query() query: FindOptions): Promise<Photo[]>
In order to support error responses compliant with the JSONAPI specification, the JsonapiExceptionFilter
exists.
const { httpAdapter } = app.get(HttpAdapterHost);
app.useGlobalFilters(new JsonapiExceptionFilter(httpAdapter));
The JsonapiService
requires schemas for the resources it is going to handle. You have control of how that is configured, by defining a schematic. We provide a thin wrapper around the transformalizer
library.
Typically you will want to register your schemas on module initialization.
export class AppModule implements OnModuleInit {
constructor(@Inject(JSONAPI_MODULE_SERVICE) private readonly jsonapiService: JsonapiService) {}
public async onModuleInit(): Promise<void> {
const photoBuilder = new SchemaBuilder<Photo>(RESOURCE_PHOTOS);
photoBuilder.dataBuilder.untransformAttributes({ deny: ["createdAt", "updatedAt"] });
this.jsonapiService.register(photoBuilder);
}
}
nest-jsonapi-example is an example project that demonstrates the usage of this module. Since not all aspects of the module have been fully tested yet (coming soon!), I highly suggest checking this out!
For detailed API information please visit the API documentation.
See the Contributing guide for details.
This project is licensed under the MIT License.
FAQs
a NestJS module that provides JSONAPI integration
The npm package nest-jsonapi receives a total of 2 weekly downloads. As such, nest-jsonapi popularity was classified as not popular.
We found that nest-jsonapi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.