Nestia

Nestia is a set of helper libraries for NestJS, supporting below features:
@nestia/core
: super-fast decorators
- 20,000x faster validation
- 200x faster JSON serialization
@nestia/sdk
: evolved SDK and Swagger generators
- SDK (Software Development Kit)
- interaction library for client developers
- almost same with tRPC
nestia
: just CLI (command line interface) tool
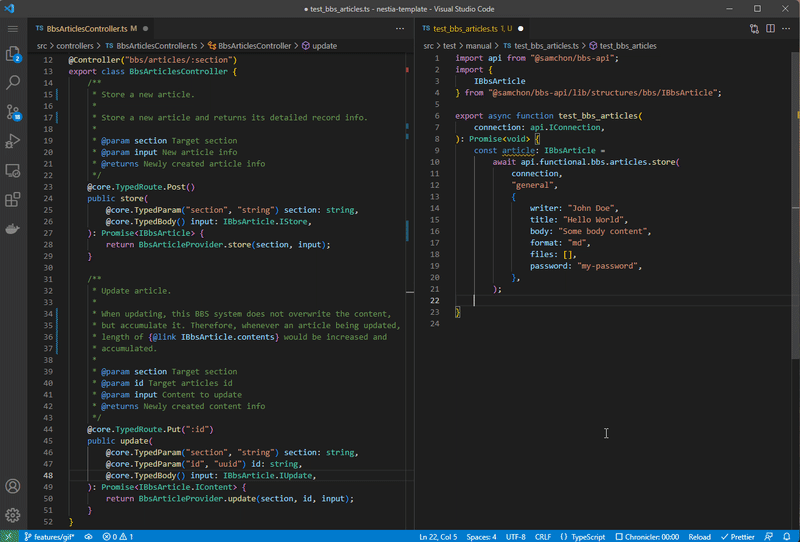
Sponsors and Backers
Thanks for your support.
Your donation would encourage nestia
development.

Setup
Boilerplate Project
npx nestia start <directory>
Just run above command, then boilerplate project would be constructed.
Setup Wizard
npm install --save-dev nestia
npx nestia setup
If you've installed ttypescript during setup, you should compile @nestia/core
utilization code through ttsc
command, instead of tsc
.
npx ttsc
npx ts-node -C ttypescript src/index.ts
Otherwise, you've chosen ts-patch, you can use original tsc
command. However, ts-patch hacks node_modules/typescript
source code. Also, whenever update typescript
version, you've to run npm run prepare
command repeatedly.
By the way, when using @nest/cli
, you must just choose ts-patch.
tsc
npx ts-node src/index.ts
npm install --save-dev typescript@latest
npm run prepare
Manual Setup
If you want to install and configure nestia
manually, read Guide Documents -> Setup.
@nestia/core

Super-fast validation decorators for NestJS.
- 15,000x faster request body validation
- 100x faster JSON response, even type safe
- Do not need DTO class definition, just fine with interface
@nestia/core
is a transformer library of NestJS, supporting super-fast validation decorators, by wrapping typia. Comparing validation speed with class-validator
, typia is maximum 15,000x times faster and it is even much safer.
Furthermore, @nestia/core
can use pure interface typed DTO with only one line. With @nestia/core
, you don't need any extra dedication like defining JSON schema (@nestjs/swagger
), or using class definition with decorator function calls (class-validator
). Just enjoy the superfast decorators with pure TypeScript type.
import { Controller } from "@nestjs/common";
import { TypedBody, TypedRoute } from "@nestia/core";
import type { IBbsArticle } from "@bbs-api/structures/IBbsArticle";
@Controller("bbs/articles")
export class BbsArticlesController {
@TypedRoute.Post()
public async store(
@TypedBody() input: IBbsArticle.IStore
): Promise<IBbsArticle>;
}
If you want to know more about this core library, visit Guide Documents.
- Decorators
- Enhancements
- Advanced Usage
@nestia/sdk

Automatic SDK and Swagger generator for @nestia/core.
With @nestia/core, you can boost up validation speed maximum 15,000x times faster. However, as @nestjs/swagger
does not support @nestia/core, you can't generate swagger documents from @nestjs/swagger
more.
Instead, I provide you @nestia/sdk
module, which can generate not only swagger documents, but also SDK (Software Development Kit) library. The SDK library can be utilized by client developers and it is almost same with tRPC
.
Usage
npx nestia <sdk|swagger> <source_directories_or_patterns> \
--exclude <exclude_directory_or_pattern> \
--out <output_directory_or_file>
npx nestia sdk "src/**/*.controller.ts" --out "src/api"
npx nestia swagger "src/controllers" --out "dist/swagger.json"
npx nestia sdk
npx nestia swagger
You can generate sdk or swagger documents by above commands.
If you want to know more, visit Guide Documents.
- Generators
- Advanced Usage
Demonstration
Here is example projects building Swagger Documents and SDK Library with npx nestia swagger
and npx nestia sdk
comands. @nestia/sdk
generates those documents and libraries by analyzing your NestJS backend server code in the compilation level.
Appendix
Typia
https://github.com/samchon/typia
@nestia/core
is wrapping typia
and the typia
is:

export function is<T>(input: unknown | T): input is T;
export function assert<T>(input: unknown | T): T;
export function validate<T>(input: unknown | T): IValidation<T>;
export const customValidators: CustomValidatorMap;
export function application<T>(): IJsonApplication;
export function assertParse<T>(input: string): T;
export function assertStringify<T>(input: T): string;
export function random<T>(g?: Partial<IRandomGenerator>): Primitive<T>;
typia
is a transformer library of TypeScript, supporting below features:
- Super-fast Runtime Validators
- Safe JSON parse and fast stringify functions
- JSON schema generator
- Random data generation
All functions in typia
require only one line. You don't need any extra dedication like JSON schema definitions or decorator function calls. Just call typia
function with only one line like typia.assert<T>(input)
.
Also, as typia
performs AOT (Ahead of Time) compilation skill, its performance is much faster than other competitive libaries. For an example, when comparing validate function is()
with other competitive libraries, typia
is maximum 20,000x times faster than class-validator
.