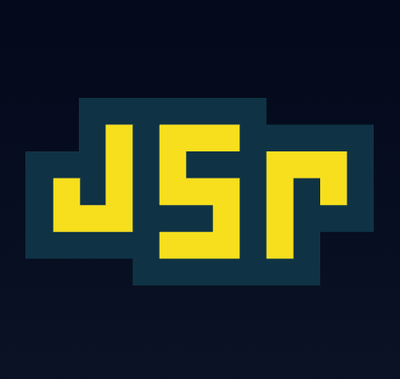
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The 'net' npm package provides an asynchronous network API for creating stream-based TCP or IPC servers and clients. It is a core module in Node.js, allowing developers to build network applications with ease.
Creating a TCP Server
This feature allows you to create a TCP server that listens for incoming connections on a specified port and IP address. The server can send and receive data from connected clients.
const net = require('net');
const server = net.createServer((socket) => {
socket.write('Hello from server!\n');
socket.on('data', (data) => {
console.log('Received:', data.toString());
});
});
server.listen(8080, '127.0.0.1', () => {
console.log('Server listening on port 8080');
});
Creating a TCP Client
This feature allows you to create a TCP client that connects to a specified server. The client can send and receive data from the server.
const net = require('net');
const client = net.createConnection({ port: 8080, host: '127.0.0.1' }, () => {
console.log('Connected to server!');
client.write('Hello from client!');
});
client.on('data', (data) => {
console.log('Received:', data.toString());
client.end();
});
client.on('end', () => {
console.log('Disconnected from server');
});
Handling Multiple Connections
This feature demonstrates how to handle multiple client connections on a TCP server. Each new connection is logged, and data can be sent and received from each client independently.
const net = require('net');
const server = net.createServer((socket) => {
console.log('New connection');
socket.write('Welcome!\n');
socket.on('data', (data) => {
console.log('Received:', data.toString());
});
socket.on('end', () => {
console.log('Connection closed');
});
});
server.listen(8080, '127.0.0.1', () => {
console.log('Server listening on port 8080');
});
The 'ws' package is a simple to use, blazing fast, and thoroughly tested WebSocket client and server for Node.js. Unlike 'net', which is for TCP connections, 'ws' is specifically designed for WebSocket connections, providing a higher-level protocol for real-time communication.
The 'socket.io' package enables real-time, bidirectional, and event-based communication. It works on every platform, browser, or device, focusing equally on reliability and speed. While 'net' is for low-level TCP connections, 'socket.io' abstracts many complexities and provides a more user-friendly API for real-time web applications.
The 'http' package is a core Node.js module for creating HTTP servers and clients. While 'net' is used for TCP connections, 'http' is specifically designed for handling HTTP requests and responses, making it more suitable for web server development.
FAQs
Globalizes the 'net' module functions
The npm package net receives a total of 211,757 weekly downloads. As such, net popularity was classified as popular.
We found that net demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.