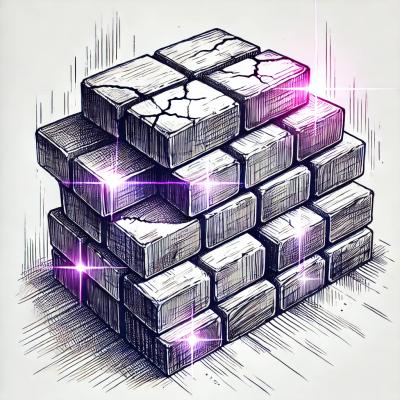
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
npm i netget
When a node (be it service or registry) is created, the constructor will gather details about the node.
constructor(options = {}) {
this.nodeType = options.nodeType || 'service'; // 'service' or 'registry'
this.metadata = options.metadata || {};
this.network = options.network || 'development';
this.registryNodes = new Set(options.registryNodes || []);
}
The node can be classified as either a 'service' node or a 'registry' node. Metadata contains information about the node, and the network determines which environment the node belongs to (e.g., 'development', 'production').
These methods allow the system to keep track of registry nodes. If Netget
is initialized as a service node, it will use these registry nodes to register itself.
addRegistryNode(node) {
this.registryNodes.add(node);
}
removeRegistryNode(node) {
this.registryNodes.delete(node);
}
When a node wants to register itself as a service node, the registerWithNetwork
method communicates with the registry nodes to do so.
registerWithNetwork() {
// Communicate with registry nodes to register this node.
// Send necessary details like metadata, IP address, etc.
}
This function allows other services or clients to discover and retrieve details of a specific service node.
discoverServiceNode(serviceName) {
// Contact registry nodes to find the service node by name.
// Return the details of the found service node.
}
If needed, Netget
can manage a distributed list of nodes and sync them. This would likely involve more advanced methods, such as using a Distributed Hash Table (DHT) or a decentralized protocol.
Security is vital. The module will have private methods (prefixed with _
for convention) to ensure communications are secure and validate node authenticity during registration.
_validateNode() {
// Logic to validate a node's authenticity during registration
}
The network
property determines the environment the node belongs to. This allows for separation and management of different networks.
A private method can periodically check the health status of nodes. This ensures that all nodes in the network are active and responsive.
_checkNodeHealth() {
// Logic to periodically check the health of nodes.
// Remove or flag nodes that are offline or unresponsive.
}
The classification of a node as either a 'service' node or a 'registry' node dictates its primary responsibilities and functionalities within the Netget
framework. Let's break down each type of node:
A Service Node is essentially a participant in the network that provides a specific functionality or service. This could be anything from a database service, an API endpoint, a web application, or any other service that other nodes or clients might want to interact with.
Characteristics of a Service Node:
Initiation of a Service Node:
const node = new Netget({
nodeType: 'service',
metadata: {
name: 'DatabaseService',
description: 'Provides database functionalities',
// ... other metadata
},
network: 'development',
registryNodes: ['http://registry1.netget.me', 'http://registry2.netget.me']
});
node.registerWithNetwork();
A Registry Node serves as a directory or lookup service for the network. It maintains a list of all Service Nodes, allowing clients or other nodes to discover and communicate with any Service Node they need.
Characteristics of a Registry Node:
Initiation of a Registry Node:
const registry = new Netget({
nodeType: 'registry',
network: 'development',
registryNodes: [] // Note: It's a registry node itself, so it might not need to register with other registry nodes.
});
// Additional initialization specific to registry functionalities...
In the Netget
system, these two types of nodes work in tandem. Service Nodes ensure that services are available and operational, while Registry Nodes make sure that these services can be easily found and accessed.
In essence, Netget
acts as a facilitator for nodes in a network. If a new service is spun up and wants to be discoverable, it will use Netget
to register with the known registry nodes. When another service or client wants to find a service, it will ask Netget
to discover it for them. The entire module serves as a manager for network-related tasks, abstracting away the intricacies of node management, service discovery, and network health.
This modular design ensures that Netget
remains separate from the application logic. Whether the end application uses Express, Next.js, or any other framework, Netget
can be easily integrated to manage networking tasks, ensuring that application developers can focus on building their application without worrying about the complexities of the network layer.
Enhance netget to support the concept of registry nodes and service nodes. Service nodes, when initialized, use netget to register with the network. netget handles the process of finding registry nodes, validating the service node, and updating its status. Additionally, netget can provide methods for service discovery, where clients can find the address and service details of a particular node.
Service Node Initialization: netget will provide a simple API that allows any node to initialize itself as a service node. During initialization, the service node will specify its metadata, including service details, preferred network (e.g., development, production, custom), and other information. Registry Node Management:
netget must have a way to identify and communicate with registry nodes. It could come pre-configured with a list of trusted registry nodes, or nodes might specify them during initialization. Service Registration:
When a service node is initialized, netget will handle the registration process with the registry nodes. This includes sharing the node's metadata, IP address, and validating its authenticity. Service Discovery:
netget will provide APIs that allow any client or service to query for a specific service node. It will connect to registry nodes, find the requested service details, and return them to the client. Decentralization Support:
If decentralization is a goal, netget needs mechanisms to manage a distributed list of nodes and synchronize this data. For example, using a DHT approach as mentioned previously. Security & Validation:
netget should ensure all communications are secure, possibly using cryptographic techniques. It should provide methods for validating the authenticity of nodes during the registration process. Network Management:
Nodes should be able to specify which network they want to be part of (e.g., development, production). netget will manage these networks separately, ensuring isolation between them. Node Health & Monitoring:
netget could have built-in features to periodically check the health of registered nodes. If a node is found to be offline or unresponsive, it could be temporarily removed from the active nodes list.
Welcome to the THIS.ME Playground, where the entire THIS.ME suite comes together with NEURONS.ME to provide a rich development and execution environment for your AI adventures.
git clone https://github.com/suiGn/.me.git
cd .me
You can use either Yarn or npm to install the necessary dependencies. Using Yarn:
yarn install
Using npm:
npm install
npx electron index.js
If you have suggestions or issues, please open an issue. We encourage contributions from the community.
This project is licensed under the MIT License. See the LICENSE file for details. This README provides an overview of the project, instructions for installation and usage, and highlights future projections. Feel free to modify or expand it as needed. Congratulations on building this exciting tool, and good luck with its continued development!
FAQs
Rette Adepto/ Recibido Directamente.
The npm package netget receives a total of 16 weekly downloads. As such, netget popularity was classified as not popular.
We found that netget demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.