Comparing version 0.0.4 to 0.1.0
104
index.js
@@ -1,86 +0,44 @@ | ||
module.exports = newStruct; | ||
var methodify = require("methodify"); | ||
var mix = require("mix-objects"); | ||
function newStruct (content){ | ||
var struct = Object.create(content), | ||
props = create.props = [], | ||
methods = create.methods = []; | ||
module.exports = define; | ||
var key; | ||
for (key in struct) { | ||
if (typeof struct[key] == 'function') { | ||
methods.push(key); | ||
} else { | ||
props.push(key); | ||
} | ||
function define () { | ||
var functions = arguments[arguments.length - 1]; | ||
var i = arguments.length - 1; | ||
while (i--) { | ||
mix.one(functions, arguments[i].methods); | ||
} | ||
function create (values){ | ||
var copy = Object.create(struct), | ||
key, i; | ||
i = undefined; | ||
constructor.methods = functions; | ||
constructor.from = constructFrom; | ||
constructor.isAStruct = true; | ||
if (arguments.length == 1 && typeof values == 'object') { | ||
for (key in values) { | ||
copy[key] = values[key]; | ||
} | ||
} else { | ||
i = arguments.length; | ||
while (i--) { | ||
copy[ props[i] ]= arguments[i]; | ||
} | ||
} | ||
extractStaticMethods(functions, constructor); | ||
i = methods.length; | ||
while (i --) { | ||
copy[methods[i]] = wrapMethod(copy, struct[methods[i]]); | ||
} | ||
return constructor; | ||
if (struct.construct) { | ||
struct.construct(copy); | ||
} | ||
function constructor (object) { | ||
return methodify(object, functions); | ||
} | ||
return copy; | ||
}; | ||
function constructFrom () { | ||
var mixwith = Array.prototype.slice.call(arguments, 0, -1); | ||
var object = arguments[arguments.length - 1]; | ||
create.extend = function(ext){ | ||
var config = Object.create(content), | ||
supers = {}, | ||
create; | ||
mix(object, mixwith); | ||
var key; | ||
for (key in ext) { | ||
if (typeof config[key] == 'function') { | ||
supers[key] = config[key]; | ||
} | ||
config[key] = ext[key]; | ||
} | ||
create = newStruct(config); | ||
create.supers = supers; | ||
var ind; | ||
for (key in ext) { | ||
if (typeof ext[key] != 'function') { | ||
create.props.splice(create.props.indexOf(key), 1); | ||
} | ||
} | ||
return create; | ||
}; | ||
create.method = function (name, fn){ | ||
methods.push(name); | ||
struct[name] = fn; | ||
return create; | ||
}; | ||
return create; | ||
return methodify(object, functions); | ||
} | ||
} | ||
function wrapMethod (copy, method){ | ||
return function(){ | ||
var args = Array.prototype.slice.call(arguments); | ||
args.splice(0, 0, copy); | ||
return method.apply(undefined, args); | ||
}; | ||
function extractStaticMethods (from, to) { | ||
var key; | ||
for (key in from) { | ||
if (typeof from[key] != 'function' || key[0] != key[0].toUpperCase()) continue; | ||
to[key] = from[key]; | ||
delete from[key]; | ||
} | ||
} |
{ | ||
"name": "new-struct", | ||
"version": "0.0.4", | ||
"version": "0.1.0", | ||
"description": "Structs inspired from Golang", | ||
"main": "index.js", | ||
"scripts": { | ||
"test": "fox" | ||
"test": "prova" | ||
}, | ||
"dependencies": { | ||
"mix-objects": "0.0.2", | ||
"methodify": "0.0.2" | ||
}, | ||
"devDependencies": { | ||
"fox": "*" | ||
"prova": "*" | ||
}, | ||
@@ -12,0 +16,0 @@ "keywords": [ |
151
README.md
## new-struct | ||
Structs inspired from Golang | ||
A minimalistic class system designed for flexibility, functional programming. Inspired from Golang's Struct concept. | ||
Motivation: | ||
* `new-struct` is small and simple. All it does is composing functions and objects. | ||
* It doesn't have `new` and `this` keywords. So, you'll never have to fix scopes. | ||
* You can do currying, partial programming with both `new-struct` and structs. | ||
How does a struct look? | ||
```js | ||
newStruct = require('new-struct') | ||
var Struct = require('new-struct') | ||
Animal = newStruct({ | ||
name: '', | ||
type: '', | ||
age: 0, | ||
run: run | ||
var Animal = Struct({ | ||
sleep: sleep, | ||
speak: speak | ||
}) | ||
function run (animal) { | ||
console.log('%s is running', animal.name) | ||
} | ||
module.exports = Animal; | ||
dongdong = Animal('dongdong', 'cat', 4) | ||
blackbear = Animal('blackbear', 'cat', 3) | ||
function sleep (animal) { console.log('zzzz'); } | ||
dongdong.run() | ||
// => dongdong is running | ||
blackbear.run() | ||
// => blackbear is running | ||
function speak (animal, text) { | ||
console.log('%s says %s', animal.name, text); | ||
} | ||
``` | ||
Check out [Usage](#usage) for more info about it. | ||
## Install | ||
@@ -37,51 +40,113 @@ | ||
You can pass the values as an object, too; | ||
A new struct is defined by an object of methods: | ||
```js | ||
dongdong = Animal({ type: 'cat', foobar: 'dfjh' }) | ||
Struct = require('new-struct') | ||
Animal = Struct({ | ||
sleep: sleep, | ||
run: run, | ||
speak: speak | ||
}) | ||
function sleep (animal) { | ||
console.log('zzz'); | ||
} | ||
function speak (animal, sound) { | ||
console.log('%s says %s', animal.name, sound) | ||
} | ||
function run (animal) { | ||
console.log('%s is running', animal.name) | ||
} | ||
``` | ||
Methods can also be defined later; | ||
To create a an instance of the `Animal` struct, just call it with an object. | ||
`this` and `new` keywords are not needed, everything is just functions. | ||
``` | ||
dongdong = Animal({ name: 'dongdong' }) | ||
blackbear = Animal({ name: 'blackbear' }) | ||
dongdong.name | ||
// => 'dong dong' | ||
dongdong.run() | ||
// dongdong is running | ||
blackbear.sleep() | ||
// blackbear is sleeping | ||
``` | ||
### Factory Functions | ||
It doesn't support constructors, but constructor-like factory functions are easy to implement: | ||
```js | ||
Animal.method('jump', function(animal){ | ||
console.log('%s is jumping!!', animal.name) | ||
}) | ||
function NewAnimal (name, age) { | ||
return Animal({ name: name, age: age }) | ||
} | ||
``` | ||
To extend an existing struct: | ||
Note that you can attach your constructor as a static method. So, you could have such a module: | ||
```js | ||
Cat = Animal.extend({ | ||
type: 'cat', | ||
grrr: grrr | ||
Animal = Struct({ | ||
New: New, | ||
run: run, | ||
speak: speak | ||
}) | ||
function grrr(cat) { | ||
console.log('grrrrrr') | ||
module.exports = Animal; | ||
function New (name, age) { | ||
return Animal({ name: name, age: age }) | ||
} | ||
dongdong = Cat('dongdong', 2) // notice how 'type' property got eleminated from parameter order. | ||
function speak (animal, sound) { | ||
console.log('%s says %s', animal.name, sound) | ||
} | ||
function run (animal) { | ||
console.log('%s is running', animal.name) | ||
} | ||
``` | ||
To define a constructor method: | ||
This will allow other modules requiring this have more flexibility: | ||
```js | ||
Animal = newStruct({ | ||
construct: construct, | ||
name: '', | ||
type: '', | ||
age: 0, | ||
run: run | ||
Animal = require('./animal') | ||
// You can either create using constructor: | ||
Animal.New('dong dong', 13) | ||
// Or calling the constructor itself: | ||
Animal({ name: 'dong dong', age: 13 }) | ||
// You can also access the methods of Animal: | ||
Animal.methods.run({ name: 'black bear' }) | ||
// will output: black bear is running | ||
``` | ||
### Mixing | ||
You can create structs that mixes other ones: | ||
```js | ||
Animal = require('./animal') | ||
Cat = Struct(Animal, { | ||
meow: meow | ||
}) | ||
function construct (animal) { | ||
animal.log = console.log | ||
animal.log('%s just born!', animal.name) | ||
function meow (cat) { | ||
Animal.methods.speak(cat, 'meooww') | ||
} | ||
dondong = Animal('dongdong', 'cat') | ||
// => dongdong just born | ||
``` | ||
Notice that each struct has a property called `methods` that keeps all the functions passed to it, including the ones derived from other structs. | ||
See the tests and examples for more info, or create issues & send pull requests to improve the documentation. | ||
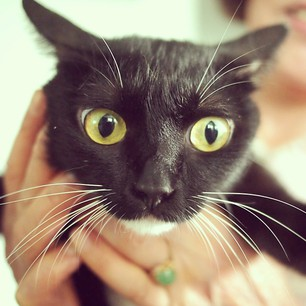 |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
4570
152
2
33
+ Addedmethodify@0.0.2
+ Addedmix-objects@0.0.2
+ Addedmethodify@0.0.2(transitive)
+ Addedmix-objects@0.0.2(transitive)