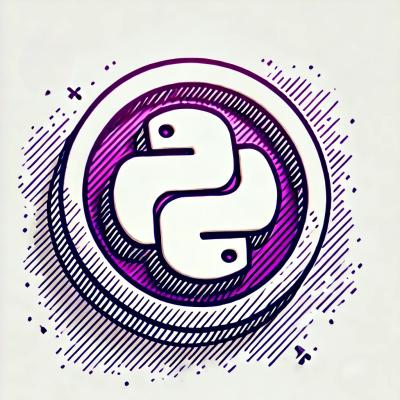
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
next-auth
Advanced tools
NextAuth.js is a complete open-source authentication solution for Next.js applications. It provides a simple and secure way to add authentication to your Next.js app, supporting various authentication providers, including OAuth, email/password, and custom credentials.
OAuth Authentication
NextAuth.js supports OAuth authentication with various providers like Google, Facebook, GitHub, etc. The code sample demonstrates how to configure Google and Facebook OAuth providers.
```javascript
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET
}),
Providers.Facebook({
clientId: process.env.FACEBOOK_CLIENT_ID,
clientSecret: process.env.FACEBOOK_CLIENT_SECRET
})
]
});
```
Email/Password Authentication
NextAuth.js allows email/password authentication by sending magic links to users' email addresses. The code sample shows how to configure the email provider.
```javascript
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Email({
server: process.env.EMAIL_SERVER,
from: process.env.EMAIL_FROM
})
]
});
```
Custom Credentials Authentication
NextAuth.js supports custom credentials authentication, allowing you to define your own logic for authenticating users. The code sample demonstrates a simple username/password authentication.
```javascript
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Credentials({
name: 'Credentials',
credentials: {
username: { label: 'Username', type: 'text' },
password: { label: 'Password', type: 'password' }
},
authorize: async (credentials) => {
const user = { id: 1, name: 'User' };
if (credentials.username === 'user' && credentials.password === 'password') {
return Promise.resolve(user);
} else {
return Promise.resolve(null);
}
}
})
]
});
```
Session Management
NextAuth.js provides session management, allowing you to access the user's session data on both the client and server sides. The code sample shows how to retrieve the session in a Next.js page using getServerSideProps.
```javascript
import { getSession } from 'next-auth/client';
export async function getServerSideProps(context) {
const session = await getSession(context);
return {
props: { session }
};
}
```
Passport is a popular authentication middleware for Node.js. It is highly flexible and supports a wide range of authentication strategies. Compared to NextAuth.js, Passport requires more manual setup and configuration, but it offers greater flexibility and can be used with any Node.js framework.
Auth0 is a cloud-based authentication service that provides a comprehensive solution for authentication and authorization. It supports various authentication methods and integrates well with many platforms. Compared to NextAuth.js, Auth0 is a third-party service that requires a subscription for advanced features, but it offers a more extensive set of features and easier integration with multiple platforms.
Firebase Authentication is a part of Google's Firebase platform, providing backend services to authenticate users in your app. It supports email/password, phone number, and various social providers. Compared to NextAuth.js, Firebase Authentication is a managed service that integrates seamlessly with other Firebase services, but it may not offer the same level of customization as NextAuth.js.
This is work in progress of version 2.0 of NextAuth, an authentication library for Next.js.
Version 2.0 is a complete re-write, designed from the ground up for serverless.
If you are familiar with version 1.x you will appreciate the much simpler and hassle free configuration, especially for provider configuration, database adapters and much improved Cross Site Request Forgery token handling (now enabled by default for next-auth routes only).
Additional options and planned features will be announced closer to release.
Note: NextAuth is not associated with Next.js or Vercel.
Configuration is much simpler and more powerful than in NextAuth 1.0, with both SQL and Document databases supported out of the box. There are predefined models for Users and Sessions, which you can use (or extend or replace with your own models/schemas).
To add next-auth
to a project, create a file to handle authentication requests at pages/api/auth/[...slug.js]
:
import NextAuth from 'next-auth'
import Providers from 'next-auth/providers'
import Adapters from 'next-auth/adapters'
const options = {
site: process.env.SITE_NAME || 'http://localhost:3000',
providers: [
Providers.Twitter({
clientId: process.env.TWITTER_CLIENT_ID,
clientSecret: process.env.TWITTER_CLIENT_SECRET,
}),
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET
}),
Providers.GitHub({
clientId: process.env.GITHUB_CLIENT_ID,
clientSecret: process.env.GITHUB_CLIENT_SECRET
}),
],
adapter: Adapters.Default()
}
export default (req, res) => NextAuth(req, res, options)
All requests to pages/api/auth/*
(signin, callback, signout) will now be automatically handed by NextAuth.
You can use the useSession()
hook to see if a user is signed in.
export default () => {
const [session, loading] = NextAuth.useSession()
return <>
{loading && <p>Loading session…</p>}
{!loading && session && <p>Logged in as {session.user.name || session.user.email}.</p>}
{!loading && !session && <p>Not logged in.</p>}
</>
}
This is all the code you need to add support for signing in to a project!
Authentication in Server Side Rendering flows is also supported.
import NextAuth from 'next-auth/client'
export default ({ session }) => <>
{session && <p>You are logged in as {session.user.name || session.user.email}.</p>}
{!session && <p>You are not logged in.</p>}
</>
export async function getServerSideProps({req}) {
const session = await NextAuth.session({req})
return {
props: {
session
}
}
}
You can use this method and the useSession()
hook together - the hook can be pre-populated with the session object from the server side call, so that it is avalible immediately when the page is loaded, and updated client side when the page is viewed in the browser.
You can also call NextAuth.session()
function in client side JavaScript, without needing to pass a req
object - it is only needed when calling the function from getServerSideProps
or getInitialProps
.
Authentication between the client and server is handled securely, using an HTTP only cookie for the session ID.
Important! The API for 2.0 is subject to change before release.
Configuration options are passed to NextAuth when initalizing it (in your /api/
route).
The only things you will probably need to configure are your site name (e.g. 'http://www.example.com'), which should be set explicitly for security reasons, a list of authentication services (Twitter, Facebook, Google, etc) and a database adapter.
An "Adapter" in NextAuth is the thing that connects your application to whatever system you want to use to store data for user accounts, sessions, etc.
NextAuth comes with a default adapter that uses TypeORM so that it can be be used with many different databases without any configuration, you simply add the database driver you want to use to your project and tell NextAuth to use it.
This is an example of how to use an SQLite in memory database, which can be useful for development and testing, and to check everything is working:
npm i sqlite3
NextAuth()
in your API route.e.g.
adapter: Adapters.Default({
type: 'sqlite',
database: ':memory:'
}),
You can pass database credentials securely, using environment variables for options.
See the TypeORM configuration documentation for more details about supported options.
The following databases are supported by the default adapter:
Appropriate tables / collections for Users, Sessions (etc) will be created automatically. You can customize, extend or replace the models by passing additional options to the Adapters.Default()
function.
If you are using a database that is not supported out of the box - or if you want to use NextAuth with an existing database (or have a more complex setup, with accounts and sessions spread across different systems - you can pass your own methods to be called for user and session creation / deletion (etc).
Important! The list of supported databases is subject to change before release.
NextAuth automatically crates simple, unbranded authentication pages for handling Sign in, Email Verification, callbacks, etc.
These are generated based on the configuration supplied, You can create custom authentication pages if you would like to customize the experience.
This documentation will be updated closer to release.
FAQs
Authentication for Next.js
The npm package next-auth receives a total of 215,160 weekly downloads. As such, next-auth popularity was classified as popular.
We found that next-auth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.