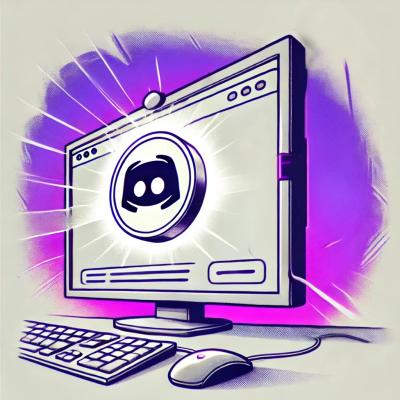
Research
Security News
Malicious PyPI Package ‘pycord-self’ Targets Discord Developers with Token Theft and Backdoor Exploit
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Easily compose and manage meta and open graph tags in your Next.js app/site.
Easily compose and manage meta and open graph tags in your Next.js app/site.
NOTE: This package is for use with Next.js’ Pages Router. Some App Router helpers are in the works and will live here in the future as well.
Via npm
npm install next-meta
Via Yarn
yarn add next-meta
Setting defaults within the Next.js App with MetaProvider
.
import { ReactElement, ReactNode } from 'react'
import { NextPage } from 'next'
import { AppProps } from 'next/app'
import Head from 'next/head'
import { usePathname } from 'next/navigation'
import { MetaProvider } from 'next-meta'
// eslint-disable-next-line @typescript-eslint/ban-types
export type NextPageWithLayout<P = {}, IP = P> = NextPage<P, IP> & {
getLayout?: (page: ReactElement) => ReactNode
}
type AppPropsWithLayout = AppProps & {
Component: NextPageWithLayout
}
const BASE_URL = 'https://test.com'
const SITE_NAME = 'Example Site'
const DEFAULT_TITLE = 'An example title for using next-meta in your _app file.'
const DEFAULT_DESCRIPTION = 'Hopefully this makes things a little easier with adding good meta/og tags to your site.'
const DEFAULT_IMAGE_URL = '/social-share.png'
function CustomApp({ Component, pageProps }: AppPropsWithLayout) {
const getLayout = Component.getLayout ?? ((page) => page)
const metaUrl = usePathname()
return (
<>
<Head>
<link rel="icon" type="image/png" href="/favicon.ico" />
</Head>
<MetaProvider
baseUrl={BASE_URL}
canonical={metaUrl}
description={DEFAULT_DESCRIPTION}
imageUrl={DEFAULT_IMAGE_URL}
imageWidth={1200}
imageHeight={630}
siteName={SITE_NAME}
title={DEFAULT_TITLE}
twitterCard="summary_large_image"
twitterSite="@exampleSite"
url={metaUrl}
>
{getLayout(<Component {...pageProps} />)}
</MetaProvider>
</>
)
}
export default CustomApp
Specifying page specific meta tags using the SiteMeta
component.
import Head from 'next/head'
import { SiteMeta } from 'next-meta'
const ExamplePage = () => (
return (
<>
<Head>
<SiteMeta
imageUrl="/share/about-social.png"
title="About"
siteName="Example Site"
url="/about"
/>
</Head>
{...page code...}
</>
)
)
Prop | Description |
---|---|
audioUrl?: string | URL to audio file. |
audioType?: string | Mimetype of audio file. |
baseUrl?: string | Used specify base url to use for all xUrl props, allowing you to simply pass in url="/about" vs. url="https://yourdomain.com/about" . |
description?: string | You know, <meta name="description" content="You know, a description" /> |
determiner?: string | The word that appears before this object's title in a sentence.An enum of (a, an, the, "", auto). If auto is chosen, the consumer of your data should chose between "a" or "an". Default is "" (blank). |
imageUrl?: string | URL to image. |
imageWidth?: number | string | Width of the image. (Typically: 1200px ) |
imageHeight?: number | string | Height of the image. (Typically: 630px ) |
locale?: string | Locale of site/page |
siteName?: string | Use for og:site_name and appended to <title> |
title?: string | Title of page. Generates: <title> + og:title + twitter:title tags |
twitterCard?: string | Twitter card display type. |
twitterCreator?: string | Username to associate with a page/post. |
twitterSite?: string | Username to associate with the site/app. |
url?: string | URL of page/site. |
videoUrl?: string | URL to video file. |
videoType?: string | Mimetype of the video file. |
FAQs
Easily compose and manage meta and open graph tags in your Next.js app/site.
The npm package next-meta receives a total of 21 weekly downloads. As such, next-meta popularity was classified as not popular.
We found that next-meta demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.