Next Rating Component
I've been searching for a reliable React rating component for Next.js with server-side rendering (SSR) support and essential features like fractional ratings. After trying several existing libraries over the past five days, I've found that many either don't work well with SSR, lack crucial functionality, or don't support fractions. Therefore, I've decided to build my own customizable rating component in TypeScript to meet common project needs.
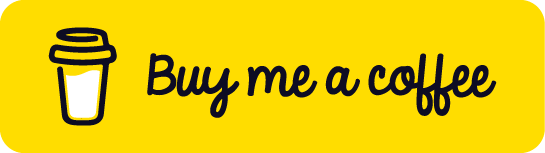
- Small in Size
- Zero Dependency
- Fractional step (It support any fractional value)
- Custom Icon
- All necessary options
Demo
See Live Demo
Installation
$ npm i next-rating-component
App Router
"use client"
import { useState } from "react";
import { Rating } from "next-rating-component";
const MyComponent = () => {
const [rating, setRating] = useState<number>(0);
return (
<div>
<Rating
value={rating}
onChange={(e) => setRating(e)}
fractions={0.1}
/>
</div>
);
};
export default MyComponent;
Available props
Name | Description | Type | Default/Required |
---|
maxRating | Rating star count | number | 5 |
value | The value of rating (You can use Rating component with controls using this value prop) | number | Required |
icon | Change the icon of Rating Component (Only SVG Icon supported) | React Element | Optional |
fractions | Fractional Step For Icon | number | 1 |
onChange | The new rating value when clicked | (newRating)=> void | Optional |
onHover | The new rating value when hovered | (newRating)=> void | ease |
readOnly | The interactivity of the icon | boolean | false |
Size | The Size of the Icon | number | 24 |
emptyColor | The color of the empty Icon | string | #dfe2e6 |
fillColor | The color of the fill Icon | string | #fab005 |
toolTip | For showing tooltip | boolean | false |
customTooltip | Custom tooltip for every step. Here step will 2 decimal position number for each step | {step:number, text:string}[] | optional |
Stay in touch