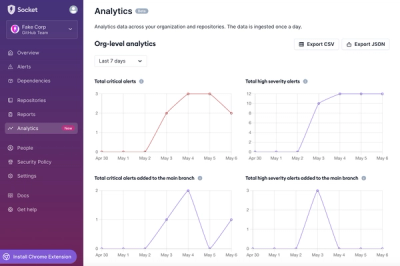
Product
Introducing Dashboard Analytics
We're introducing a new Analytics feature in the Socket dashboard so you can view changes in your organization's and repositories' alerts over time.
next-sanity
Advanced tools
Sanity.io toolkit for Next.js.
Features:
next-sanity
Running groq queries
next-sanity
Visual Editing with Content Source Mapsnext-sanity/preview
Preview drafts, hot reload on changesnext-sanity/studio
next-sanity/webhook
npm install next-sanity @sanity/client @portabletext/react @sanity/image-url
yarn add next-sanity @sanity/client @portabletext/react @sanity/image-url
pnpm install next-sanity @sanity/client @portabletext/react @sanity/image-url
next-sanity/studio
peer dependenciesWhen using npm
newer than v7
, or pnpm
newer than v8
, you should end up with needed dependencies like sanity
and styled-components
when you npm install next-sanity
. It also works in yarn
v1
using install-peerdeps
:
npx install-peerdeps --yarn next-sanity
next-sanity
Running groq queriesimport {createClient, groq} from 'next-sanity'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID // "pv8y60vp"
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET // "production"
const apiVersion = process.env.NEXT_PUBLIC_SANITY_API_VERSION // "2023-05-03"
const client = createClient({
projectId,
dataset,
apiVersion, // https://www.sanity.io/docs/api-versioning
useCdn: true, // if you're using ISR or only static generation at build time then you can set this to `false` to guarantee no stale content
})
const data = await client.fetch(groq`*[]`)
The perspective
option can be used to specify special filtering behavior for queries. The default value is raw
, which means no special filtering is applied, while published
and previewDrafts
can be used to optimize for specific use cases. Read more about this option:
useCdn
be true
or false
?The general rule is that useCdn
should be true
when:
useEffect
hook or in response to a user interaction where the client.fetch
call is made in the browser.And it makes sense to set useCdn
to false
when:
getStaticProps
or getStaticPaths
.stale-while-revalidate
caching is in place that keeps API requests on a consistent low, even if traffic to Next.js spikes.app-router
, React Server Components and cachingWe are working on bringing
fetch
support to@sanity/client
when used by Next.js'nodejs
runtime. If you're using theedge
runtime yourclient.fetch
calls are automatically deduped.
As @sanity/client
will only sometimes use fetch
under the hood, it depends on the environment, it's best to implement the cache function to ensure reliable deduping of requests.
import {createClient, groq} from 'next-sanity'
import {cache} from 'react'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID // "pv8y60vp"
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET // "production"
const apiVersion = process.env.NEXT_PUBLIC_SANITY_API_VERSION // "2023-05-03"
const client = createClient({
projectId,
dataset,
apiVersion, // https://www.sanity.io/docs/api-versioning
useCdn: true, // if you're using ISR or only static generation at build time then you can set this to `false` to guarantee no stale content
})
// Wrap the cache function in a way that reuses the TypeScript definitions
const clientFetch = cache(client.fetch.bind(client))
// Now use it just like before, fully deduped, cached and optimized by react
const data = await clientFetch(groq`*[]`)
// You can use the same generics as before
const total = await clientFetch<number>(groq`count*()`)
Until @sanity/client
can be updated to use fetch
in all environments, it's recommended that you configure cache rules and revalidation on the route segment level.
The new revalidateTags
API is not yet supported, but will be in a future release.
next-sanity
Visual Editing with Content Source MapsNote
Content Source Maps are available as an API for select Sanity enterprise customers. Contact our sales team for more information.
The createClient
method in next-sanity
supports visual editing, it supports all the same options as @sanity/preview-kit/client
. Add studioUrl
to your client configuration and it'll automatically show up on Vercel Preview Deployments:
import {createClient, groq} from 'next-sanity'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID // "pv8y60vp"
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET // "production"
const apiVersion = process.env.NEXT_PUBLIC_SANITY_API_VERSION // "2023-05-03"
const client = createClient({
projectId,
dataset,
apiVersion, // https://www.sanity.io/docs/api-versioning
useCdn: true, // if you're using ISR or only static generation at build time then you can set this to `false` to guarantee no stale content
studioUrl: '/studio', // Or: 'https://my-cool-project.sanity.studio'
encodeSourceMap: true, // Optional. Default to: process.env.NEXT_PUBLIC_VERCEL_ENV === 'preview',
})
Our setup guide walks you through how to customize the experience.
next-sanity/preview
Preview drafts, hot reload on changesChose a setup guide for the router you're using:
Since next-sanity/preview
is simply re-exporting LiveQueryProvider
and useLiveQuery
from @sanity/preview-kit
you'll find advanced usage and comprehensive docs in its README.
next-sanity/studio
The latest version of Sanity Studio allows you to embed a near-infinitely configurable content editing interface into any React application. This opens up many possibilities:
NextStudio
loads up the import {Studio} from 'sanity'
component for you and wraps it in a Next-friendly layout. metadata
specifies the necessary <meta>
tags for making the Studio adapt to mobile devices, and prevents the route from being indexed by search engines.
Both the Next /app
and /pages
examples uses this config file:
sanity.config.ts
:
import {defineConfig} from 'sanity'
import {deskTool} from 'sanity/desk'
import {schemaTypes} from './schemas'
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID!
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET!
export default defineConfig({
basePath: '/studio', // <-- important that `basePath` matches the route you're mounting your studio from, it applies to both `/pages` and `/app`
projectId,
dataset,
plugins: [deskTool()],
schema: {
types: schemaTypes,
},
})
To use sanity.cli.ts
with the same projectId
and dataset
as your sanity.config.ts
:
/* eslint-disable no-process-env */
import {loadEnvConfig} from '@next/env'
import {defineCliConfig} from 'sanity/cli'
const dev = process.env.NODE_ENV !== 'production'
loadEnvConfig(__dirname, dev, {info: () => null, error: console.error})
const projectId = process.env.NEXT_PUBLIC_SANITY_PROJECT_ID
const dataset = process.env.NEXT_PUBLIC_SANITY_DATASET
export default defineCliConfig({api: {projectId, dataset}})
Now you can run commands like npx sanity cors add
. See npx sanity help
for a full list of what you can do.
app-router
app/studio/[[...index]]/page.tsx
:
import {Studio} from './Studio'
// Set the right `viewport`, `robots` and `referer` meta tags
export {metadata} from 'next-sanity/studio/metadata'
export default function StudioPage() {
return <Studio />
}
app/studio/[[...index]]/Studio.tsx
:
'use client'
import {NextStudio} from 'next-sanity/studio'
import config from '../../../sanity.config'
export function Studio() {
// Supports the same props as `import {Studio} from 'sanity'`, `config` is required
return <NextStudio config={config} />
}
Customize meta tags
app/studio/[[...index]]/page.tsx
:
import type {Metadata} from 'next'
import {metadata as studioMetadata} from 'next-sanity/studio/metadata'
import {Studio} from './Studio'
// Set the right `viewport`, `robots` and `referer` meta tags
export const metadata: Metadata = {
...studioMetadata,
// Overrides the viewport to resize behavior
viewport: `${studioMetadata.viewport}, interactive-widget=resizes-content`,
}
export default function StudioPage() {
return <Studio />
}
pages-router
/pages/studio/[[...index]].tsx
:
import Head from 'next/head'
import {NextStudio} from 'next-sanity/studio'
import {metadata} from 'next-sanity/studio/metadata'
import config from '../../sanity.config'
export default function StudioPage() {
return (
<>
<Head>
{Object.entries(metadata).map(([key, value]) => (
<meta key={key} name={key} content={value} />
))}
</Head>
<NextStudio config={config} />
</>
)
}
StudioProvider
and StudioLayout
If you want to go lower level and have more control over the studio you can pass StudioProvider
and StudioLayout
from sanity
as children
:
import {NextStudio} from 'next-sanity/studio'
import {StudioProvider, StudioLayout} from 'sanity'
import config from '../../../sanity.config'
function StudioPage() {
return (
<NextStudio config={config}>
<StudioProvider config={config}>
{/* Put components here and you'll have access to the same React hooks as Studio gives you when writing plugins */}
<StudioLayout />
</StudioProvider>
</NextStudio>
)
}
next-sanity/webhook
Implements @sanity/webhook
to parse and verify that a Webhook is indeed coming from Sanity infrastructure.
pages/api/revalidate
:
import type {NextApiRequest, NextApiResponse} from 'next'
import {parseBody} from 'next-sanity/webhook'
// Export the config from next-sanity to enable validating the request body signature properly
export {config} from 'next-sanity/webhook'
export default async function revalidate(req: NextApiRequest, res: NextApiResponse) {
try {
const {isValidSignature, body} = await parseBody(req, process.env.SANITY_REVALIDATE_SECRET)
if (!isValidSignature) {
const message = 'Invalid signature'
console.warn(message)
res.status(401).json({message})
return
}
const staleRoute = `/${body.slug.current}`
await res.revalidate(staleRoute)
const message = `Updated route: ${staleRoute}`
console.log(message)
return res.status(200).json({message})
} catch (err) {
console.error(err)
return res.status(500).json({message: err.message})
}
}
v4
to v5
<0.4
to v4
Run "CI & Release" workflow. Make sure to select the main branch and check "Release new version".
Semantic release will only release on configured branches, so it is safe to run release on any branch.
MIT-licensed. See LICENSE.
FAQs
Sanity.io toolkit for Next.js
The npm package next-sanity receives a total of 68,031 weekly downloads. As such, next-sanity popularity was classified as popular.
We found that next-sanity demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 55 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We're introducing a new Analytics feature in the Socket dashboard so you can view changes in your organization's and repositories' alerts over time.
Security News
A new OpenSSF report uncovers critical gaps in secure software training, with 75% of new developers unfamiliar with secure practices, highlighting urgent educational needs.
Security News
The 2023 Python Developers Survey reveals key trends in packaging, web frameworks, and developer demographics, highlighting a shift toward innovative tools as the Python community diversifies and grows among less experienced developers.