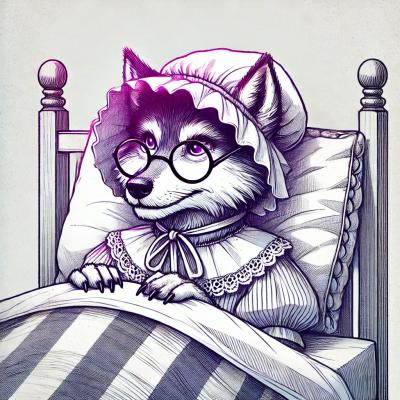
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
next-typed-routes
Advanced tools
// /routes.ts
import { createRoute } from "next-typed-routes";
export const routes = {
// For `/pages/index.tsx`
top: createRoute("/"),
// For `/pages/users/index.tsx`
users: createRoute("/users"),
// For `/pages/users/[userId].tsx`
usersDetail: (userId: number) => createRoute("/users/[userId]", { userId }),
};
// /pages/index.tsx
import Link from "next/link";
import { routes } from "../routes.ts";
const targetUserId = 5;
const Page () => (
<Link {...routes.usersDetail(targetUserId)}>
<a>Go to the user page (id: {targetUserId})</a>
</Link>
);
...
Page.getInitialProps = async ({ res }) => {
// Redirect to `/users/123&limit=30` .
// This works fine on client-side and server-side.
movePage(routes.usersDetail(123), { res, queryParameters: { limit: 30 } })
};
FEATURES | WHAT YOU CAN DO |
---|---|
❤️ Designed for Next.js | You can use Next.js routing system without custom server |
🌐 Build for Universal | Ready for Universal JavaScript |
📄 Write once, Manage one file | All you need is write routes to one file |
🎩 Type Safe | You can use with TypeScript |
Next.js 9 is the first version to support dynamic routing without any middleware. It is so useful and easy to use, and it supports
dynamic parameters such as /users/123
.
However the dynamic parameters do not support to be referred type safely. So when we rename a dynamic parameter, we should search
codes which use the parameter in <Link>
component and others and replace them with a new parameter name. Also it is thought
that developers may forget to specify required dynamic parameters when creating links.
next-typed-routes provides some APIs to resolve this issues. You can manage all routes with dynamic parameters in one file and you can create links type safely.
$ npm install next-typed-routes
If you are using Yarn, use the following command.
$ yarn add --dev next-typed-routes
import { createRoute } from "next-typed-routes";
export const routes = {
// For `/pages/index.tsx`
top: createRoute("/"),
// For `/pages/users/index.tsx`
users: createRoute("/users"),
// For `/pages/users/[userId].tsx`
usersDetail: (userId: number) => createRoute("/users/[userId]", { userId }),
};
Firstly, you need to define routes using next-typed-routes.
createRoute
function exported from next-typed-routes returns an object for <Link>
component props, which has href
and as
properties.
So when you manage values created by createRoute
, you can get <Link>
component props via the keys like the following.
<Link {...routes.top}>
<a>Go to top.</a>
</Link>
// Or
<Link href={routes.top.href} as={routes.top.as}>
<a>Go to top.</a>
</Link>
createRoute
Currently, createRoute
accepts three parameters.
<Link {...createRoute("/")} />
<Link {...createRoute("/users", undefined, { limit: 10 })} />
<Link {...createRoute("/users/[userId]/items/[itemId]", { userId: 1, itemId: 2 })} />
path: string
/pages
in general.parameters: { [key: string]: number | string | undefined | null }
{}
.queryParameters: { [key: string]: number | string | boolean | null | undefined }
{}
.import { createRoute } from "next-typed-routes";
export const routes = {
// For `/pages/index.tsx`
"/": createRoute("/"),
// For `/pages/users/index.tsx`
"/users": createRoute("/users"),
// For `/pages/users/[userId].tsx`
"/users/[userId]": (userId: number) => createRoute("/users/[userId]", { userId }),
};
You can freely name keys of routes
, but I recommend you to adopt the same with page file path for key names in order to reduce
the thinking time to name them.
import Router from "next/router";
import { createPageMover } from "next-typed-routes";
const movePage = createPageMover("https://you-project.example.com", Router);
movePage("/about");
movePage(createRoute("/"));
next-typed-routes provides a function to reidrect to a specific page using createRoute
. This works fine on cliet-side (web browsers)
and server-side.
If you want to support server-side, you must give res
object from getInitialProps
arguments.
Component.getInitialProps = async ({ res }) => {
movePage("/about", { res });
};
createRoute(path, parameters, queryParameters): { href: string, as: string }
createRoute("/")
createRoute("/users", undefined, { limit: 10 })
createRoute("/users/[userId]/items/[itemId]", { userId: 1, itemId: 2 })
Returns an object for <Link>
component props.
path: string
/pages
in generalparameters: { [key: string]: number | string | undefined | null }
{}
queryParameters: { [key: string]: number | string | boolean | undefined | null }
{}
createPageMover(baseURI, Router): PageMover
createPageMover("https://you-project.example.com", Router);
createPageMover(new URL("https://you-project.example.com"), Router);
Returns a function to redirect URL.
baseURI: string | URL
Router: NextRouter
next/router
in your projectmovePage(path, options): void
movePage("/about");
movePage(createRoute("/about"));
movePage("/about", { res, statusCode: 301 });
movePage("/about", { res, queryParameters: { limit: 30 } });
This function is created from createPageMover
.
path: string | { href: string, as: string }
createRoute
options: Options
{}
res: ServerResponse
getInitialProps
argumentsqueryParameters: { [key: string]: number | string | boolean | undefined | null }
path
already has query string, it will be merged with queryParameters
statusCode: 301 | 302
Bug reports and pull requests are welcome on GitHub at https://github.com/jagaapple/next-typed-routes. This project is intended to be a safe, welcoming space for collaboration, and contributors are expected to adhere to the Contributor Covenant code of conduct.
Please read Contributing Guidelines before development and contributing.
The library is available as open source under the terms of the MIT License.
Copyright 2019 Jaga Apple. All rights reserved.
1.0.2 (2019-12-07)
FAQs
Type safe route utilities for Next.js.
The npm package next-typed-routes receives a total of 147 weekly downloads. As such, next-typed-routes popularity was classified as not popular.
We found that next-typed-routes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.