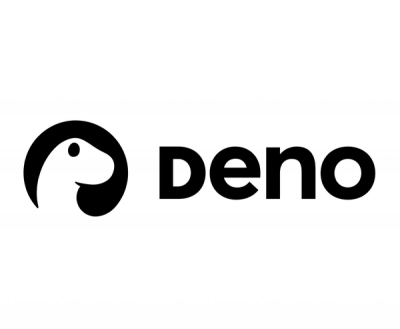
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This module will add the google api to your project. It wraps the Gapi in to a service layer allowing to work with Gapi in a Angular 9+ project.
made by codeforges
We have started to work on our video tutorials series, Angular + NestJs a full fledged application.
npm install ng-gapi
To use the ng-gapi
simply add GoogleApiModule
to your module imports
and set the configuration.
Bellow are all available parameters that can be provided in the forRoot()
method.
export interface NgGapiClientConfig extends ClientConfig {
discoveryDocs: string[];
}
//And the extended ClientConfig
interface ClientConfig {
/**
* The app's client ID, found and created in the Google Developers Console.
*/
client_id?: string;
/**
* The domains for which to create sign-in cookies. Either a URI, single_host_origin, or none.
* Defaults to single_host_origin if unspecified.
*/
cookie_policy?: string;
/**
* The scopes to request, as a space-delimited string. Optional if fetch_basic_profile is not set to false.
*/
scope?: string;
/**
* Fetch users' basic profile information when they sign in. Adds 'profile' and 'email' to the requested scopes. True if unspecified.
*/
fetch_basic_profile?: boolean;
/**
* The Google Apps domain to which users must belong to sign in. This is susceptible to modification by clients,
* so be sure to verify the hosted domain property of the returned user. Use GoogleUser.getHostedDomain() on the client,
* and the hd claim in the ID Token on the server to verify the domain is what you expected.
*/
hosted_domain?: string;
/**
* Used only for OpenID 2.0 client migration. Set to the value of the realm that you are currently using for OpenID 2.0,
* as described in <a href="https://developers.google.com/accounts/docs/OpenID#openid-connect">OpenID 2.0 (Migration)</a>.
*/
openid_realm?: string;
/**
* The UX mode to use for the sign-in flow.
* By default, it will open the consent flow in a popup.
*/
ux_mode?: "popup" | "redirect";
/**
* If using ux_mode='redirect', this parameter allows you to override the default redirect_uri that will be used at the end of the consent flow.
* The default redirect_uri is the current URL stripped of query parameters and hash fragment.
*/
redirect_uri?: string;
}
import {
GoogleApiModule,
GoogleApiService,
GoogleAuthService,
NgGapiClientConfig,
NG_GAPI_CONFIG,
GoogleApiConfig
} from "ng-gapi";
let gapiClientConfig: NgGapiClientConfig = {
client_id: "CLIENT_ID",
discoveryDocs: ["https://analyticsreporting.googleapis.com/$discovery/rest?version=v4"],
scope: [
"https://www.googleapis.com/auth/analytics.readonly",
"https://www.googleapis.com/auth/analytics"
].join(" ")
};
@NgModule({
imports: [
//...
GoogleApiModule.forRoot({
provide: NG_GAPI_CONFIG,
useValue: gapiClientConfig
}),
//...
]
})
export MyModule {}
Now you will have Access to the GoogleApi service.
The service has a a event method onLoad(callback)
This event will fire when the gapi script is loaded.
Usage example :
export class FooService {
constructor(gapiService: GoogleApiService) {
gapiService.onLoad().subscribe(()=> {
// Here we can use gapi
});
}
}
Also check the example folder with a google api reports module
The module has a GoogleAuth service which allows you to work with the google auth
Usage:
//Example of a UserService
@Injectable()
export class UserService {
public static SESSION_STORAGE_KEY: string = 'accessToken';
private user: GoogleUser;
constructor(private googleAuth: GoogleAuthService){
}
public getToken(): string {
let token: string = sessionStorage.getItem(UserService.SESSION_STORAGE_KEY);
if (!token) {
throw new Error("no token set , authentication required");
}
return sessionStorage.getItem(UserService.SESSION_STORAGE_KEY);
}
public signIn(): void {
this.googleAuth.getAuth()
.subscribe((auth) => {
auth.signIn().then(res => this.signInSuccessHandler(res));
});
}
private signInSuccessHandler(res: GoogleUser) {
this.user = res;
sessionStorage.setItem(
UserService.SESSION_STORAGE_KEY, res.getAuthResponse().access_token
);
}
}
Lets go step by step through the example
SESSION_STORAGE_KEY
is just a sugar to store string in a property rather then hardcodeFrom gapi docs https://developers.google.com/api-client-library/javascript/features/batch
we should use gapi.client.newBatch()
But in our case we have typings and OOP, so we can do this:
export class FooService {
constructor(gapiService: GoogleApiService) {
gapiService.onLoad().subscribe(()=> {
const myBatch: HttpBatch = new HttpBatch();
myBatch.add(
// your request
);
});
}
}
The GoogleApiConfig class provides the required configuration for the Api
Configuration is easy to use. The GoogleApiModule has a static method which sets the configs.
As shown in the example you simply provide a configuration object of type ClientConfig
.
{
client_id: "your client id",
discoveryDocs: ["url to discovery docs", "another url"],
scope: "space separated scopes"
}
Configure them according your google app configurations and resource scope.
We are providing Web Development and Consulting Services. codeforges.com
FAQs
Angular 9+ Google api module ng-gapi
The npm package ng-gapi receives a total of 1,369 weekly downloads. As such, ng-gapi popularity was classified as popular.
We found that ng-gapi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.