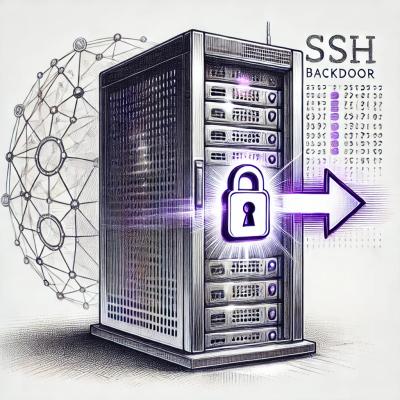
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Angular 2 Drag-and-Drop without dependencies.
npm install ng2-dnd --save`
To be aavailable soon...
If you use SystemJS to load your files, you might have to update your config with this if you don't use defaultJSExtensions: true
:
System.config({
packages: {
"/ng2-dnd": {"defaultExtension": "js"}
}
});
Finally, you can use ng2-dnd in your Angular 2 project:
DND_PROVIDERS, DND_DIRECTIVES
from ng2-toasty/ng2-toasty
;DND_PROVIDERS
in the bootstrap of your application;DND_DIRECTIVES
to the "directives" property of your application component;dnd-draggable
and 'dnd-droppable' properties in template of your components.import {Component} from 'angular2/core';
import {DND_PROVIDERS, DND_DIRECTIVES} from 'ng2-dnd/ng2-dnd';
import {bootstrap} from 'angular2/platform/browser';
bootstrap(AppComponent, [
DND_PROVIDERS // It is required to have 1 unique instance of your service
]);
@Component({
selector: 'app',
directives: [DND_DIRECTIVES],
template: `
<h4>Simple DnD</h4>
<div class="row">
<div class="col-sm-3">
<div class="panel panel-success">
<div class="panel-heading">Available to drag</div>
<div class="panel-body">
<div class="panel panel-default" dnd-draggable [dragEnabled]="true">
<div class="panel-body">
<div>Drag Me</div>
</div>
</div>
</div>
</div>
</div>
<div class="col-sm-3">
<div dnd-droppable class="panel panel-info">
<div class="panel-heading">Place to drop</div>
<div class="panel-body">
</div>
</div>
</div>
<div class="col-sm-3">
<div dnd-droppable class="panel panel-warning">
<div class="panel-heading">Restricted to drop</div>
<div class="panel-body">
</div>
</div>
</div>
</div>
`
})
export class AppComponent {
constructor() { }
}
Result of simple Drag&Drop operation:
You can use property dropZones (actually an array) to specify in which place you would like to drop the draggable element:
import {Component} from 'angular2/core';
import {DND_PROVIDERS, DND_DIRECTIVES} from 'ng2-dnd/ng2-dnd';
import {bootstrap} from 'angular2/platform/browser';
bootstrap(AppComponent, [
DND_PROVIDERS // It is required to have 1 unique instance of your service
]);
@Component({
selector: 'app',
directives: [DND_DIRECTIVES],
template: `
<h4>Restricted DnD with zones</h4>
<div class="row">
<div class="col-sm-3">
<div class="panel panel-primary">
<div class="panel-heading">Available to drag</div>
<div class="panel-body">
<div class="panel panel-default" dnd-draggable [dragEnabled]="true" [dropZones]="['zone1']">
<div class="panel-body">
<div>Drag Me</div>
<div>Zone 1 only</div>
</div>
</div>
</div>
</div>
<div class="panel panel-success">
<div class="panel-heading">Available to drag</div>
<div class="panel-body">
<div class="panel panel-default" dnd-draggable [dragEnabled]="true" [dropZones]="['zone1', 'zone2']">
<div class="panel-body">
<div>Drag Me</div>
<div>Zone 1 & 2</div>
</div>
</div>
</div>
</div>
</div>
<div class="col-sm-3">
<div dnd-droppable class="panel panel-info" [dropZones]="['zone1']">
<div class="panel-heading">Zone 1</div>
<div class="panel-body">
</div>
</div>
</div>
<div class="col-sm-3">
<div dnd-droppable class="panel panel-warning" [dropZones]="['zone2']">
<div class="panel-heading">Zone 2</div>
<div class="panel-body">
</div>
</div>
</div>
</div>
`
})
export class AppComponent {
constructor() { }
}
Result of restricted Drag&Drop operation:
You can transfer data from draggable to droppable component via dragData property of Draggable component:
import {Component} from 'angular2/core';
import {DND_PROVIDERS, DND_DIRECTIVES} from 'ng2-dnd/ng2-dnd';
import {bootstrap} from 'angular2/platform/browser';
bootstrap(AppComponent, [
DND_PROVIDERS // It is required to have 1 unique instance of your service
]);
@Component({
selector: 'app',
directives: [DND_DIRECTIVES],
template: `
<h4>Transfer custom data in DnD</h4>
<div class="row">
<div class="col-sm-3">
<div class="panel panel-success">
<div class="panel-heading">Available to drag</div>
<div class="panel-body">
<div class="panel panel-default" dnd-draggable [dragEnabled]="true" [dragData]="transferData">
<div class="panel-body">
<div>Drag Me</div>
<div>{{transferData | json}}</div>
</div>
</div>
</div>
</div>
</div>
<div class="col-sm-3">
<div dnd-droppable class="panel panel-info" (onDropSuccess)="transferDataSuccess($event)">
<div class="panel-heading">Place to drop (Items:{{receivedData.length}})</div>
<div class="panel-body">
<div [hidden]="!receivedData.length > 0" *ngFor="#data of receivedData">{{data | json}}</div>
</div>
</div>
</div>
</div>
`
})
export class AppComponent {
transferData:Object = {id:1, msg: 'Hello'};
receivedData:Array<any> = [];
constructor() { }
transferDataSuccess($event) {
this.receivedData.push($event);
}
}
Result of transfer cudtom data in Drag&Drop operation:
Here is an example of shopping backet with products adding via drag and drop operation:
import {Component} from 'angular2/core';
import {DND_PROVIDERS, DND_DIRECTIVES} from 'ng2-dnd/ng2-dnd';
import {bootstrap} from 'angular2/platform/browser';
bootstrap(AppComponent, [
DND_PROVIDERS // It is required to have 1 unique instance of your service
]);
@Component({
selector: 'app',
directives: [DND_DIRECTIVES],
template: `
<h4>Drag And Drop - Shopping basket</h4>
<div class="row">
<div class="col-sm-3">
<div class="panel panel-success">
<div class="panel-heading">Available products</div>
<div class="panel-body">
<div *ngFor="#product of availableProducts" class="panel panel-default"
dnd-draggable [dragEnabled]="product.quantity>0" [dragData]="product" (onDragSuccess)="orderedProduct($event)" [dropZones]="['demo1']">
<div class="panel-body">
<div [hidden]="product.quantity===0">{{product.name}} - \${{product.cost}}<br>(available: {{product.quantity}})</div>
<div [hidden]="product.quantity>0"><del>{{product.name}}</del><br>(NOT available)</div>
</div>
</div>
</div>
</div>
</div>
<div class="col-sm-3">
<div dnd-droppable (onDropSuccess)="addToBasket($event)" [dropZones]="['demo1']" class="panel panel-info">
<div class="panel-heading">Shopping Basket<br>(to pay: \${{totalCost()}})</div>
<div class="panel-body">
<div *ngFor="#product of shoppingBasket" class="panel panel-default">
<div class="panel-body">
{{product.name}}<br>(ordered: {{product.quantity}}<br>cost: \${{product.cost * product.quantity}})
</div>
</div>
</div>
</div>
</div>
</div>
`
})
export class AppComponent {
availableProducts: Array<Product> = [];
shoppingBasket: Array<Product> = [];
constructor() {
this.availableProducts.push(new Product("Blue Shoes", 3, 35));
this.availableProducts.push(new Product("Good Jacket", 1, 90));
this.availableProducts.push(new Product("Red Shirt", 5, 12));
this.availableProducts.push(new Product("Blue Jeans", 4, 60));
}
orderedProduct(orderedProduct: Product) {
orderedProduct.quantity--;
}
addToBasket(newProduct: Product) {
for (let indx in this.shoppingBasket) {
let product:Product = this.shoppingBasket[indx];
if (product.name === newProduct.name) {
product.quantity++;
return;
}
}
this.shoppingBasket.push(new Product(newProduct.name, 1, newProduct.cost));
}
totalCost():number {
let cost:number = 0;
for (let indx in this.shoppingBasket) {
let product:Product = this.shoppingBasket[indx];
cost += (product.cost * product.quantity);
}
return cost;
}
}
FAQs
Angular 2 Drag-and-Drop without dependencies
We found that ng2-dnd demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.