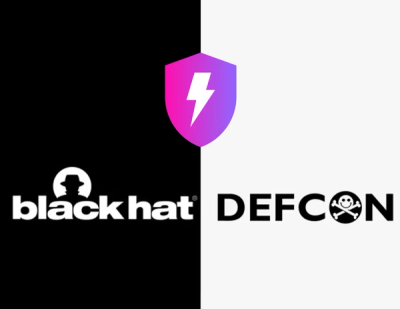
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
ngx-component-outlet
Advanced tools
NgComponentOutlet + Data-Binding + Full Lifecycle = NgxComponentOutlet for Angular 5, 6+
Best way to quickly use Dynamic Components with Angular
Use like NgComponentOutlet
but with @Input/@Output
auto bindings:
<!-- host component -->
<app-dynamic
<!-- dynamic component -->
[ngxComponentOutlet]="component"
<!-- regular input -->
[entity]="entity"
<!-- regular output -->
(action)="onAction($event)">
</app-dynamic>
Feature | NgxComponentOutlet | ComponentFactoryResolver | NgComponentOutlet |
---|---|---|---|
Friendliness | ⭐⭐⭐ | ⭐ | ⭐⭐ |
Dynamic Components | ✅ | ✅ | ✅ |
AOT support | ✅ | ✅ | ✅ |
Reactivity | ✅ | ✅ | ✅ |
Injector | ✅ | ✅ | ✅ |
NgModule | ✅ | ✅ | ✅ |
projectionNodes | ✅ | ✅ | ✅ |
Component Access | ✅ | ✅ | ❌ |
Lifecycle OnChanges | ✅ | ⭕️ manually | ❌ |
Binding @Input() | ✅ | ⭕️ manually | ❌ |
Binding @Output() | ✅ | ⭕️ manually | ❌ |
Activate Event | ✅ | ⭕️ manually | ❌ |
Deactivate Event | ✅ | ⭕️ manually | ❌ |
List of heroes
Table of heroes with table schema form
ngx-component-outlet
:npm install --save ngx-component-outlet
yarn add ngx-component-outlet
import { NgxComponentOutletModule } from 'ngx-component-outlet';
@NgModule({
declarations: [ AppComponent ],
imports: [ NgxComponentOutletModule.forRoot() ],
bootstrap: [ AppComponent ]
})
export class AppModule {}
@Component({
selector: 'app-dynamic-comp-a',
template: `I'm Dynamic Component A. Hello, {{ name }}!`
})
export class CompAComponent {
@Input() name: string;
}
@Component({
selector: 'app-dynamic-comp-b',
template: `I'm Dynamic Component B. Hello, {{ name }}!`
})
export class CompBComponent {
@Input() name: string;
}
declarations
and entryComponents
:@NgModule({
...
declarations: [ ..., CompAComponent, CompBComponent ],
entryComponents: [ CompAComponent, CompBComponent ]
})
export class AppModule {}
@Component({
selector: 'app-host-for-dynamic',
template: ''
})
export class HostComponent {
@Input() name: string;
}
declarations
:@NgModule({
...
declarations: [ ..., HostComponent ],
...
})
export class AppModule {}
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<app-host-for-dynamic [ngxComponentOutlet]="componentA"
[name]="'Angular 5!'"></app-host-for-dynamic>
<app-host-for-dynamic [ngxComponentOutlet]="componentB"
[name]="'Angular 6?'"></app-host-for-dynamic>
`
})
export class AppComponent {
componentA = CompAComponent;
componentB = CompBComponent;
}
import { NgxComponentOutletModule } from 'ngx-component-outlet';
@NgModule({
imports: [ NgxComponentOutletModule.forRoot() ],
declarations: [ AppComponent, CompAComponent, CompBComponent, HostComponent ],
entryComponents: [ CompAComponent, CompBComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule {}
Input | Type | Default | Required | Description |
---|---|---|---|---|
[ngxComponentOutlet] | Type<any> | n/a | yes | |
[ngxComponentOutletInjector] | Injector | n/a | no | |
[ngxComponentOutletContent] | any[][] | n/a | no | |
[ngxComponentOutletNgModuleFactory] | NgModuleFactory<any> | n/a | no |
Output | Type | Description |
---|---|---|
(ngxComponentOutletActivate) | any | |
(ngxComponentOutletDeactivate) | any |
Here is a demo repository showing ngx-component-outlet and Angular in action.
FAQs
NgComponentOutlet + Data-Binding + Full Lifecycle = NgxComponentOutlet for Angular 5, 6+
The npm package ngx-component-outlet receives a total of 12 weekly downloads. As such, ngx-component-outlet popularity was classified as not popular.
We found that ngx-component-outlet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.