ngx-marquee
Powerful Marquee Component for Angular (based on observers behavior). Improved alternative to the deprecated HTML marquee tag.

This library was generated with Angular CLI.
How to install
Run npm i ngx-marquee
to install the library.
How to use
Import Module
import { NgxMarqueeModule } from 'ngx-marquee';
@NgModule({
imports: [ NgxMarqueeModule ],
...
})
export class AppModule { }
Use Component
<ngx-marquee>
<mark class="ticker-custom">My ticker content</mark>
</ngx-marquee>
Demo
Resume properties
Name | Type | Description |
---|
[direction] | string | Sets the controlling direction of marquee movement. |
[duration] | string | Sets the length of time that an animation takes to complete one cycle. |
[pauseOnHover] | boolean | Set to pause the marquee movement while hover user event. |
[animation] | string | Sets animation entrance when the marquee cycle starts. |
[pendingUpdates] | boolean | Indicates that taskOnUpdateContent callback function will be executed when the current movement cycle has been finished. |
[taskOnUpdateDuration] | function | Callback function to be used to determine the new duration value that an animation takes to complete the next cycle. This callback function will be executed if pendingUpdates property is set on true and the current cycle of marquee movement has been finished. |
[taskOnUpdateContent] | function | Callback function to be used to set new content in the next cycle. This callback function will be executed while pendingUpdates property is set on true and the current cycle of marquee movement has been finished. |
(pendingUpdatesChange) | boolean | This event indicates that the taskOnUpdateDuration or taskOnUpdateContent callbacks functions have been executed. |
(playStateChange) | MarqueeState | This event indicates the current state movement of the marquee. |
Resume methods
Name | Description |
---|
playPause | Toggle the movement of the marquee to play or pause. |
stop | Stop the movement of the marquee. |
restart | Restart the movement of the marquee to the initial move point. |
API
input properties
@Input() direction
string
Property sets the controlling direction of marquee movement.
default value: "left"
Note: The taskOnUpdateContent()
callback function is not fired when direction
value is "alternate".
possible values
Example
<ngx-marquee direction="right">
<mark>My ticker text</mark>
</ngx-marquee>
@Input() duration
string
Property sets the length of time that an animation takes to complete one cycle.
default value: "20s"
Note: The duration string format must be a value preceded by a letter s or ms to denote time in seconds or milliseconds respectively.
Example
<ngx-marquee duration="120s">
<mark>My ticker text</mark>
</ngx-marquee>
@Input() pauseOnHover
boolean
Property set to pause the marquee movement while hover user event.
default value: false
Example
<ngx-marquee pauseOnHover="true">
<mark>My ticker text</mark>
</ngx-marquee>
@Input() animation
string
Property sets animation entrance when the marquee cycle starts.
default value: "default"
Note: Only "default" animation is available when direction
value is "alternate".
possible values
- "default"
- "slideInUp"
- "slideInDown"
Example
<ngx-marquee animation="slideInUp">
<mark>My ticker text</mark>
</ngx-marquee>
@Input() pendingUpdates
boolean
Property indicates that taskOnUpdateContent
callback function will be executed when the current movement cycle has been finished.
default value: false
Example
<ngx-marquee pendingUpdates="false">
<mark>My ticker text</mark>
</ngx-marquee>
@Input() taskOnUpdateDuration
function
Customized callback function to be used to determine the new duration
value that an animation takes to complete the next cycle. This callback function will be executed if pendingUpdates
property is set on true and the current cycle of marquee movement has been finished.
return: string
Note: If taskOnUpdateDuration( ) function is not supplied, the duration
value is considered.
possible values
foo = (): string => {
return "90ms";
};
Example
<ngx-marquee [taskOnUpdateDuration]="foo" pendingUpdates="true">
<mark>My ticker text</mark>
</ngx-marquee>
@Input() taskOnUpdateContent
function
Customized callback function to be used to set new content in the next cycle. This callback function will be executed while pendingUpdates
property is set on true and the current cycle of marquee movement has been finished.
return: void
Note: If taskOnUpdateContent( ) function is not supplied, the marquee remains unchanged.
possible values
anotherFoo = (): void => {
};
Example
<ngx-marquee [taskOnUpdateContent]="anotherFoo" pendingUpdates="true">
<mark>My ticker text</mark>
</ngx-marquee>
output properties
@Output() pendingUpdatesChange
This event indicates that the taskOnUpdateDuration
or taskOnUpdateContent
callbacks functions have been executed.
return: EventEmitter<boolean>
Note: You can take advantage of two-way data binding pattern for update pendingUpdates
value simultaneously between your component logic and the marquee.
Example
<ngx-marquee [(pendingUpdates)]="isPendingUpdate" [taskOnUpdateContent]="anotherFoo">
<mark>My ticker text</mark>
</ngx-marquee>
@Output() playStateChange
This event indicates the current state movement of the marquee.
return: EventEmitter<MarqueeState>
possible values
Note: You can map these values to respective lowercase "running", "paused", or "stopped" string's value.
Example
<ngx-marquee (playStateChange)="listenerFoo($event)">
<mark>My ticker text</mark>
</ngx-marquee>
methods
playPause( ): void
Toggle the movement of the marquee to play or pause.
Note: Sets and emit (by playStateChange
event) the current MarqueeState
to Running or Paused.
stop( ): void
Stop the movement of the marquee.
Note: Sets and emit (by playStateChange
event) the current MarqueeState
to Stopped. Also, set the marquee to the initial move point and set the value of pendingUpdates
to false.
restart( ): void
Restart the movement of the marquee to the initial move point.
Note: Sets and emit (by playStateChange
event) the current MarqueeState
to Running.
Any contributions are appreciated
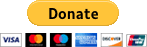
Inspired by https://github.com/shivarajnaidu/ng-marquee