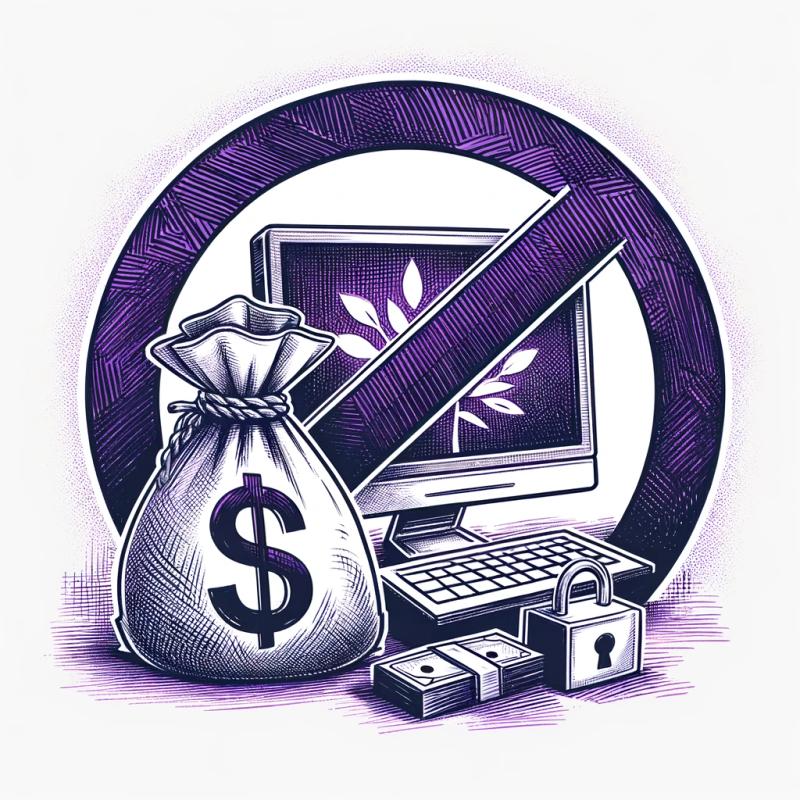
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Package description
The nise npm package is a library for creating fake servers, responses, and timers in JavaScript tests. It allows developers to simulate server responses and time-based behavior without the need for an actual server or waiting for real time to pass. This is particularly useful in unit testing, where tests need to be both fast and deterministic.
Fake XMLHttpRequest and server
This feature allows you to create a fake server that can respond to XMLHttpRequests. You can specify the HTTP method, URL, and response details. This is useful for testing AJAX requests without needing to hit a real server.
var fakeServer = nise.fakeServer.create();
fakeServer.respondWith('GET', '/some/article', [200, { 'Content-Type': 'application/json' }, '{ "id": 12, "comment": "Hey there" }']);
fakeServer.respondImmediately = true;
Fake timers
With fake timers, you can simulate the passage of time in tests. This is useful for functions that rely on setTimeout, setInterval, or Date objects. It allows you to test time-dependent code without real time delays.
var clock = nise.useFakeTimers();
clock.tick(1000); // Simulate the passage of 1 second
Sinon is a popular testing library that includes functionalities similar to nise, such as spies, stubs, mocks, and fake servers. While nise focuses on network requests and timers, Sinon provides a broader range of testing utilities, making it a more comprehensive solution for many testing scenarios.
Nock is a powerful HTTP server mocking and expectations library for Node.js. Unlike nise, which provides both fake servers and timers, nock focuses exclusively on intercepting and mocking HTTP requests. It allows for a more detailed and flexible setup of request interception, making it a strong choice for testing HTTP interactions.
Readme
fake XHR and Server
Documentation: http://sinonjs.github.io/nise/
Support us with a monthly donation and help us continue our activities. [Become a backer]
Become a sponsor and get your logo on our README on GitHub with a link to your site. [Become a sponsor]
nise was released under BSD-3
FAQs
Fake XHR and server
We found that nise demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).