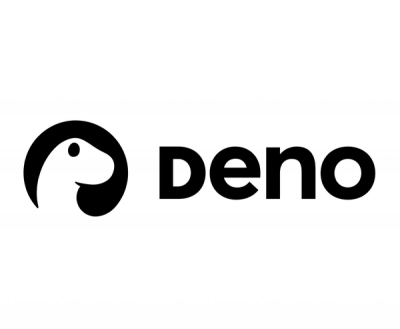
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Simple commandline/terminal/shell interface to allow you to run cli or bash style commands as if you were in the terminal.
Node.js commandline/terminal interface.
Simple commandline, terminal, or shell interface to allow you to run cli or bash style commands as if you were in the terminal.
Run commands asynchronously, and if needed can get the output as a string.
npm info :
See npm trends and stats for node-cmd
GitHub info :
Package details websites :
This work is licenced via the MIT Licence.
method | arguments | functionality | returns |
---|---|---|---|
run | command, callback | runs a command asynchronously | args for callback err ,data ,stderr |
runSync | command | runs a command synchronously | obj {err ,data ,stderr } |
//*nix
var cmd=require('node-cmd');
//*nix supports multiline commands
cmd.runSync('touch ./example/example.created.file');
cmd.run(
`cd ./example
ls`,
function(err, data, stderr){
console.log('examples dir now contains the example file along with : ',data)
}
);
//Windows
var cmd=require('node-cmd');
//Windows multiline commands are not guaranteed to work try condensing to a single line.
const syncDir=cmd.runSync('cd ./example & dir');
console.log(`
Sync Err ${syncDir.err}
Sync stderr: ${syncDir.stderr}
Sync Data ${syncDir.data}
`);
cmd.run(`dir`,
function(err, data, stderr){
console.log('the node-cmd dir contains : ',data)
}
);
//clone this repo!
var cmd=require('node-cmd');
const syncClone=cmd.runSync('git clone https://github.com/RIAEvangelist/node-cmd.git');
console.log(syncClone);
var cmd=require('node-cmd');
var process=cmd.run('node');
console.log(process.pid);
const cmd=require('node-cmd');
const processRef=cmd.run('python -i');
let data_line = '';
//listen to the python terminal output
processRef.stdout.on(
'data',
function(data) {
data_line += data;
if (data_line[data_line.length-1] == '\n') {
console.log(data_line);
}
}
);
const pythonTerminalInput=`primes = [2, 3, 5, 7]
for prime in primes:
print(prime)
`;
//show what we are doing
console.log(`>>>${pythonTerminalInput}`);
//send it to the open python terminal
processRef.stdin.write(pythonTerminalInput);
Output :
>>>primes = [2, 3, 5, 7]
for prime in primes:
print(prime)
2
3
5
7
FAQs
Simple commandline/terminal/shell interface to allow you to run cli or bash style commands as if you were in the terminal.
The npm package node-cmd receives a total of 20,227 weekly downloads. As such, node-cmd popularity was classified as popular.
We found that node-cmd demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.